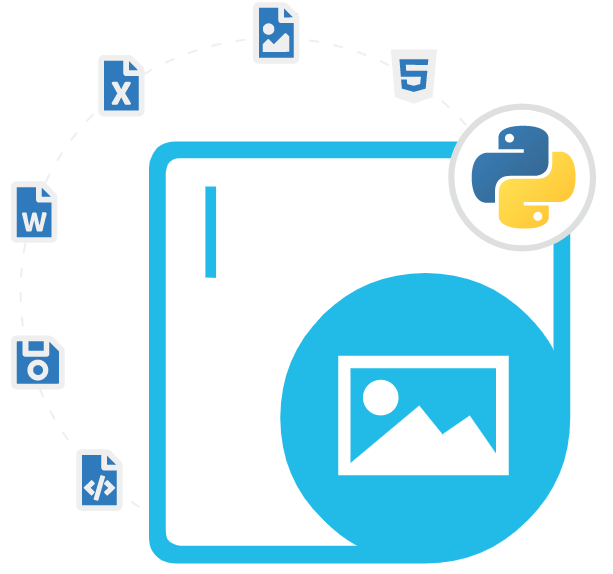
Aspose.Imaging Cloud SDK for Python
画像を作成および変換するための Python REST API
画像処理用の Python REST SDK を使用すると、ソフトウェア開発者は画像を作成、編集、圧縮、操作、変換、圧縮できます。
画像は現代のコミュニケーションに欠かせない要素であり、ソーシャル メディア、広告、ヘルスケアなどの分野で使用されています。画像を効率的かつ正確に作成および処理することは、多くの現代のビジネスにとって非常に重要な要件です。幸いなことに、Aspose.Imaging Cloud SDK for Python は、画像を操作する包括的なソリューションを提供し、ソフトウェア開発者が作成、変換、サイズ変更、トリミング、表示、印刷などのさまざまな重要なタスクを実行できるようにします。Aspose.Imaging Cloud SDK for Python の主な利点の 1 つは、ソフトウェア開発者が特別なソフトウェアやハードウェアを必要とせずに画像を処理できることです。
Aspose.Imaging Cloud SDK for Python は、開発者が BMP、GIF、JPEG、JPEG2000、PSD、TIFF、WEBP、PNG、WMF、EMF、SVG など、さまざまな形式のさまざまなタイプの画像を操作できるようにするクラウドベースの API です。ライブラリには、画像からのメタデータの抽出、カラー管理のサポート、画像内の複数のレイヤーの操作、レイヤーの追加/削除または変更、画像への透かしの追加 (テキストおよび画像の透かし)、画像の反転と回転、画像の向きの問題の修正、画像の視点の変更など、その他の重要な機能もいくつか含まれています。
Aspose.Imaging Cloud SDK for Python は非常に扱いやすく、開発者は PNG 画像を JPEG または BMP に変換するなど、画像をある形式から別の形式に簡単に変換できます。SDK はさまざまな画像形式をサポートしているため、非常に汎用性があります。SDK のもう 1 つの非常に便利な機能は、ユーザーが SDK を使用して画像のサイズを変更できることです。これは、ソーシャル メディアの投稿や Web サイトのデザインなど、特定の目的に合わせて画像を最適化するのに役立ちます。SDK は、比例サイズ変更と非比例サイズ変更の両方をサポートしています。さらに、ユーザーは画像をトリミングして不要な部分を削除したり、特定の関心領域に焦点を合わせたりできます。ユーザーは、トリミング領域やトリミング モードなどのトリミング パラメーターを指定できます。
Aspose.Imaging Cloud SDK for Python を使い始める
Aspose.Imaging Cloud SDK for Python をインストールするには、PyPi を使用することをお勧めします。スムーズにインストールするには、次のコマンドを使用してください。
PyPi 経由で Aspose.Imaging Cloud SDK for Python をインストールする
pip install aspose-imaging-cloud
Aspose 製品ページから直接ダウンロードすることもできます。Python API 経由でクラウド内のイメージの読み取りと書き込みを行う
Aspose.Imaging Cloud SDK for Python は、非常に便利な REST API です。これを使用すると、コンピューター プログラマーは、初期コストなしで、クラウド内での作成、操作、変換などの幅広いイメージ処理操作を実行できます。ライブラリには、いくつかの一般的なイメージ ファイル形式のサポートが含まれており、BMP、GIF、JPEG、JPEG2000、PSD、TIFF、WEBP、PNG、WMF、EMF、SVG、TGA、APNG などのイメージ ファイル形式の読み取りと書き込みが可能です。イメージが作成されると、ソフトウェア開発者は必要に応じて簡単にイメージをロードして変更できます。次の例は、ユーザーがクラウド ストレージからイメージを読み取る方法を示しています。
Python 経由でクラウド ストレージに画像を読み書きする方法
# set the input image path and format
name = 'input_image.jpg'
format = 'jpg'
folder = 'your_folder_path'
# send the request to the API to download the image
response = imaging_api.get_image_download(name, folder=folder, format=format)
# read the image data from the response
image_data = response.content
# Write an Image to the Cloud Storage via Python API
# set the output image path and format
name = 'output_image.jpg'
format = 'jpg'
folder = 'your_folder_path'
# send the request to the API to upload the image
response = imaging_api.create_updated_image(name, image_data, folder=folder, format=format)
# read the response to confirm the image was uploaded successfully
if response.status_code == 200:
print('Image uploaded successfully.')
else:
print('Error uploading image:', response.content)
Python API による画像のサイズ変更、切り取り、回転
Aspose.Imaging Cloud SDK for Python を使用すると、ソフトウェア開発者は独自のクラウド アプリケーション内でさまざまな画像操作を実行できます。サイズ変更タスクを実行するには、開発者は画像を Cloud Storage にアップロードし、その名前を API URL に渡す必要があります。画像パラメータを更新すると、API は更新された画像を応答で返します。REST API には、画像の回転、反転、画像の拡大縮小、既存の画像の切り取り、TIFF 画像の追加など、その他の重要な機能もいくつか含まれています。
Python API を使用して画像のサイズを変更またはトリミングする方法
import asposeimagingcloudsdk
from asposeimagingcloudsdk.models.requests import CreateResizedImageRequest, CreateCroppedImageRequest
# Initialize Aspose.Imaging Cloud API client
imaging_api = asposeimagingcloudsdk.ImagingApi(api_key='YOUR_API_KEY', app_sid='YOUR_APP_SID')
# Set the input image file name and format
filename = 'input_image.jpg'
format = 'jpg'
# Set the output image file name and format
output_filename = 'output_image.jpg'
output_format = 'jpg'
# Set the new size for the resized image
new_width = 500
new_height = 500
# Set the coordinates and size of the area to be cropped
x = 50
y = 50
width = 400
height = 400
# Create a request object for creating the resized image
resize_request = CreateResizedImageRequest(filename, new_width, new_height, format, output_format, folder='input')
# Call the API to resize the image and save the result to the cloud storage
response = imaging_api.create_resized_image(resize_request)
# Create a request object for creating the cropped image
crop_request = CreateCroppedImageRequest(output_filename, output_format, x, y, width, height, format, folder='output')
# Call the API to crop the image and save the result to the cloud storage
response = imaging_api.create_cropped_image(crop_request)
Python API 経由で TIFF フレームを操作する
Aspose.Imaging Cloud SDK for Python には、Python アプリケーション内での TIFF (タグ付き画像ファイル形式) 画像に対する非常に強力なサポートが含まれています。ライブラリには、マルチフレーム TIFF 画像からのフレームの抽出、TIFF フレームのプロパティの取得、TIFF フレームのサイズ変更、TIFF フレームの回転または反転のサポート、TIFF フレームの切り取り、別の TIFF 画像への TIFF フレームの追加、さらに処理するための個々の TIFF フレームの抽出など、TIFF ファイル形式を処理するための重要な機能がいくつかあります。
クラウド アプリでの高度な画像検索
Aspose.Imaging Cloud SDK for Python には、Python クラウド アプリケーション内でさまざまな方法で画像を検索するための非常に強力なサポートが含まれています。このライブラリを使用すると、ソフトウェア開発者は、少なくとも 1 つの画像を含むソース画像セットを他の複数の画像と比較する逆画像検索を実行できます。ソフトウェア開発者は、2 つの画像の比較、検索コンテキストからの画像の取得、検索コンテキストでの画像機能の更新、類似画像の検索、重複画像の検索、タグによる画像の検索などの操作を実行できます。
Python API で重複画像を見つける方法
# optional parameters are base URL, API version and debug mode
imaging_api = ImagingApi('yourClientSecret', 'yourClientId')
# create search context or use existing search context ID if search context was
# created earlier
api_response = imaging_api.create_image_search(CreateImageSearchRequest())
search_context_id = api_response.id
# extract images features if it was not done before
imaging_api.create_image_features(CreateImageFeaturesRequest(
search_context_id, image_id=None, images_folder='WorkFolder'))
# wait 'till image features extraction is completed
while imaging_api.get_image_search_status(
GetImageSearchStatusRequest(
search_context_id)).search_status != 'Idle':
time.sleep(10)
# request finding duplicates
response = imaging_api.find_image_duplicates(
FindImageDuplicatesRequest(search_context_id, 90))
# process duplicates search result
for duplicates in response.duplicates:
print('Duplicates:')
for duplicate in duplicates.duplicate_images:
print('ImageName: {0}, Similarity: {1}'.format(duplicate.image_id,
duplicate.similarity))