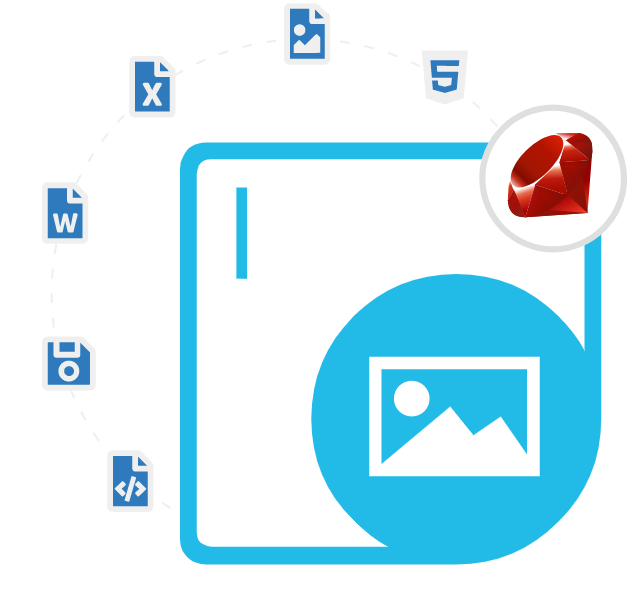
Aspose.Imaging Cloud SDK for Ruby
画像を作成、サイズ変更、回転、変換するための Ruby API
ソフトウェア開発者がさまざまな画像形式のサイズ変更、切り取り、操作、変換を行える強力なクラウドベースの画像処理API.
Aspose.Imaging Cloud SDK for Ruby は、非常に便利なクラウドベースの画像処理 API です。ソフトウェア開発者は、これを使用して Ruby アプリケーション内でさまざまな画像ファイル形式を操作および変換できます。SDK は、サイズ変更、切り取り、回転、反転、フィルタリングなど、包括的な画像処理操作を提供します。SDK は、画像の品質を損なうことなく画像のサイズを縮小するために使用できるさまざまな最適化手法を提供します。
Aspose.Imaging Cloud SDK for Ruby は、画像プロパティの取得、画像のサイズ変更と他の形式への保存、画像の別の形式への変換、TIFF 画像の追加、画像の傾き補正、画像へのフィルターの適用、複数ページの画像からのフレーム範囲の取得、画像の逆検索など、ソフトウェア開発者がアプリケーション内で画像を処理および変換できるようにする幅広い機能を提供します。さらに、ソフトウェア プログラマーは、SDK を使用して、BMP、JPEG、PNG、GIF、TIFF、PSD などの形式から別の形式に画像を簡単に変換できます。
Aspose.Imaging Cloud SDK for Ruby には、BMP、JPEG、PNG、GIF、JPEG2000、WEBP、PNG、WMF、EMF、SVG、TIFF、CMX、PSD など、さまざまな画像形式がサポートされています。これは、世界中のどこからでもアクセスできるクラウド ベースの API です。開発者は、API を使用するためにローカル マシンにソフトウェアをインストールする必要はありません。Ruby アプリケーション用のイメージング API をお探しの場合は、Aspose.Imaging Cloud SDK for Ruby を検討する価値があります。
Aspose.Imaging Cloud SDK for Ruby を使い始める
Aspose.Imaging Cloud SDK for Ruby をインストールするには、RubyGems を使用することをお勧めします。スムーズにインストールするには、次のコマンドを使用してください。
RubyGems 経由で Aspose.Imaging Cloud SDK for Ruby をインストールする
gem build aspose-imaging-cloud.gemspec
Aspose 製品ページから直接ダウンロードすることもできます。Ruby API による画像のサイズ変更と切り取り
Aspose.Imaging Cloud SDK for Ruby は、Ruby アプリケーション内で画像のサイズ変更やその他の関連操作を処理するための完全なサポートを提供します。API には、画像の切り取り、切り取り四角形の位置と寸法の指定、切り取り画像の形式の変更、画像の幅の更新、画像の高さの変更、既存の画像のサイズの変更と別の形式での保存など、さまざまなサポートが含まれています。次の例は、Ruby アプリケーション内で SDK を使用して画像のサイズを変更する方法を示しています。
Ruby SDK を使用して画像のサイズを変更する方法
require 'aspose_imaging_cloud'
# Initialize the API client
imaging_api = AsposeImagingCloud::ImagingApi.new
# Set the image file name
name = 'image.png'
# Set the new size
new_width = 200
new_height = 200
# Call the resize_image API method
response = imaging_api.resize_image(name, new_width, new_height)
# Save the resized image
File.write('resized_image.png', response.content)
Ruby による画像の他の形式への変換
Aspose.Imaging Cloud SDK for Ruby には、BMP、JPEG、PNG、GIF、TIFF、PSD など、さまざまな画像形式がサポートされています。SDK には、BMP、GIF、DJVU、WMF、EMF、JPEG、JPEG2000、PSD、TIFF、WEBP、PNG、DICOM、CDR、CMX、ODG、DNG、SVG などの一般的な画像ファイル形式の読み込み、表示、変換のサポートが含まれています。ソフトウェア開発者は、入力および出力画像の名前、形式、および入力フォルダーと出力フォルダーなどのその他のパラメーターを指定することもできます。プログラマーは、クラウド ストレージに画像を簡単にアップロードし、クラウド内で目的の画像形式に変換できます。ソフトウェア開発者が Ruby Cloud SDK を使用して画像を別の形式に変換する方法を示すサンプル コードを以下に示します。
Ruby API を使用して画像を別の形式に変換する方法
require 'aspose_imaging_cloud'
# Configure Aspose.Imaging Cloud API credentials
config = AsposeImagingCloud::Configuration.new
config.client_id = 'Your App SID'
config.client_secret = 'Your App Key'
# Create an instance of the API client
imaging_api = AsposeImagingCloud::ImagingApi.new(config)
# Set the input image name and format
name = 'input.jpg'
format = 'png'
# Set the output image name and format
output_name = 'output.png'
# Convert the input image to the output format
imaging_api.convert_image(AsposeImagingCloud::ConvertImageRequest.new(name, format, output_format: output_format, folder: 'input_folder', out_path: 'output_folder/' + output_name))
puts 'Image converted successfully.'
Ruby API 経由で画像にフィルターを適用する
Aspose.Imaging Cloud SDK for Ruby を使用すると、ソフトウェア開発者は Ruby アプリケーション内の既存の画像にフィルターを適用できます。ライブラリには、BigRectangular、SmallRectangular、Median、GaussWiener、MotionWiener、GaussianBlur、Sharpen、BilateralSmoothing など、いくつかの重要なフィルター タイプが含まれています。次の例では、半径 2.0、スムース 2.0 で GaussWiener フィルターが画像に適用されています。出力画像形式は PNG で、出力画像はクラウド ストレージの「出力」フォルダーに保存されます。
Ruby SDK を使用して画像にフィルターを適用する
filter_type = 'GaussWiener'
filter_properties = {
radius: 2.0,
smooth: 2.0
}
output_format = 'png'
response = imaging_api.apply_filter(
AsposeImagingCloud::ApplyFilterRequest.new(
name: source_image,
filter_type: filter_type,
filter_properties: filter_properties,
format: output_format,
folder: 'output'
)
)
// Download the output image:
output_image = 'output_image.png'
response = imaging_api.download_file(
AsposeImagingCloud::DownloadFileRequest.new(
path: "output/#{output_image}"
)
)
File.open(output_image, 'wb') do |file|
file.write(response)
end