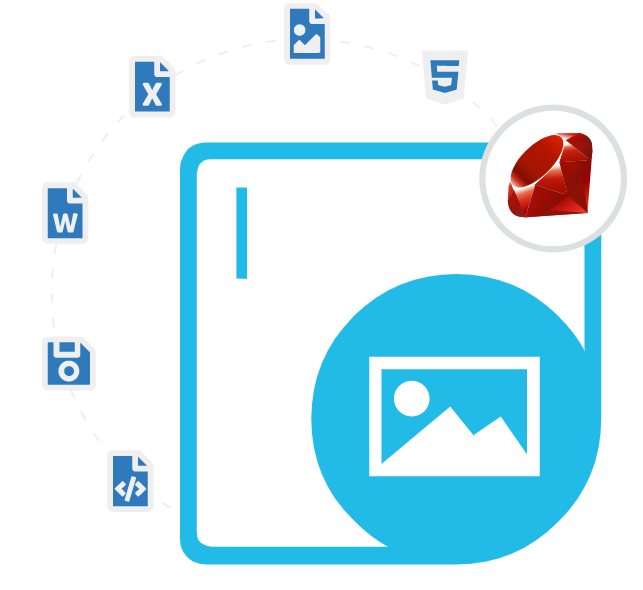
Aspose.Imaging Cloud SDK for Ruby
Ruby API to Create, Re-size, Rotate, & Convert Images
A Powerful cloud-based Image Processing API that allows Software Developers to Resize, Crop, Manipulate and Convert various Image Formats.
Aspose.Imaging Cloud SDK for Ruby is a very useful cloud-based image processing API that allows software developers to manipulate and convert numerous image file formats inside Ruby applications. The SDK provides a comprehensive set of image processing operations, including resizing, cropping, rotating, flipping, filtering, and many more. The SDK provides various optimization techniques that can be used to reduce the size of images without compromising on their quality.
Aspose.Imaging Cloud SDK for Ruby provides a wide range of features that allow software developers to process and convert images inside their applications, such as getting image properties, resizing images and saving to other formats, converting Images to another format, appending a TIFF image to another, deskew an Image, applying a filter to an image, getting frames range from the multipage image, reverse image search and many more. Moreover, software programmers can easily convert images from one format to another using the SDK, such as BMP, JPEG, PNG, GIF, TIFF, PSD, and more.
Aspose.Imaging Cloud SDK for Ruby has included support for a wide range of image formats, such as BMP, JPEG, PNG, GIF, JPEG2000, WEBP, PNG, WMF, EMF, SVG, TIFF, CMX, PSD, and more. It is a cloud-based API that can be accessed from anywhere in the world. Developers do not need to install any software on their local machines to use the API. If you're looking for an imaging API for your Ruby applications, Aspose.Imaging Cloud SDK for Ruby is definitely worth considering.
Getting Started with Aspose.Imaging Cloud SDK for Ruby
The recommend way to install Aspose.Imaging Cloud SDK for Ruby is using RubyGems. Please use the following command for a smooth installation.
Install Aspose.Imaging Cloud SDK for Ruby via RubyGems
gem build aspose-imaging-cloud.gemspec
You can also download it directly from Aspose product page.Image Resizing & Cropping via Ruby API
Aspose.Imaging Cloud SDK for Ruby has provided complete support for handling image resizing and other related operations inside Ruby applications. The API has included support for cropping images, specifying the position as well as the dimensions of the cropping rectangle, change format of the crop image, update width of the image, modify the height of the image, resize an existing image and save it in another format, and many more. The following example demonstrates how to resize an image using the SDK inside Ruby applications.
How to Resize an Image using the Ruby SDK?
require 'aspose_imaging_cloud'
# Initialize the API client
imaging_api = AsposeImagingCloud::ImagingApi.new
# Set the image file name
name = 'image.png'
# Set the new size
new_width = 200
new_height = 200
# Call the resize_image API method
response = imaging_api.resize_image(name, new_width, new_height)
# Save the resized image
File.write('resized_image.png', response.content)
Images Conversion to Other Formats via Ruby
Aspose.Imaging Cloud SDK for Ruby has included support for a wide range of image formats, such as BMP, JPEG, PNG, GIF, TIFF, PSD, and more. The SDK has included support for loading, viewing and converting some popular image file formats such as BMP, GIF, DJVU, WMF, EMF, JPEG, JPEG2000, PSD, TIFF, WEBP, PNG, DICOM, CDR, CMX, ODG, DNG and SVG. Software developers can also specify the input and output image names, formats, and other parameters such as the input and output folders. Programmers can easily upload an image to the cloud storage and convert it to desired image format in the cloud. Here's a sample code that shows how software developers can convert an image to another format using Ruby Cloud SDK.
How to Convert an Image to Another Format via Ruby API?
require 'aspose_imaging_cloud'
# Configure Aspose.Imaging Cloud API credentials
config = AsposeImagingCloud::Configuration.new
config.client_id = 'Your App SID'
config.client_secret = 'Your App Key'
# Create an instance of the API client
imaging_api = AsposeImagingCloud::ImagingApi.new(config)
# Set the input image name and format
name = 'input.jpg'
format = 'png'
# Set the output image name and format
output_name = 'output.png'
# Convert the input image to the output format
imaging_api.convert_image(AsposeImagingCloud::ConvertImageRequest.new(name, format, output_format: output_format, folder: 'input_folder', out_path: 'output_folder/' + output_name))
puts 'Image converted successfully.'
Apply Filter to an Image via Ruby API
Aspose.Imaging Cloud SDK for Ruby enables software developers to apply filter to an existing image inside Ruby applications. The library has included several important Filter types, such as BigRectangular, SmallRectangular, Median, GaussWiener, MotionWiener, GaussianBlur, Sharpen, BilateralSmoothing and more. In the following example the GaussWiener filter is applied to the image with a radius of 2.0 and smooth of 2.0. The output image format is PNG and the output image will be saved to the "output" folder in the cloud storage.
Apply Filter to An Image using Ruby SDK
filter_type = 'GaussWiener'
filter_properties = {
radius: 2.0,
smooth: 2.0
}
output_format = 'png'
response = imaging_api.apply_filter(
AsposeImagingCloud::ApplyFilterRequest.new(
name: source_image,
filter_type: filter_type,
filter_properties: filter_properties,
format: output_format,
folder: 'output'
)
)
// Download the output image:
output_image = 'output_image.png'
response = imaging_api.download_file(
AsposeImagingCloud::DownloadFileRequest.new(
path: "output/#{output_image}"
)
)
File.open(output_image, 'wb') do |file|
file.write(response)
end