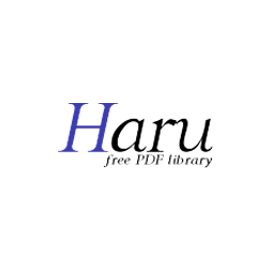
LibHaru
Open Source C++ Library for PDF Documents
Generate, Edit, Manipulate & Convert PDF Files via Open Source C++ API.
LibHaru is an open source C++ library that enables software developers to generate PDF file format, currently, the API doesn't allow reading or edited existing PDF documents. Using the API you can generate PDF file - add text, lines, and annotations int it. Furthermore, you can also add images of PNG and JPEG format in the document. LibHaru also allows compressing the PDF document with the deflate-decode format and generates encrypted PDF documents.
LibHaru is written ANSI-C and can work both as static-library and shared-library. To use it with a C++ program you can compile it with any C++ compiler and use it as a static-library.
Getting Started with LibHaru
LibHaru is written in ANSI-C and to use it with C++, you can compile it with any compliant C++ compiler. First of all, you can download and extract the latest version of the API. There are several kinds of makefile, for every compiler, in the script directory. Build the library with an appropriate makefile.
There are several kinds of makefile, for every compiler, in the script directory. Build the library with an appropriate makefile.
Build the Library for Compiler
//Microsoft VC++ Compiler
NMAKE -f script/Makefile.msvc
//Borland C++ Compiler
make -f script/Makefile.BCC
C++ Library to Generate PDF File Format
LibHaru has provided a set of features that enables software developers to generate PDF file format. Using the API you can create a new PDF document, set document object attributes, create a new page, set page object, set page description and save the document to a file ora memory stream.
Embed Images in PDF using C++
LibHaru enables software developers to embed JPEG and PNG images in PDF documents. Using the API you can get image size, width, height, bits per component and color space. Furthermore, you can set a color mask and mask image for the embedded image.
Create Encrypted PDF Files using C++ API
Encryption is a very useful mechanism that allows of encoding information into secret code that hides the information's true meaning. The Open source library LibHaru enables software developers to create encrypted PDF files without any external dependencies.
Create Encrypted PDF Files via C++
const static char* text = "This is an encrypt document example.";
const static char* owner_passwd = "owner";
const static char* user_passwd = "user";
jmp_buf env;
#ifdef HPDF_DLL
void __stdcall
#else
void
#endif
error_handler (HPDF_STATUS error_no,
HPDF_STATUS detail_no,
void *user_data)
{
printf ("ERROR: error_no=%04X, detail_no=%u\n", (HPDF_UINT)error_no,
(HPDF_UINT)detail_no);
longjmp(env, 1);
}
int
main (int argc, char **argv)
{
HPDF_Doc pdf;
HPDF_Font font;
HPDF_Page page;
char fname[256];
HPDF_REAL tw;
strcpy (fname, argv[0]);
strcat (fname, ".pdf");
pdf = HPDF_New (error_handler, NULL);
if (!pdf) {
printf ("error: cannot create PdfDoc object\n");
return 1;
}
if (setjmp(env)) {
HPDF_Free (pdf);
return 1;
}
/* create default-font */
font = HPDF_GetFont (pdf, "Helvetica", NULL);
/* add a new page object. */
page = HPDF_AddPage (pdf);
HPDF_Page_SetSize (page, HPDF_PAGE_SIZE_B5, HPDF_PAGE_LANDSCAPE);
HPDF_Page_BeginText (page);
HPDF_Page_SetFontAndSize (page, font, 20);
tw = HPDF_Page_TextWidth (page, text);
HPDF_Page_MoveTextPos (page, (HPDF_Page_GetWidth (page) - tw) / 2,
(HPDF_Page_GetHeight (page) - 20) / 2);
HPDF_Page_ShowText (page, text);
HPDF_Page_EndText (page);
HPDF_SetPassword (pdf, owner_passwd, user_passwd);
/* save the document to a file */
HPDF_SaveToFile (pdf, fname);
/* clean up */
HPDF_Free (pdf);
return 0;
}