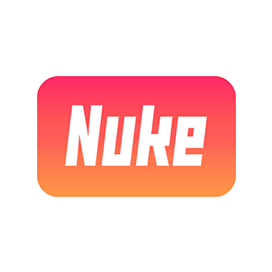
Nuke
Open Source Swift Library for Image Editing & Processing
Free Swift API that allows to customize image pipeline, resize images, use a custom processor, round image corners, download, and cash images & so on.
Nuke is a very useful open source Swift framework that enables software developers to easily load and display images inside their own Swift applications. It requires just one line of code to download and display images in your apps. The library has also included support for several advanced features such as image preheating and progressive decoding that can dramatically enhance the performance of the app as well user experience.
Nuke API is very easy to use and has a very advanced architecture that enables software developers to think of virtually unlimited possibilities for image processing and customization. It has included built-in support for basic image formats such as JPEG, PNG, HEIF, and many more. It also supports image encoding and decoding using Swift code.
The library is very feature-rich and has included several important features for handling their images using Swift commands, such as load images, customizing image pipeline, resizing images, applying filters like Gaussian blur, using a custom processor, round image corners, LRU Memory Cache, HTTP Disk Cache, Aggressive LRU Disk Cache, Reloading Images, image animation, Smart background decompression, downloads Resuming, Request prioritization, Low data mode and many more.
Getting Started with Nuke
The recommended way to install Nuke is using CocoaPods. Please add the following lines to your Podfile
Install Nuke via CocoaPods.
pod "Nuke"
pod "Nuke-Alamofire-Plugin" # optional
pod "Nuke-AnimatedImage-Plugin" # optional
Clone the latest sources using the following command.
Install Nuke via GitHub.
$ git https://github.com/kean/Nuke.git
Download & Use Images using Swift
The open source Nuke API provides a well-organized and effective way for downloading and using images inside your Swift apps. To fetch the images you need to pass the URL of the required image and then call the resume method. It will download the requested image in the background and will inform the response closure when it’s done.
View Images in Different Ways inside Swift Apps
// Load images directly using Async/Await
func loadImage() async throws {
let response = try await pipeline.image(for: url, delegate: self)
}
// Use UI components provided by NukeUI mode
struct ContainerView: View {
var body: some View {
LazyImage(url: URL(string: "https://example.com/image.jpeg"))
}
}
Prefetch Image using Swift API
Prefetching is a very useful feature that enables users to download the image or other data ahead of time in anticipation of its use. The apps can download it and store it in the application’s network cache. Later when you need to view the image the response to your request will come back from the cache instead of the network. The open source API Nuke has included support for an exciting feature known as Prefetching images using Swift code. Please remember that Prefetching can takes up users' data and put extra pressure on the CPU as well as memory. To reduce this pressure you can choose only the disk cache as the prefetching destination.
Prefetch & Cache GitHub using Swift API
inal class PrefetchingDemoViewController: UICollectionViewController {
private let prefetcher = ImagePrefetcher()
private var photos: [URL] = []
override func viewDidLoad() {
super.viewDidLoad()
collectionView?.isPrefetchingEnabled = true
collectionView?.prefetchDataSource = self
}
}
extension PrefetchingDemoViewController: UICollectionViewDataSourcePrefetching {
func collectionView(_ collectionView: UICollectionView, prefetchItemsAt indexPaths: [IndexPath]) {
let urls = indexPaths.map { photos[$0.row] }
prefetcher.startPrefetching(with: urls)
}
func collectionView(_ collectionView: UICollectionView, cancelPrefetchingForItemsAt indexPaths: [IndexPath]) {
let urls = indexPaths.map { photos[$0.row] }
prefetcher.stopPrefetching(with: urls)
}
}
Load and Cash Images via Swift
Image caching is a very useful method that improves application performance and end-user experience. The open source Nuke API enables software apps to automatically cache the downloaded images. Nuke relies on two built-in caching layers. The first one is used to store processed image, which is ready for display. It uses the LRU algorithm – the least recently used entries are removed first during the sweep. The 2nd one uses HTTP Disk Cache to store the unprocessed image data. It is also possible to check the existence of the requested image in the cache.
Read/Write Images in Memory or Disk Cache via Swift API
let cache = pipeline.cache
let request = ImageRequest(url: URL(string: "https://example.com/image.jpeg")!)
cache.cachedImage(for: request) // From any cache layer
cache.cachedImage(for: request, caches: [.memory]) // Only memory
cache.cachedImage(for: request, caches: [.disk]) // Only disk (decodes data)
let data = cache.cachedData(for: request)
cache.containsData(for: request) // Fast contains check
// Stores image in the memory cache and stores an encoded
// image in the disk cache
cache.storeCachedImage(ImageContainer(image: image), for: request)
cache.removeCachedImage(for: request)
cache.removeAll()