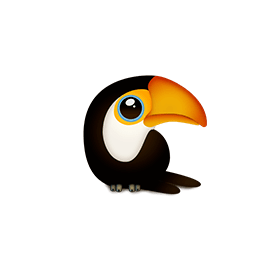
Toucan
Open Source Swift Library for Images Processing
Free Swift API that allows software developers to resize, crop, and stylize your images with ease.
Toucan is a very useful and feature-rich open source Swift library that gives software developers the capability to work with different kinds of image formats inside their own applications. The library is very simple to use and can be easily integrated. The library has provided very flexible methods for interaction firstly you can wrap a single image within the library instance or use a static function for a single operation.
The library has provided a very clean and fast image processing approach and makes it very easy for developers to generate images inside their application with just a couple of lines of code. It has included several important features related to image handling such as generating images, modifying images, smart image resizing, different functions for image masking, crop images, image stylizing, Chainable image processing stages, and many more.
Getting Started with Toucan
Clone the latest sources with using the following command.
Install Toucan via GitHub.
$ git clone https://github.com/gavinbunney/Toucan.git
Image Masking via Swift API
The open source library Toucan allows software developers to apply masks to their images with ease. There are different functions provided that can be used to alter the original image with the mask such as an ellipse, rounded, and image masks. You can also apply the mask on a given image with a path using just a couple of lines of code. The library also allows applying an extra border on the image after the masking effect.
Mask Image using Swift API
// Mask the given image by specifying border width
Toucan(image: myImage).maskWithEllipse(borderWidth: 10, borderColor: UIColor.yellowColor()).image
//Mask the given image with a path
path.moveToPoint(CGPointMake(0, 50))
path.addLineToPoint(CGPointMake(50, 0))
path.addLineToPoint(CGPointMake(100, 50))
path.addLineToPoint(CGPointMake(50, 100))
path.closePath()
Toucan(image: myImage).maskWithPath(path: path).image
Resize Image using Swift API
The Toucan Swift library enables software developers to resize images inside their applications using swift code. The resizing process determines what to do with an image to make it fit the given size bounds. For image resizing, you need to provide the correct path and name of the image. The library has provided support for several operations for resizing images such as image clipping, image cropping, and scaling.
Resize Image via Toucan API
// Resize to fit within the width and height boundaries
let croppedImage = Toucan(image: sourceImage).resize(CGSize(width: 500, height: 500), fitMode: Toucan.Resize.FitMode.Crop).image
// Resize image by Clipping the extra
func ResizeSquareClipped() {
let resized = Toucan(image: maskImage).resize(CGSize(width: 350, height: 350), fitMode: Toucan.Resize.FitMode.clip).image!
XCTAssertEqual(resized.size.width, CGFloat(350), "Verify width not changed")
XCTAssertEqual(resized.size.height, resized.size.width, "Verify height same as width")
}
Image Cropping using Swift
The open source library Toucan has provided complete support for cropping as well as flipping images using swift commands. You need to provide the width and height of the images. It will resize the image to fill the width and height boundaries and crop any excess image data. The library has also included several functions for image flipping, such as flipping images horizontally or vertically as well as both.
Crop Image via Swift API
// Resize image & crops any excess image data
Toucan(image: portraitImage).resize(CGSize(width: 500, height: 500), fitMode: Toucan.Resize.FitMode.Crop).image