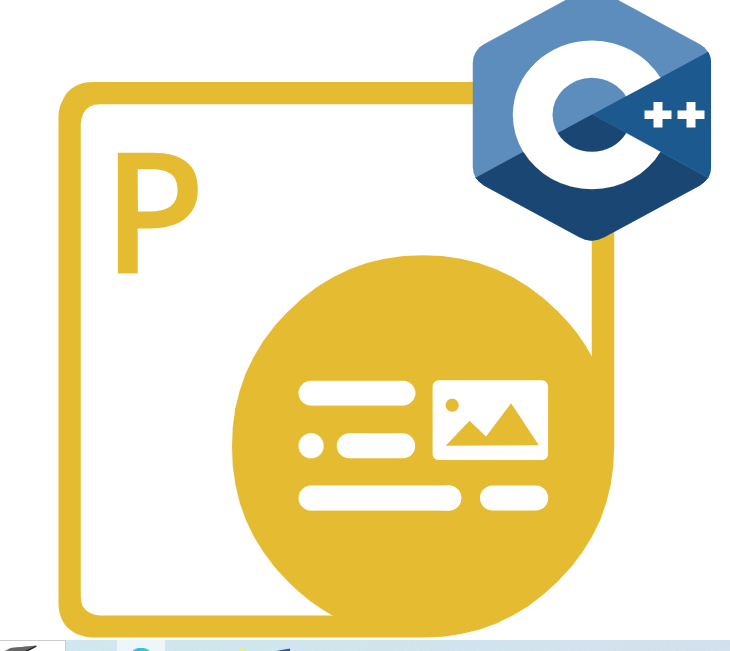
Aspose.PDF for JavaScript via C++
JavaScript API for PDFs Creation & Conversion
PDF Document Generation, Editing, Rendering & Conversion to other supported file formats without using Adobe Acrobat via JavaScript Library.
Aspose.PDF for JavaScript via C++ is an advanced PDF processing and conversion library that helps software developers to build web applications with the ability to create, modify, and convert PDF documents with ease. It is a unique offering that enables software developers to leverage the power of JavaScript for PDF manipulation inside their own applications. Aspose.PDF for JavaScript via C++ is designed for high performance, with optimized algorithms that ensure fast and efficient PDF processing.
Aspose.PDF for JavaScript via C++ can be easily integrated into existing JavaScript applications, with simple and intuitive APIs that require minimal coding effort. There are several important features part of the library for working with PDF documents, such as optimizing PDF documents, adding a stamp to a PDF file, adding an image to PDF file, extracting text from PDFs, splitting PDF into two files, converting PDF to Images format, Encrypt & Decrypt PDF files, add hyperlinks to PDF, add attachments to a PDF, Unicode support and many more.
Aspose.PDF for JavaScript via C++ can be used to extract data from PDF documents for analysis and processing in industries such as healthcare, finance, and research. Software developers can secure their PDF documents by adding password protection, encryption, and digital signatures to prevent unauthorized access and protect sensitive information. With its advanced features, cross-platform support, efficient performance, easy integration, and comprehensive documentation and support, Aspose.PDF for JavaScript via C++ is a reliable and efficient solution for your PDF manipulation needs.s
Getting Started with Aspose.PDF for Java
To install and use Aspose.PDF for JavaScript via C++ from a -*ZIP archive, follow the following instructions
- extract the files from the ZIP archive
- encrypt your *.lic file using ‘encrypt_lic.html’
- edit ‘settings.json’ and setup your settings
Follow the link for Direct Download
You can also download the library directly from Aspose.PDF product page
Convert PDF Files via JavaScript API
Portable Document Format (PDF) is a very popular among the internet community and due to its portability, mobility and readability it is considered to be a safe, convenient way to distribute private information through the web. Aspose.PDF for JavaScript via C++ is a very useful tool that helps software developers to convert their PDF documents to other support file formats such as JPEG, PDF and more. With just a couple of steps you can convert PDF files to JPEG file formats as given below.
Convert PDF to JPEG using JavaScript API
var ffileToJpg = function (e) {
const file_reader = new FileReader();
file_reader.onload = (event) => {
//convert a PDF file to jpg-files with template "ResultPdfToJpg{0:D2}.jpg" ({0}, {0:D2}, {0:D3}, ... format page number) and save
const json = AsposePdfPagesToJpg(event.target.result, e.target.files[0].name, "ResultPdfToJpg{0:D2}.jpg");
if (json.errorCode == 0) {
document.getElementById('output').textContent = "Files(pages) count: " + json.filesCount.toString();
//make links to result files
for (let fileIndex = 0; fileIndex < json.filesCount; fileIndex++) (json.filesNameResult[fileIndex], "mime/type");
}
else document.getElementById('output').textContent = json.errorText;
};
file_reader.readAsArrayBuffer(e.target.files[0]);
};
Optimize PDF Document via JavaScript API
Aspose.PDF for JavaScript via C++ gives software developers the power to optimize PDF documents content for the Web using JavaScript code. PDF Optimization, or linearization for Web, is a very useful process that helps users in making PDF documents suitable for online browsing using a web browser. Usually it can considerably compress PDF size, boost display speed and make it more appropriate for the user. The following JavaScript example demonstrates how to optimize PDF document suitable for web with just a couple of lines of JavaScript code.
How to Optimize a PDF Document using JavaScript API?
var ffileOptimize = function (e) {
const file_reader = new FileReader();
file_reader.onload = (event) => {
/*optimize a PDF-file and save the "ResultOptimize.pdf"*/
const json = AsposePdfOptimize(event.target.result, e.target.files[0].name, "ResultOptimize.pdf");
if (json.errorCode == 0) document.getElementById('output').textContent = json.fileNameResult;
else document.getElementById('output').textContent = json.errorText;
/*make a link to download the result file*/
DownloadFile(json.fileNameResult, "application/pdf");
};
file_reader.readAsArrayBuffer(e.target.files[0]);
};
Combine PDF Files into Single PDF via JavaScript API
Aspose.PDF for JavaScript via C++ has included very powerful features for PDF manipulation inside Web applications. Software Developers can easily combine multiple PDF documents or Split a large PDF file into multiple PDF documents using JavaScript commands. It is very a very useful process that helps users to combine multiple files into a single one to manage or print it easily. The following JavaScript code example shows how computer programmers can concatenate multiple PDF files with ease.
How to how to Concatenate PDF Files using JavaScript?
var ffileMerge = function (e) {
const file_reader = new FileReader();
function readFile(index) {
/*only two files*/
if (index >= e.target.files.length || index >= 2) {
/*merge two PDF-files and save the "ResultMerge.pdf"*/
const json = AsposePdfMerge2Files(undefined, undefined, e.target.files[0].name, e.target.files[1].name, "ResultMerge.pdf");
if (json.errorCode == 0) document.getElementById('output').textContent = json.fileNameResult;
else document.getElementById('output').textContent = json.errorText;
/*make a link to download the result file*/
DownloadFile(json.fileNameResult, "application/pdf");
return;
}
const file = e.target.files[index];
file_reader.onload = function (event) {
/*prepare(save) file from BLOB*/
AsposePdfPrepare(event.target.result, file.name);
readFile(index + 1)
}
file_reader.readAsArrayBuffer(file);
}
readFile(0);
}
Extract Text from All Pages of PDF via JavaScript
Aspose.PDF for JavaScript via C++ gives software developers the capability to extract text from PDF documents using JavaScript API. It is a very useful process allowing programmers to get a particular part of a PDF documents and use it in a place where they need it. The library makes it easy for software developers to extract text from PDF images or scanned PDFs without any external dependencies. The following example shows how to get PDF text with just a couple of lines of JavaScript code.
How to Extract Text from PDF File via JavaScript?
var ffileExtract = function (e) {
const file_reader = new FileReader();
file_reader.onload = (event) => {
//Extarct text from a PDF-file
const json = AsposePdfExtractText(event.target.result, e.target.files[0].name);
if (json.errorCode == 0) document.getElementById('output').textContent = json.extractText;
else document.getElementById('output').textContent = json.errorText;
};
file_reader.readAsArrayBuffer(e.target.files[0]);
};