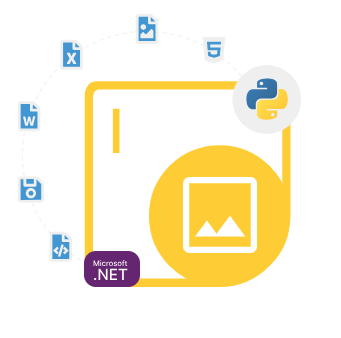
Aspose.Imaging for Python via .NET
Create, Edit & Convert Images via Python
Python Imaging API that enables Programmers to Generate, Modify, Export, Process, Resize, Crop, Flip, Rotate & Compress different types of Images inside Python applications.
In the world of image processing and manipulation, developers often seek reliable and efficient tools to handle a wide range of tasks, from basic image conversions to advanced editing and enhancement. Aspose.Imaging for Python via .NET Library is one such powerful tool that has gained popularity in the developer community allowing them to work with images efficiently and effectively. It is a Python API built on top of the Aspose.Imaging for .NET library. It allows Python developers to work with a variety of image formats, perform image processing tasks, and seamlessly integrate them into their Python applications.
Aspose.Imaging for Python via .NET Library is a comprehensive API that enables software developers to perform a wide range of image processing tasks, from simple operations like resizing and cropping to complex tasks such as applying filters, converting between different image formats, and more. Its versatility makes it an invaluable tool for both beginners and seasoned developers, as it seamlessly integrates with Python and leverages the .NET framework to deliver high-performance image processing capabilities.
Aspose.Imaging for Python via .NET Library is a powerful and versatile library for Python developers who need to handle various types of images including JPEG, PNG, TIFF, BMP, TGA and ICO inside their applications. Whether you're building a web application, a desktop software, or a mobile app, this library simplifies image processing, conversion, and manipulation tasks. Its support for various image formats and advanced editing features make it a valuable addition to any developer's toolkit. If you're working on a project that involves image handling, consider giving Aspose.Imaging a try to see how it can streamline your image-related tasks.
Getting Started with Aspose.Imaging for Python via .NET
The recommend way to install Aspose.Imaging for Python via .NET is using PyPi. Please use the following command for a smooth installation.
Install Aspose.Imaging for Python via .NET via PyPi
pip install aspose-imaging-python-net
You can also download it directly from Aspose product page.Image Generation in Various Formats via Python
Aspose.Imaging for Python via .NET has provided complete support for creating new images from the scratch and manipulate existing images inside Python applications. The Library supports a wide range of image formats, including JPEG, PNG, BMP, TIFF, GIF, and many more. This means developers can easily work with images in different formats without worrying about compatibility issues. It supports various popular image file formats and allows reading as well as writing image file formats such as BMP, GIF, JPEG, PSD, TIFF, WEBP, PNG, WMF, EMF, SVG, TGA, and so on. Here is an example that shows how to create an image by setting path inside Python application.
How to Create an Image using Python Code?
import aspose.pycore as aspycore
from aspose.imaging import Image
from aspose.imaging.imageoptions import BmpOptions
from aspose.imaging.sources import FileCreateSource
import os
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
data_dir = templates_folder
# Creates an instance of BmpOptions and set its various properties
with BmpOptions() as image_options:
image_options.bits_per_pixel = 24
# Define the source property for the instance of BmpOptions Second boolean parameter determines if the file is temporal or not
image_options.source = FileCreateSource(os.path.join(data_dir, "result1.bmp"), False)
# Creates an instance of Image and call Create method by passing the BmpOptions object
with Image.create(image_options, 500, 500) as image:
image.save(os.path.join(data_dir, "result2.bmp"))
if delete_output:
os.remove(os.path.join(data_dir, "result1.bmp"))
os.remove(os.path.join(data_dir, "result2.bmp"))
Edit & Manipulate Image using Python API
Aspose.Imaging for Python via .NET makes it easy for software developers to load, edit and manipulate various types of images using Python API. The library provides a comprehensive set of tools for image editing, allowing you to perform tasks like resizing, cropping, rotating, and flipping images as well as and applying various filters and effects with ease. It also supports various color adjustments, including brightness, contrast, and saturation. The following example shows how to resize an image inside Python applications.
How to Resize an Image inside Python Applications?
from asposeimaging import Image
# Load the image
image = Image.load("input.jpg")
# Resize the image
new_width = 800
new_height = 600
image.resize(new_width, new_height)
# Save the resized image
image.save("output.jpg")
Image Metadata & Image Compression Support
Aspose.Imaging for Python via .NET enables computer programmers to access and modify image metadata, such as EXIF data and IPTC information. This is vital for tasks that require preserving or altering the metadata associated with images. Moreover, it allows users to compress images without compromising quality. This can be beneficial for optimizing image sizes, which is crucial for web applications. Thanks to its integration with the .NET framework, Aspose.Imaging delivers high-performance image processing, making it suitable for handling large and complex image manipulation tasks efficiently.
Image Conversion to Other Formats via Python
Aspose.Imaging for Python via .NET is a powerful tool that enables software developers to load and convert images with just a couple of lines of Python code. One of the standout features of Aspose.Imaging is its ability to convert images between different formats. Whether you need to transform a JPEG into a PNG, a TIFF into a BMP, or any other format, the library provides a straightforward way to achieve it. The following example shows how software developers can convert vector image to vectorized PSD image inside Python applications.
How to Convert Vector Image to Vectorized psd Image inside Python Apps?
import aspose.pycore as aspycore
from aspose.imaging import Image
from aspose.imaging.fileformats.psd import VectorDataCompositionMode
from aspose.imaging.imageoptions import PsdVectorizationOptions, PsdOptions, VectorRasterizationOptions
import os
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
# The path to the documents directory.
data_dir = templates_folder
input_file_name = os.path.join(data_dir, "template.cmx")
# properties is as simple as the following snippet:
with Image.load(input_file_name) as image:
obj_init = PsdVectorizationOptions()
obj_init.vector_data_composition_mode = VectorDataCompositionMode.SEPARATE_LAYERS
obj_init2 = PsdOptions()
obj_init2.vector_rasterization_options = VectorRasterizationOptions()
obj_init2.vectorization_options = obj_init
image_options = obj_init2
image_options.vector_rasterization_options.page_width = float(image.width)
image_options.vector_rasterization_options.page_height = float(image.height)
image.save(os.path.join(data_dir, "result.psd"), image_options)
if delete_output:
os.remove(os.path.join(data_dir, "result.psd"))