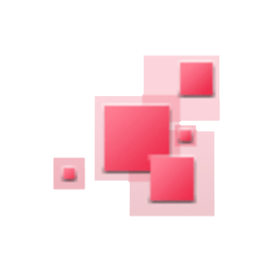
PDFsharp
PDF 처리를 위한 오픈소스 .NET API
무료 C# .NET 라이브러리를 통해 PDF 문서를 생성, 조작, 변환 및 처리합니다.
PDFsharp는 무엇입니까?
PDF 파일의 텍스트 또는 메타 데이터를 생성, 렌더링, 병합, 분할, 수정, 인쇄 및 추출하는 데 사용할 수 있는 오픈 소스 .NET 라이브러리입니다. PDFsharp API는 모든 .NET 언어에서 즉석에서 PDF 문서 생성을 지원합니다. 또한 XML 파일 또는 직접 인터페이스를 통해 다양한 소스에서 데이터 가져오기를 지원합니다. 페이지 레이아웃, 텍스트 서식 및 문서 디자인을 위한 다양한 옵션을 지원합니다.
PDFsharp는 GDI+ 또는 WPF를 기반으로 하는 그래픽 구현을 제공합니다. API는 하나의 소스 코드를 사용하여 PDF 페이지는 물론 창이나 프린터에서도 그릴 수 있는 기능을 제공하여 개발자의 작업을 쉽게 만듭니다. PDF 수정, PDF 병합 또는 분할, XPS에서 PDF로 변환, PDF 렌더링, PDF 데이터 추출, 글꼴 포함 및 하위 설정, 유니코드 지원 등과 같은 PDF 파일 처리를 위한 몇 가지 중요한 기능을 지원합니다.
PDFsharp 시작하기
PDFsharp는 AGPL/상용 소프트웨어로 이중 라이선스가 부여됩니다. AGPL은 무료/오픈 소스 소프트웨어 라이선스입니다.
NuGet을 사용하여 프로젝트에 PDFsharp를 추가하는 것이 좋습니다.
NuGet 명령
Install-Package PdfSharp
Visual Studio를 사용하면 NuGet 패키지 관리자를 설치하여 NuGet 패키지에 쉽게 액세스할 수 있습니다. VS 2012 Express는 물론 VS 2013 및 VS 2015의 커뮤니티 에디션에서도 작동합니다. Visual Studio에서 "Tools" => "확장 및 업데이트..."를 클릭하여 아직 설치하지 않은 경우 NuGet 패키지 관리자를 설치합니다. NuGet 패키지 관리자가 패키지를 다운로드하여 설치하고 프로젝트에 대한 참조를 추가합니다.
무료 .NET API를 통해 PDF 문서 생성 및 수정
소프트웨어 개발자는 PDFsharp API를 사용하여 자신의 .NET 응용 프로그램 내에서 새 PDF 문서를 만들 수 있습니다. 문서가 생성되면 빈 페이지를 추가하고 그래픽이나 텍스트를 쉽게 삽입할 수 있습니다. 또한 개발자는 필요에 따라 기존 문서를 수정하고 원하는 이름으로 저장할 수 있습니다. 다음 단계를 사용하여 C#에서 PDF 문서를 생성 및 조작할 수 있습니다.
- PDF 문서 초기화
- 페이지 추가
- 그리기용 XGraphics 개체 가져오기
- 글꼴 만들기
- 텍스트 추가
- 문서 저장
C#을 사용하여 PDF 만들기
// Create a new PDF document
PdfDocument pdfDocument = new PdfDocument();
// Create an empty page
PdfPage pdfPage = pdfDocument.AddPage();
// Get an XGraphics object for drawing
XGraphics xGraphics = XGraphics.FromPdfPage(pdfPage);
// Create a font
XFont xFont = new XFont("Verdana", 20, XFontStyle.BoldItalic);
// Draw the text
xGraphics.DrawString("File Format Developer Guide", xFont, XBrushes.Black,
new XRect(0, 0, pdfPage.Width, pdfPage.Height),
XStringFormats.Center);
// Save the document...
pdfDocument.Save("fileformat.pdf");
.NET API를 통해 PDF 주석 생성
주석을 사용하면 PDF 페이지에 사용자 정의 콘텐츠를 추가할 수 있습니다. PDF 응용 프로그램을 사용하면 일반적으로 텍스트, 선, 메모 또는 모양 등과 같은 다양한 유형의 주석을 만들고 수정할 수 있습니다. PDFsharp를 사용하면 프로그래머는 자신의 응용 프로그램 내에서 다양한 유형의 PDF 주석을 만들 수 있습니다. 라이브러리는 텍스트 주석, 링크 및 고무 스탬프 주석 생성을 지원합니다.
C#을 통해 PDF 텍스트 주석 만들기
// Create a PDF text annotation
PdfTextAnnotation textAnnot = new PdfTextAnnotation();
textAnnot.Title = "This is the title";
textAnnot.Subject = "This is the subject";
textAnnot.Contents = "This is the contents of the annotation.\rThis is the 2nd line.";
textAnnot.Icon = PdfTextAnnotationIcon.Note;
gfx.DrawString("The first text annotation", font, XBrushes.Black, 30, 50, XStringFormats.Default);
// Convert rectangle from world space to page space. This is necessary because the annotation is
// placed relative to the bottom left corner of the page with units measured in point.
XRect rect = gfx.Transformer.WorldToDefaultPage(new XRect(new XPoint(30, 60), new XSize(30, 30)));
textAnnot.Rectangle = new PdfRectangle(rect);
// Add the annotation to the page
page.Annotations.Add(textAnnot);
.NET을 통해 여러 PDF 문서 결합
하나의 큰 문서로 결합해야 하는 수많은 PDF 문서가 있습니까? PDFsharp API는 몇 줄의 코드로 여러 PDF 파일을 단일 파일로 결합하는 기능을 제공합니다. 개발자는 기존 PDF 파일에서 새 문서를 쉽게 만들 수 있습니다. 이것은 시각적 비교 또는 기타 여러 중요한 작업에 유용할 수 있습니다.
Java를 통해 문서 결합
// Open the input files
PdfDocument inputDocument1 = PdfReader.Open(filename1, PdfDocumentOpenMode.Import);
PdfDocument inputDocument2 = PdfReader.Open(filename2, PdfDocumentOpenMode.Import);
// Create the output document
PdfDocument outputDocument = new PdfDocument();
// Show consecutive pages facing. Requires Acrobat 5 or higher.
outputDocument.PageLayout = PdfPageLayout.TwoColumnLeft;
XFont font = new XFont("Verdana", 10, XFontStyle.Bold);
XStringFormat format = new XStringFormat();
format.Alignment = XStringAlignment.Center;
format.LineAlignment = XLineAlignment.Far;
XGraphics gfx;
XRect box;
int count = Math.Max(inputDocument1.PageCount, inputDocument2.PageCount);
for (int idx = 0; idx < count; idx++)
{
// Get page from 1st document
PdfPage page1 = inputDocument1.PageCount > idx ?
inputDocument1.Pages[idx] : new PdfPage();
// Get page from 2nd document
PdfPage page2 = inputDocument2.PageCount > idx ?
inputDocument2.Pages[idx] : new PdfPage();
// Add both pages to the output document
page1 = outputDocument.AddPage(page1);
page2 = outputDocument.AddPage(page2);
// Write document file name and page number on each page
gfx = XGraphics.FromPdfPage(page1);
box = page1.MediaBox.ToXRect();
box.Inflate(0, -10);
gfx.DrawString(String.Format("{0} • {1}", filename1, idx + 1),
font, XBrushes.Red, box, format);
gfx = XGraphics.FromPdfPage(page2);
box = page2.MediaBox.ToXRect();
box.Inflate(0, -10);
gfx.DrawString(String.Format("{0} • {1}", filename2, idx + 1),
font, XBrushes.Red, box, format);
}
// Save the document...
const string filename = "CompareDocument1_tempfile.pdf";
outputDocument.Save(filename);