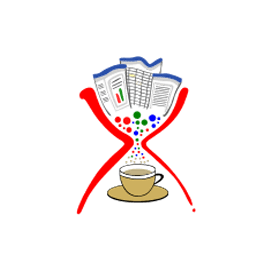
Apache POI XWPF
Word OOXML 문서용 자바 API
자바 애플리케이션에서 Microsoft Word DOCX 파일을 생성, 읽기, 수정 및 변환하는 오픈 소스 솔루션입니다.
Apache POI XWPF는 Microsoft Word 2007 DOCX 파일 형식을 읽고 쓰는 기능을 제공합니다. XWPF에는 Word DOCX 파일의 주요 부분에 대한 액세스를 제공하는 상당히 안정적인 핵심 API가 있습니다. 기본 및 특정 텍스트 추출, 머리글 및 바닥글 조작, 텍스트 조작 및 스타일 지정 기능에 사용할 수 있습니다.
Apache POI XWPF는 Microsoft Word 파일 생성 및 문서 편집, 텍스트 및 단락 서식 지정, 이미지 삽입, 테이블 생성 및 구문 분석, 메일 병합 기능, 양식 요소 관리 등으로 더 유명합니다.
Apache POI XWPF 시작하기
먼저 시스템에 JDK(Java Development Kit)가 설치되어 있어야 합니다. 이미 가지고 있는 경우 Apache POI의 다운로드 페이지로 이동하여 아카이브에서 최신 안정 릴리스를 얻으십시오. 필요한 라이브러리가 Java 프로그램에 링크될 수 있는 디렉토리에서 ZIP 파일의 컨텐츠를 추출하십시오. 그게 다야!
Maven 기반 Java 프로젝트에서 Apache POI를 참조하는 것은 훨씬 더 간단합니다. pom.xml에 다음 종속성을 추가하고 IDE가 Apache POI Jar 파일을 가져와 참조하도록 하기만 하면 됩니다.
Apache POI Maven 종속성
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.0</version>
</dependency>
Java API를 사용하여 Word 문서 생성 및 편집
Apache POI XWPF를 사용하면 소프트웨어 프로그래머가 DOCX 파일 형식으로 새 Word 문서를 만들 수 있습니다. 개발자는 기존 Microsoft Word DOCX 파일을 로드하여 응용 프로그램 요구 사항에 따라 편집할 수도 있습니다. 새 단락을 추가하고, 텍스트를 삽입하고, 텍스트 정렬 및 테두리를 적용하고, 텍스트 스타일을 변경하는 등의 작업을 수행할 수 있습니다.
처음부터 DOCX 파일 생성
// initialize a blank document
XWPFDocument document = new XWPFDocument();
// create a new file
FileOutputStream out = new FileOutputStream(new File("document.docx"));
// create a new paragraph paragraph
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
run.setText("File Format Developer Guide - " +
"Learn about computer files that you come across in " +
"your daily work at: www.fileformat.com ");
document.write(out);
out.close();
Word 문서에 단락, 이미지 및 표 추가
Apache POI XWPF를 사용하면 개발자가 Word 문서에 단락 및 이미지를 추가할 수 있습니다. API는 또한 DOCX 문서에 표를 추가하는 기능을 제공하는 동시에 사용자 정의 데이터로 단순하고 중첩된 표를 만들 수 있습니다.
표로 새 DOCX 파일 만들기
// initialize a blank document
XWPFDocument document = new XWPFDocument();
// create a new file
FileOutputStream out = new FileOutputStream(new File("table.docx"));
// create a new table
XWPFTable table = document.createTable();
// create first row
XWPFTableRow tableRowOne = table.getRow(0);
tableRowOne.getCell(0).setText("Serial No");
tableRowOne.addNewTableCell().setText("Products");
tableRowOne.addNewTableCell().setText("Formats");
// create second row
XWPFTableRow tableRowTwo = table.createRow();
tableRowTwo.getCell(0).setText("1");
tableRowTwo.getCell(1).setText("Apache POI XWPF");
tableRowTwo.getCell(2).setText("DOCX, HTML, FO, TXT, PDF");
// create third row
XWPFTableRow tableRowThree = table.createRow();
tableRowThree.getCell(0).setText("2");
tableRowThree.getCell(1).setText("Apache POI HWPF");
tableRowThree.getCell(2).setText("DOC, HTML, FO, TXT");
document.write(out);
out.close();
Word OOXML 문서에서 텍스트 추출
Apache POI XWPF는 몇 줄의 코드로 Microsoft Word DOCX 문서에서 데이터를 추출하는 특수 클래스를 제공합니다. 같은 방식으로 Word 파일에서 머리글, 각주, 표 데이터 등을 추출할 수도 있습니다.
Word 파일에서 텍스트 추출
// load DOCX file
FileInputStream fis = new FileInputStream("document.docx");
// open file
XWPFDocument file = new XWPFDocument(OPCPackage.open(fis));
// read text
XWPFWordExtractor ext = new XWPFWordExtractor(file);
// display text
System.out.println(ext.getText());
DOCX 문서에 사용자 정의 머리글 및 바닥글 추가
머리글과 바닥글은 일반적으로 날짜, 페이지 번호, 작성자 이름 및 각주와 같은 추가 정보를 포함하므로 더 긴 문서를 구성하고 읽기 쉽게 유지하는 데 도움이 되는 추가 정보를 포함하므로 Word 문서의 중요한 부분입니다. Apache POI XWPF를 사용하면 Java 개발자가 Word 문서에 사용자 정의 머리글과 바닥글을 추가할 수 있습니다.
Word DOCX 파일에서 사용자 정의 머리글 및 바닥글 관리
public class HeaderFooterTable {
public static void main(String[] args) throws IOException {
try (XWPFDocument doc = new XWPFDocument()) {
// Create a header with a 1 row, 3 column table
XWPFHeader hdr = doc.createHeader(HeaderFooterType.DEFAULT);
XWPFTable tbl = hdr.createTable(1, 3);
// Set the padding around text in the cells to 1/10th of an inch
int pad = (int) (.1 * 1440);
tbl.setCellMargins(pad, pad, pad, pad);
// Set table width to 6.5 inches in 1440ths of a point
tbl.setWidth((int) (6.5 * 1440));
CTTbl ctTbl = tbl.getCTTbl();
CTTblPr ctTblPr = ctTbl.addNewTblPr();
CTTblLayoutType layoutType = ctTblPr.addNewTblLayout();
layoutType.setType(STTblLayoutType.FIXED);
BigInteger w = new BigInteger("3120");
CTTblGrid grid = ctTbl.addNewTblGrid();
for (int i = 0; i < 3; i++) {
CTTblGridCol gridCol = grid.addNewGridCol();
gridCol.setW(w);
}
// Add paragraphs to the cells
XWPFTableRow row = tbl.getRow(0);
XWPFTableCell cell = row.getCell(0);
XWPFParagraph p = cell.getParagraphArray(0);
XWPFRun r = p.createRun();
r.setText("header left cell");
cell = row.getCell(1);
p = cell.getParagraphArray(0);
r = p.createRun();
r.setText("header center cell");
cell = row.getCell(2);
p = cell.getParagraphArray(0);
r = p.createRun();
r.setText("header right cell");
// Create a footer with a Paragraph
XWPFFooter ftr = doc.createFooter(HeaderFooterType.DEFAULT);
p = ftr.createParagraph();
r = p.createRun();
r.setText("footer text");
try (OutputStream os = new FileOutputStream(new File("headertable.docx"))) {
doc.write(os);
}
}
}
}