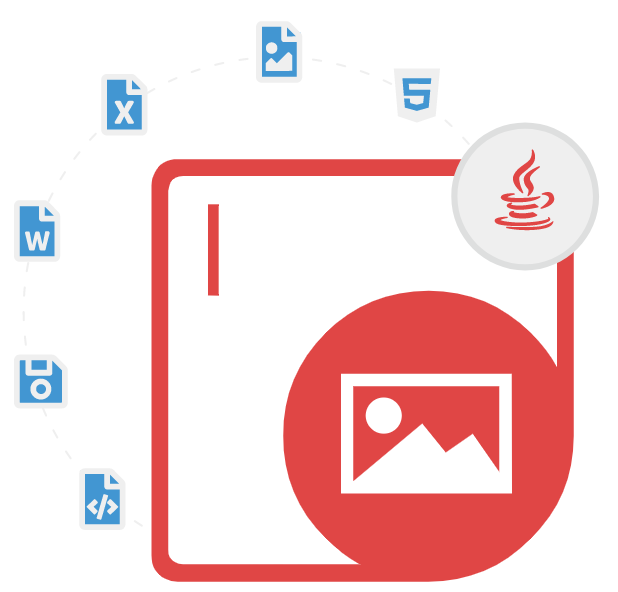
Aspose.Imaging for Java
画像を生成、変更、変換するための Java API
便利な Java API を使用すると、ソフトウェア開発者は画像を作成、圧縮、編集、読み込み、操作し、JPEG、BMP、TIFF、GIF、PNG などに変換できます。
Aspose.Imaging for Java は、ソフトウェア開発者が Java アプリケーション内でさまざまな画像操作タスクを実行できるようにする強力な画像処理ライブラリです。このライブラリを使用すると、ユーザーは画像を簡単に操作したり、さまざまな画像形式間で変換したり、画像のサイズ変更、切り取り、その他の変更を簡単に実行したりできます。このライブラリには、SVG や EMF などのベクター画像のサポートが含まれています。ベクター画像をラスター画像に変換したり、ベクター画像から情報を抽出したりするためのサポートも提供されます。
Aspose.Imaging for Java を使用すると、ソフトウェア開発者は複雑な画像処理タスクを簡単に実行でき、時間と労力を節約できます。このライブラリは、画像の品質を向上させ、ファイル サイズを小さくするための画像の最適化をサポートしています。画像の明るさ、コントラスト、ガンマを調整する機能や、インデックス付き画像のカラー パレットを調整する機能も提供します。また、このライブラリは、JPEG や PNG などの形式の画像のロスレス圧縮とロッシー圧縮もサポートしています。日付と時刻、カメラのメーカーとモデル、露出時間など、画像のさまざまなプロパティの読み取りと書き込みを行う機能を提供します。
Aspose.Imaging for Java は、画像の描画、PDF への画像変換、ベクター画像からベクター化された PSD 画像への変換、透明画像の設定、ベクター画像からベクター化された PSD 画像への変換、透明画像の保存、透明 TIFF から透明 PNG へのエクスポート、Webp から PNG へのエクスポート、画像からの背景の削除、画像の結合、WMF および EMF から他の画像形式への変換、ベクター画像の描画など、画像の操作、変換、最適化、メタデータ管理のための幅広い機能を提供する包括的な画像処理ライブラリです。
Aspose.Imaging for Java を使い始める
Aspose.Imaging for Java をインストールするには、Maven リポジトリを使用することをお勧めします。簡単な構成で、Aspose.Imaging for Java API を Maven プロジェクトで直接簡単に使用できます。
Aspose.Imaging for Java の Maven リポジトリ
//First you need to specify Aspose Repository configuration / location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
//Define Aspose.PDF for Java API Dependency
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging</artifactId>
<version>22.12</version>
<classifier>22.12</classifier>
</dependency>
</dependencies>
ライブラリは、Aspose.Imaging 製品ページから直接ダウンロードできます。
Java アプリ内でイメージを生成および編集
Aspose.Imaging for Java を使用すると、ソフトウェア開発者は、わずか数行の Java コードで最初から新しいイメージを作成できます。ライブラリには、イメージの作成と管理のためのいくつかの重要なクラスが用意されています。BMP、GIF、JPEG、PNG、TIFF、PSD、DICOM、TGA、ICO、EMZ、WMZ など、さまざまな形式でイメージを作成するための多数のオプションがサポートされています。さらに、パスの設定、ストリーム経由のイメージの作成、イメージのサイズ変更、イメージ上のオブジェクトの描画、イメージの内容の更新とディスクへのイメージの保存、イメージの明るさの調整、イメージへのコントラストまたはガンマの適用、イメージへのぼかし効果の適用、イメージの透明度のチェックなどによってイメージを作成できます。
Java API 経由でパスを設定して画像を作成しますか?
// The path to the documents directory.
String dataDir = "D:/dataDir/";
// Creates an instance of BmpOptions and set its various properties
BmpOptions imageOptions = new BmpOptions();
imageOptions.setBitsPerPixel(24);
// Define the source property for the instance of BmpOptions Second boolean parameter determines if the file is temporal or not
imageOptions.setSource(new FileCreateSource(dataDir + "CreatingAnImageBySettingPath_out.bmp", false));
try
{
// Creates an instance of Image and call Create method by passing the BmpOptions object
try (Image image = Image.create(imageOptions, 500, 500))
{
image.save(dataDir + "CreatingAnImageBySettingPath1_out.bmp");
}
}
finally
{
imageOptions.close();
}
Java API による他の形式への画像変換
Aspose.Imaging for Java を使用すると、ソフトウェア開発者は Java コマンドを使用してさまざまな種類の画像を他のサポートされているファイル形式に変換できます。ライブラリには、JPEG、BMP、TIFF、GIF、PNG、DICOM、TGA、ICO、EMZ、WMZ、WebP、SVG など、画像をある形式から別の形式に変換するための関数がいくつか用意されています。ライブラリでは、画像を複数ページの TIFF に変換したり、TIFF の個々のページを別の画像として保存したり、画像を PDF に変換したりすることもできます。
Java API 経由で TIFF 画像を JPEG 画像に変換する
// The path to the documents directory.
String dataDir = Utils.getSharedDataDir(ConvertTIFFToJPEG.class) + "ManipulatingJPEGImages/";
TiffImage tiffImage = (TiffImage)Image.load(dataDir + "source2.tif");
try
{
int i = 0;
for (TiffFrame tiffFrame : tiffImage.getFrames())
{
JpegOptions saveOptions = new JpegOptions();
saveOptions.setResolutionSettings(new ResolutionSetting(tiffFrame.getHorizontalResolution(), tiffFrame.getVerticalResolution()));
TiffOptions frameOptions = tiffFrame.getFrameOptions();
if (frameOptions != null)
{
// Set the resolution unit explicitly.
switch (frameOptions.getResolutionUnit())
{
case TiffResolutionUnits.None:
saveOptions.setResolutionUnit(ResolutionUnit.None);
break;
case TiffResolutionUnits.Inch:
saveOptions.setResolutionUnit(ResolutionUnit.Inch);
break;
case TiffResolutionUnits.Centimeter:
saveOptions.setResolutionUnit(ResolutionUnit.Cm);
break;
default:
throw new RuntimeException("Current resolution unit is unsupported!");
}
}
String fileName = "source2.tif.frame." + (i++) + "."
+ ResolutionUnit.toString(ResolutionUnit.class, saveOptions.getResolutionUnit()) + ".jpg";
tiffFrame.save(dataDir + fileName, saveOptions);
}
}
finally
{
tiffImage.close();
}
Java API による画像の操作
Aspose.Imaging for Java を使用すると、コンピューター プログラマーは既存の画像に簡単にアクセスして操作できます。ライブラリには、画像プロパティの更新、ベクター グラフィックスの描画、複数ページの画像の処理、画像の背景の削除または更新、画像の結合 (JPG から JPG、JPG から PDF の結合、JPG から PNG)、画像の切り取り、画像の回転、画像のサイズ変更、画像の傾き補正、画像への透かしの追加、ベクター画像へのラスター画像の描画など、画像操作を処理するための関数がいくつかあります。
Java API 経由で画像に中央値フィルターを適用する
// Load the noisy image
Image image = Image.load(dataDir + "aspose-logo.gif");
// caste the image into RasterImage
RasterImage rasterImage = (RasterImage) image;
if (rasterImage == null)
{
return;
}
// Create an instance of MedianFilterOptions class and set the size.
MedianFilterOptions options = new MedianFilterOptions(4);
// Apply MedianFilterOptions filter to RasterImage object.
rasterImage.filter(image.getBounds(), options);
// Save the resultant image
image.save(dataDir + "median_test_denoise_out.gif");
Java API による画像の回転とサイズ変更
Aspose.Imaging for Java を使用すると、ソフトウェア開発者は独自の Java アプリケーション内でプログラムによって画像を回転およびサイズ変更できます。切り取りは、画像の一部を切り取って特定の領域に焦点を合わせるために使用できる非常に便利な手法です。ライブラリには、シフトによる画像の切り取り、長方形による画像の切り取り、ベクター画像の切り取り、90/180/270 度による画像の回転、水平または垂直方向の画像の反転、指定した角度での画像の回転、WebP 画像のサイズ変更、比例した画像のサイズ変更など、画像の回転とサイズ変更に関連するいくつかの機能が用意されています。
Java API 経由でシフトによって画像をトリミングする方法
u// The path to the documents directory.
String dataDir = "dataDir/jpeg/";
// Load an existing image into an instance of RasterImage class
try (RasterImage rasterImage = (RasterImage)Image.load(dataDir + "aspose-logo.jpg"))
{
// Before cropping, the image should be cached for better performance
if (!rasterImage.isCached())
{
rasterImage.cacheData();
}
// Define shift values for all four sides
int leftShift = 10;
int rightShift = 10;
int topShift = 10;
int bottomShift = 10;
// Based on the shift values, apply the cropping on image Crop method will shift the image bounds toward the center of image and Save the results to disk
rasterImage.crop(leftShift, rightShift, topShift, bottomShift);
rasterImage.save(dataDir + "CroppingByShifts_out.jpg");
}