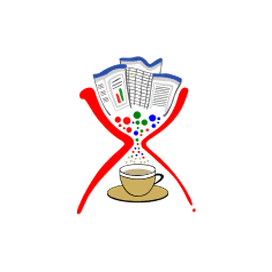
Apache POI XWPF
Word OOXML ドキュメント用のJava API
Java アプリケーションで Microsoft Word DOCX ファイルを作成、読み取り、編集、変換するオープン ソース ソリューション。
Apache POI XWPF 入門
まず、システムに Java Development Kit (JDK) をインストールする必要があります。既にお持ちの場合は、Apache POI の ダウンロード ページに進み、最新の安定版リリースをアーカイブから入手してください。必要なライブラリーを Java プログラムにリンクできる任意のディレクトリーに ZIP ファイルの内容を抽出します。それだけです!
Maven ベースの Java プロジェクトで Apache POI を参照するのはさらに簡単です。必要なのは、pom.xml に次の依存関係を追加し、IDE が Apache POI Jar ファイルを取得して参照できるようにすることだけです。
Apache POI Maven の依存関係
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.0</version>
</dependency>
Java API を使用して Word 文書を生成および編集する
Apache POI XWPF を使用すると、ソフトウェア プログラマーは新しい Word ドキュメントを DOCX ファイル形式で作成できます。開発者は、既存の Microsoft Word DOCX ファイルを読み込んで、アプリケーションのニーズに合わせて編集することもできます。新しい段落の追加、テキストの挿入、テキストの配置と境界線の適用、テキスト スタイルの変更などを行うことができます。
最初から DOCX ファイルを生成する
// initialize a blank document
XWPFDocument document = new XWPFDocument();
// create a new file
FileOutputStream out = new FileOutputStream(new File("document.docx"));
// create a new paragraph paragraph
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
run.setText("File Format Developer Guide - " +
"Learn about computer files that you come across in " +
"your daily work at: www.fileformat.com ");
document.write(out);
out.close();
Word 文書に段落、画像、表を追加する
Apache POI XWPF を使用すると、開発者は段落と画像を Word ドキュメントに追加できます。この API は、DOCX ドキュメントにテーブルを追加する機能も提供し、ユーザー定義データを使用して単純なネストされたテーブルを作成できるようにします。
表を含む新しい DOCX ファイルを作成する
// initialize a blank document
XWPFDocument document = new XWPFDocument();
// create a new file
FileOutputStream out = new FileOutputStream(new File("table.docx"));
// create a new table
XWPFTable table = document.createTable();
// create first row
XWPFTableRow tableRowOne = table.getRow(0);
tableRowOne.getCell(0).setText("Serial No");
tableRowOne.addNewTableCell().setText("Products");
tableRowOne.addNewTableCell().setText("Formats");
// create second row
XWPFTableRow tableRowTwo = table.createRow();
tableRowTwo.getCell(0).setText("1");
tableRowTwo.getCell(1).setText("Apache POI XWPF");
tableRowTwo.getCell(2).setText("DOCX, HTML, FO, TXT, PDF");
// create third row
XWPFTableRow tableRowThree = table.createRow();
tableRowThree.getCell(0).setText("2");
tableRowThree.getCell(1).setText("Apache POI HWPF");
tableRowThree.getCell(2).setText("DOC, HTML, FO, TXT");
document.write(out);
out.close();
Word OOXML ドキュメントからテキストを抽出する
Apache POI XWPF は、わずか数行のコードで Microsoft Word DOCX ドキュメントからデータを抽出する特殊なクラスを提供します。同様に、Word ファイルから見出し、脚注、表データなどを抽出することもできます。
Word ファイルからテキストを抽出する
// load DOCX file
FileInputStream fis = new FileInputStream("document.docx");
// open file
XWPFDocument file = new XWPFDocument(OPCPackage.open(fis));
// read text
XWPFWordExtractor ext = new XWPFWordExtractor(file);
// display text
System.out.println(ext.getText());
カスタム ヘッダーとフッターを DOCX ドキュメントに追加する
ヘッダーとフッターは通常、日付、ページ番号、作成者名、脚注などの追加情報が含まれているため、Word 文書の重要な部分であり、長い文書を整理して読みやすくするのに役立ちます。 Apache POI XWPF を使用すると、Java 開発者はカスタム ヘッダーとフッターを Word ドキュメントに追加できます。
Word DOCX ファイルでカスタム ヘッダーとフッターを管理する
public class HeaderFooterTable {
public static void main(String[] args) throws IOException {
try (XWPFDocument doc = new XWPFDocument()) {
// Create a header with a 1 row, 3 column table
XWPFHeader hdr = doc.createHeader(HeaderFooterType.DEFAULT);
XWPFTable tbl = hdr.createTable(1, 3);
// Set the padding around text in the cells to 1/10th of an inch
int pad = (int) (.1 * 1440);
tbl.setCellMargins(pad, pad, pad, pad);
// Set table width to 6.5 inches in 1440ths of a point
tbl.setWidth((int) (6.5 * 1440));
CTTbl ctTbl = tbl.getCTTbl();
CTTblPr ctTblPr = ctTbl.addNewTblPr();
CTTblLayoutType layoutType = ctTblPr.addNewTblLayout();
layoutType.setType(STTblLayoutType.FIXED);
BigInteger w = new BigInteger("3120");
CTTblGrid grid = ctTbl.addNewTblGrid();
for (int i = 0; i < 3; i++) {
CTTblGridCol gridCol = grid.addNewGridCol();
gridCol.setW(w);
}
// Add paragraphs to the cells
XWPFTableRow row = tbl.getRow(0);
XWPFTableCell cell = row.getCell(0);
XWPFParagraph p = cell.getParagraphArray(0);
XWPFRun r = p.createRun();
r.setText("header left cell");
cell = row.getCell(1);
p = cell.getParagraphArray(0);
r = p.createRun();
r.setText("header center cell");
cell = row.getCell(2);
p = cell.getParagraphArray(0);
r = p.createRun();
r.setText("header right cell");
// Create a footer with a Paragraph
XWPFFooter ftr = doc.createFooter(HeaderFooterType.DEFAULT);
p = ftr.createParagraph();
r = p.createRun();
r.setText("footer text");
try (OutputStream os = new FileOutputStream(new File("headertable.docx"))) {
doc.write(os);
}
}
}
}