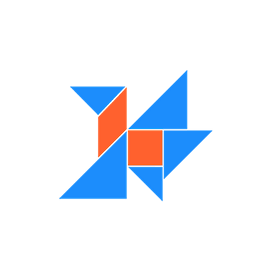
Kingfisher
Free Swift Library to Download & Cache Images
Open source Swift API that allows software developers to work with remote images inside their web apps. It allows to download and cache images, apply image processors and filters.
Kingfisher is a lightweight pure Swift implementation that provided complete functionality for working with multiple image file formats using Swift code. The library helps developers to create applications that can remotely handle images with ease. The library asynchronously downloads as well as caches images inside their own applications. The library supports a multiple-layer cache for the downloaded images that immensely enhances the performance of the apps. That means images will be cached in memory as well as on disk so no need to download it again.
The library is very easy to handle and provides a facility for cache management. Users can easily set the size as well as the duration of the cache. It will also provide an automatic cache cleaning facility that helps users to prevent the library from utilizing too many resources. One other great feature is included for task cancellation. Users can easily cancel the downloading or image retrieving process if it is not needed anymore.
The Kingfisher library facilitates developers to use image downloading and image caching components separately according to their needs. You can even create your own cache according to your own needs using Swift code. It Improves the disk cache performance by avoiding unnecessary disk operations. The library is open source and is available under the MIT License.
Getting Started with Kingfisher
Clone the latest sources with using the following command
Install Kingfisher via GitHub.
$ git submodule add https://github.com/onevcat/Kingfisher.git
Download & Cache Images via Swift Library
It is a very difficult task to programmatically download an image and store it to cache using a URL. The open source Swift library Kingfisher makes it easy for software developers to efficiently download and cache images inside their own applications. The library supports caching images both in memory as well as on disk. By default, the amount of RAM that will be used is not even limited and users can set the value themselves.
Download & Cache Images via Swift Library
let urls = ["https://example.com/image1.jpg", "https://example.com/image2.jpg"]
.map { URL(string: $0)! }
let prefetcher = ImagePrefetcher(urls: urls) {
skippedResources, failedResources, completedResources in
print("These resources are prefetched: \(completedResources)")
}
prefetcher.start()
// Later when you need to display these images:
imageView.kf.setImage(with: urls[0])
anotherImageView.kf.setImage(with: urls[1])
Image Viewing inside Swift Apps
The Kingfisher library allows software programmers to included image viewing capability inside their application with ease. The easiest way to for setting an image view is using UIImageView extension. The library will download the image from URL and lead it to both memory cache and disk cache, and display it in imageView. When latter users call the same URL, it will promptly retrieve and display the image from cache. It also support several functions related to images such as fading download image, showing placeholder, round corner image and so on.
View Images inside Swift Apps
import Kingfisher
let url = URL(string: "https://example.com/image.png")
imageView.kf.setImage(with: url)
Manually Store or Remove Cache Images
The open source Swift library Kingfisher makes enables programmers to store as well as remove images from cache with ease. By default, view extension methods can be used to store the retrieved image to cache automatically. But you can do it manually with cache.store() method. You can also pass the original data of the image which help the library to determine in which format the image should be stored. It also provides support for manually removing a certain image from cache. It also support clearing all cache data, report disk storage size and creating you own cache.
View Images inside Swift Apps
//Check whether an image in the cache
let cache = ImageCache.default
let cached = cache.isCached(forKey: cacheKey)
// To know where the cached image is:
let cacheType = cache.imageCachedType(forKey: cacheKey)
// `.memory`, `.disk` or `.none`.
// Store Image in the cache
let processor = RoundCornerImageProcessor(cornerRadius: 20)
imageView.kf.setImage(with: url, options: [.processor(processor)])
// Later
cache.isCached(forKey: cacheKey, processorIdentifier: processor.identifier)
// Retrieve image from cache
cache.retrieveImage(forKey: "cacheKey") { result in
switch result {
case .success(let value):
print(value.cacheType)
// If the `cacheType is `.none`, `image` will be `nil`.
print(value.image)
case .failure(let error):
print(error)
}
}