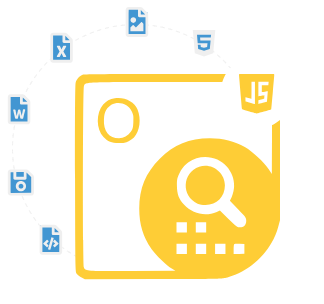
Aspose.OCR for JavaScript via a C++
Java OCR API to Optical Character Recognition
A Powerful JavaScript OCR API enables Software Developers to Add OCR Functionality to Web-based Project and Extract Text as well as Images from Documents online.
In today's fast-paced digital world, businesses and individuals alike are constantly seeking innovative solutions to streamline their operations. Optical Character Recognition (OCR) technology has become an invaluable tool in this quest, allowing for the automatic extraction of text from images and scanned documents. Aspose.OCR for JavaScript via C++ is a robust OCR solution, and while it is primarily designed for .NET applications, it is possible to integrate it with JavaScript via a C++ API. It can recognize text in multiple languages, making it suitable for global applications and supports various image formats, including JPEG, PNG, BMP, TIFF and many more.
Aspose.OCR is a powerful optical character recognition library that simplifies text extraction from images and documents. While it's primarily designed for .NET applications, software developers can use the JavaScript wrapper, to build their OCR application in JavaScript. This application can accept image files, call the C++ API for text extraction, and display or manipulate the recognized text as needed. The library supports several advanced features like can handle any image you can get form a scanner or camera, finds and automatically corrects misspelled words, Recognizing images provided as web links, multi-page PDF and TIFF files recognition, preserve formatting and so on.
Aspose.OCR for JavaScript via C++ offers high accuracy in text recognition, thanks to its advanced algorithms and machine learning capabilities. Integrating Aspose.OCR with JavaScript via a C++ API opens up new possibilities for utilizing OCR technology in web applications. Software developers can harness the power of the API to automate text extraction from images and scanned documents, ultimately improving efficiency and productivity in various industries. Its straightforward API and documentation make it accessible for developers with varying levels of experience.
Getting Started with Aspose.OCR for Java
The recommend way to install Aspose.OCR for JavaScript via C++ is using npm. Please use the following command for a smooth installation.
Install Aspose.OCR for JavaScript via C++ via npm
NuGet\Install-Package Aspose.Ocr.Cpp -Version 23.8.0
You can download the library directly from Aspose.OCR product page
Text Extraction from Images via JavaScript API
Aspose.OCR for JavaScript via C++ has included complete support for loading and extracting text from various types of images inside JavaScript applications. The API has included support some popular image file formats, such as JPEG, PNG, GIF, TIFF, PDF, BMP, and many more. There are several processing filters available that enables software developers to recognize rotated, skewed and noisy images. Moreover, the recognition results are returned in the most popular document and data exchange formats. The following example shows how JavaScript commands can be used to load and extract text from an image.
How to Perform Text Extraction from Images via JavaScript API?
const express = require('express');
const multer = require('multer'); // For handling file uploads
const child_process = require('child_process');
const app = express();
const port = 3000;
// Configure multer for handling file uploads
const storage = multer.memoryStorage();
const upload = multer({ storage: storage });
app.post('/process-image', upload.single('image'), (req, res) => {
// Save the uploaded image to a file (you might need additional processing here)
const imageBuffer = req.file.buffer;
const fs = require('fs');
fs.writeFileSync('input.jpg', imageBuffer);
// Execute the C++ backend
const child = child_process.spawn('./your_cpp_program', []);
// Capture the output from the C++ backend
let extractedText = '';
child.stdout.on('data', (data) => {
extractedText += data.toString();
});
// When the C++ process exits
child.on('close', (code) => {
if (code === 0) {
res.send({ text: extractedText });
} else {
res.status(500).send({ error: 'OCR processing failed' });
}
});
});
app.listen(port, () => {
console.log(`Server listening at http://localhost:${port}`);
});
Recognize Selected Areas of an Image via JS API
Aspose.OCR for JavaScript via C++ has included complete functionality that enables software developers to load and recognize a particular area inside an image using JavaScript API. The library can recognize the whole image or selected areas only; identifies words, lines or paragraphs. It supports detecting and recognizing all popular typefaces and font styles, including handwritten text with superior recognition speed and accuracy.
How to Recognize Selected Image Area using JavaScript API?
document.getElementById('process-button').addEventListener('click', () => {
const selectedArea = {
x: 100, // Define the selected area's coordinates (x, y, width, height)
y: 100,
width: 200,
height: 100,
};
const imageBlob = captureSelectedAreaAsBlob(selectedArea); // Implement this function to capture the selected area as an image blob
const formData = new FormData();
formData.append('image', imageBlob);
fetch('/api/ocr/recognize-selected-area', {
method: 'POST',
body: formData,
headers: {
'Accept': 'application/json',
},
})
.then(response => response.json())
.then(data => {
// Handle the recognized text response
console.log(data.text);
})
.catch(error => {
console.error(error);
});
});
Automatic Spell Checking Support in JS Apps
Aspose.OCR for JavaScript via C++ has included a very powerful support for spell checking and correction mechanism inside JavaScript applications. Sometimes non-standard fonts may cause certain characters or words to be recognized incorrectly. To further enhance the recognition process, the library has provided a powerful spell checker that enables software developers to search out and automatically corrects spelling errors. The library support various advanced features like automatic spelling correction, getting the list of misspelled words, working with custom dictionaries and so on.