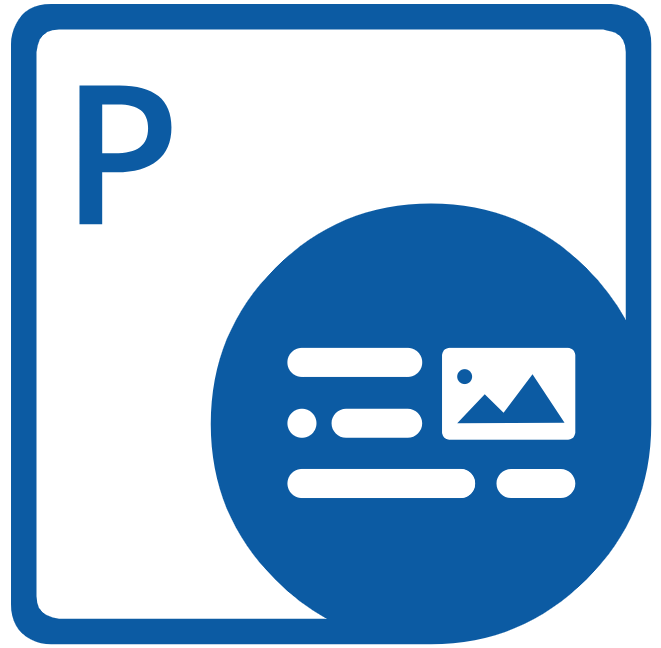
Aspose.PDF for C++
Create, Edit & Convert PDF Files via C++ API
Leading C++ API for Working with PDF Files, allows Software Professional to Generate, Modify, Merge/Split, Extract & Convert PDF Files.
What is Aspose.PDF for C++?
Aspose.PDF for C++ is a very powerful PDF document processing C++ library that enables software developers to generate and manipulate PDF documents without using Adobe Acrobat or any third-party applications. The library is very easy to handle and can easily implement rich capabilities for creating PDF files from scratch. The library can be used to build any type of 32-bit and 64-bit C++ application and can perform equally well on the server as well as the client-side.
Aspose.PDF for C++ has incorporated a wide range of features for creating, editing, parsing, manipulating, and converting PDF documents, such as Creating PDF forms and managing form fields, inserting or replacing images in PDFS, adding text to PDF, Custom font handling, Set page margin, manage page size, set PDF transition type, add & modify attachments and annotations, insert watermarks to PDF, add and manage bookmarks, split PDF documents, PDF merging, insert new pages, Transform pages to image, set metadata of PDF documents and many more.
Aspose.PDF for C++ also supports a wide range of security features, such as password protection and digital signatures, which can be used to protect sensitive information in a PDF document. The library also provides a wide range of options for optimizing and compressing PDFs, which can help to reduce their file size and make them more easily shareable. Additionally, the library fully supports a wide range of formatting options, such as font, color, and alignment, which allows developers to create professional-looking documents. Overall, Aspose.PDF for C++ is one of the best libraries for working with PDF documents in C++ applications.
Getting Started with Aspose.PDF for C++
The recommend way to install Aspose.PDF for C++ is using NuGet. Please use the following command for a smooth installation.
Install Aspose.PDF for C++ via NuGet
NuGet\Install-Package Aspose.PDF -prerelease
You can also download it directly from Aspose PDF product page.
PDF Files Generation & Editing via C++ API
Aspose.PDF for C++ has included complete support for PDF document creation, reading, and modifications inside C++ applications without the need of any other software installed on the user's machine. The library offers simple and intuitive methods for PDF creation, which makes it easy for developers to add and remove pages, add text, images, and other elements to a new document. The library has also provided various methods for opening existing PDF documents such as opening documents by specifying a file name, opening a document from the stream, opening an encrypted document, opening a document from a memory buffer, and more.
How to Create PDF Files via C++ API?
void CreatePDF() {
// String for path name.
String _dataDir("C:\\Samples\\");
// String for file name.
String filename("sample-new.pdf");
// Initialize document object
auto document = MakeObject();
// Add page
auto page = document->get_Pages()->Add();
// Add text to new page
auto textFragment = MakeObject(u"Hello World!");
page->get_Paragraphs()->Add(textFragment);
// Save updated PDF
String outputFileName = _dataDir + filename;
document->Save(outputFileName);
}
How to Secure PDF Files via C++ API?
Aspose.PDF for C++ has provided various important features for securing PDF documents inside C++ applications. It supports protect sensitive information inside PDF files by applying passwords as well as by using digital signatures. It is also possible to change the password of an existing PDF and also determine if the source PDF is password protected. The library also allows to encrypt and decrypt PDF documents with a just a couple of lines of code. You can set privileges like restricting users from changing the content of a document, extract image or text from the PDF file and only allows screen reading and so on.
How to Set Privileges of PDF File via C++ API?
void SecuringAndSigning::SetPrivilegesOnExistingPDF() {
// String for path name.
String _dataDir("C:\\Samples\\");
// Load a source PDF file
auto document = MakeObject(_dataDir + u"input.pdf");
// Instantiate Document Privileges object
// Apply restrictions on all privileges
auto documentPrivilege = DocumentPrivilege::get_ForbidAll();
// Only allow screen reading
documentPrivilege->set_AllowScreenReaders(true);
// Encrypt the file with User and Owner password.
// Need to set the password, so that once the user views the file with user password,
// Only screen reading option is enabled
document->Encrypt(u"user", u"owner", documentPrivilege, CryptoAlgorithm::AESx128, false);
// Save updated document
document->Save(_dataDir + u"SetPrivileges_out.pdf");
}
PDF Documents Conversion via C++ API
Aspose.PDF for C++ makes is easy for software developers to easily convert PDF documents to several other supported file formats inside their own C++ applications. The library has provided support for PDF files conversion to various popular formats, as well as conversion from other formats to PDF. You can convert PDF to Word documents, PowerPoint presentations, Microsoft Excel (XLSX, ODS, CSV and SpreadSheetML), EPUB, XPS, Postscript, text, PDF/A, and many other file formats. The library also has included strong support converting images to PDF as well as converting PDF pages as images in JPEG, PNG and other formats.
How to Convert PDF to XLS in C++ Apps?
void ConvertPDFtoExcel()
{
std::clog << __func__ << ": Start" << std::endl;
// String for path name
String _dataDir("C:\\Samples\\Conversion\\");
// String for file name
String infilename("sample.pdf");
String outfilename("PDFToExcel.xls");
// Open document
auto document = MakeObject(_dataDir + infilename);
try {
// Save the output in XLS format
document->Save(_dataDir + outfilename, SaveFormat::Excel);
}
catch (Exception ex) {
std::cerr << ex->get_Message();
}
std::clog << __func__ << ": Finish" << std::endl;
}
Optimize PDF Documents via C++ API
Aspose.PDF for C++ API has provided full support for PDF documents optimization inside C++ applications. The PDF optimization is mostly about reducing the size of PDFs to enhance their loading speed. The library is using various techniques for documents optimization such as page shrink or compresses all images, reusing page content, duplicate streams merging, un-embed fonts, removal of unused objects and form fields, flatten annotations removal and so on. Moreover, it also supports optimizing the content of your PDF document for better ranking in search engines.
How to Compress Images in PDF via C++ Library?
void CompressImage() {
// String for path name
String _dataDir("C:\\Samples\\");
// String for input file name
String infilename("ShrinkDocument.pdf");
String outfilename("ShrinkDocument_out.pdf");
// Open document
auto document = MakeObject(_dataDir + infilename);
// Initialize OptimizationOptions
auto optimizationOptions = MakeObject();
// Set CompressImages option
optimizationOptions->get_ImageCompressionOptions()->set_CompressImages(true);
// Set ImageQuality option
optimizationOptions->get_ImageCompressionOptions()->set_ImageQuality(50);
// Optimize PDF document using OptimizationOptions
document->OptimizeResources(optimizationOptions);
// Save updated document
document->Save(_dataDir + outfilename);
}