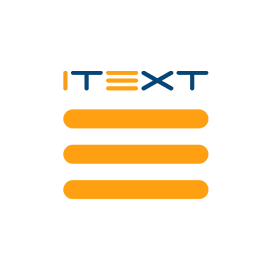
iText 7
Open Source Java API for PDF Documents
Java Library allows developers to Read, Write, Edit & Convert Text to PDF Files in Java apps.
What is iText 7?
iText 7 is an Open Source Java API that enables software developers to create their own PDF solution. It provides important features for integrating PDF functionality in Java applications or products. It helps you to create a smart document workflow. Document workflow is an important process in a company.
The Portable Document Format (PDF) is one of the World’s most used document formats and remains revolutionary. Data always play a key role in the success of an organization. iText 7 helps your organization by transforming your data into PDF documents. That can help you to save time as well as money.
Getting Started with iText 7
To use the iText’s libraries properly you will need two things. A valid license key file and the latest version of the license key library. You can easily download the license key library.
iText 7 is dual-licensed as AGPL/Commercial software. AGPL is a free/open-source software license. You can use the Central repository to download iText 7 Core.
iText 7 Core is available via Maven on The Central repository. You can choose whether you want to use one module or all modules. If you want to use all iText 7 modules you need to just add the following XML snippet in your pom.xml. Maven will do all the hard work for you and going to download the required modules from The Central Repository.
You need to have a license key library (itext-licensekey-x.y.z.jar) on the classpath.
Here is the command
mvn clean instal
It will compile the Java sources and package the binary classes into jar packages by default.
Java API to Create & Manipulate PDF Documents
iText 7 enables software developers to create as well as manipulate a PDF document inside Java applications. The PDF file format is one of the most popular file formats used nowadays. Moreover, you can easily modify the existing PDF documents. The library also gives developers the capability to insert new pages as well as add new content, to an existing PDF page with ease.
How to Manipulate PDF document via Java API?
// Initialize document
PdfDocument pdfDoc = new PdfDocument(new PdfReader("input.pdf"),
new PdfWriter("output.pdf"));
// Add annotation in it
PdfAnnotation ann = new PdfTextAnnotation(new Rectangle(400, 795, 0, 0))
.setTitle(new PdfString("FileFormat"))
.setContents("Developer Guide for fileformats");
pdfDoc.getFirstPage().addAnnotation(ann);
// Close document
pdfDoc.close();
Convert Text to PDF Documents
PDF Java API enables Java programmers to easily convert text to PDF documents inside their own Java applications. It’s very simple to export plain text file to a PDF document. It gives you the ability to define the alignment at the level of the document. Moreover, the latest release also supports several layout features that make it easier to read as compared to the older versions.
How to Convert Text to PDF via Java Library?
// Initialize output document
PdfDocument pdf = new PdfDocument(new PdfWriter("TextToPDF.pdf"));
Document document = new Document(pdf);
// Open txt document
BufferedReader br = new BufferedReader(new FileReader("input.txt"));
String line;
// Add each line
while ((line = br.readLine()) != null) {
document.add(new Paragraph(line));
}
// Save document
document.close();
Secure PDF Files by Adding Password to It via Java API/h2>
It is always very important for any organization to protect and secure their important data as well as their documents. The iText 7 Java library has provided numerous techniques for the security of use’s documents, such as share it with authorized users or editors by applying passwords to it, use digital signatures and so on. The following Java code example shows how to add a password to an existing PDF document.
How to Add Password to Secure PDF Files via Java Code?
PdfReader reader = new PdfReader(src);
WriterProperties props = new WriterProperties()
.setStandardEncryption(USERPASS, OWNERPASS, EncryptionConstants.ALLOW_PRINTING,
EncryptionConstants.ENCRYPTION_AES_128 | EncryptionConstants.DO_NOT_ENCRYPT_METADATA);
PdfWriter writer = new PdfWriter(new FileOutputStream(dest), props);
PdfDocument pdfDoc = new PdfDocument(reader, writer);
pdfDoc.close();