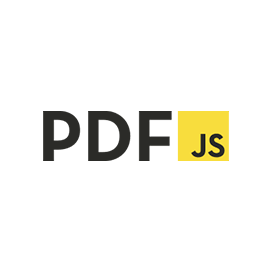
PDFjs
Open Source JavaScript Library for PDF Files
Add Annotations, Image & Text to PDFs via Open Source Free JavaScript Library.
What is PDFjs?
PDFjs is an open source Portable Document Format (PDF) generation library that can best suites server- and client-side applications development. PDF is popular across the world and several companies are using it to create & share documents or reports across the globe. With the help of the PDFjs library, you just require a couple of commands to access and reuse PDF documents inside your own applications.
The library has incorporated support for several noticeable features, such as PDF documents creation, drawing shapes to PDFs, header and footer support, adding tables to PDF, AFM fonts & OTF font embedding, images insertion to PDF pages, PDF merging, adding pages to PDF, inserting & displaying text, exporting PDF to other file formats and many more.
Getting Started with PDFjs
The recommend and easiest way to install the PDFjs library is using npm, please use the following command to achieve it.
Install PDFjs using npm
npm install pdfjs
PDF Documents Creation via Free JavaScript API
PDF documents are always very useful for companies and individuals to save and share information with each other. PDFjs library makes it easy for you to generate and modify PDF documents inside your application with just a couple of simple commands. The library also facilitates developers to add new pages, insert images, define document orientation, and much more.
How to Create PDF Documents via JavaScript Library?
const pdf = require('.lib')
// Add Text Annotations
module.exports = function(doc, { lorem, font }) {
doc.text('goto B', { goTo: 'B' })
doc.text('goto A', { goTo: 'A' })
}
Create PDF Annotations via Free JavaScript API
The open source PDFjs library supports creating PDF documents with Annotations. Annotations let developers add custom content inside PDF documents. There are various kinds of annotations that can be used in PDF documents, such as text, lines, notes or shapes, etc. PDFjs library fully supports and makes it easy for developers to create various types of PDF annotations inside their own applications. The following simple lines of code can add text annotations in PDF documents in JavaScript.
- Include PDFjs Library
- Add Text Annotations
- Export PDF document
How to Add Text Annotations in PDF - JavaScript?
const pdf = require('.lib')
// Add Text Annotations
module.exports = function(doc, { lorem, font }) {
doc.text('goto B', { goTo: 'B' })
doc.text('goto A', { goTo: 'A' })
}
Merging PDF Documents
The PDFjs library allows software developers to programmatically combine numerous PDF documents into one document inside their apps. The library enables programmers to generate a new PDF document from the existing one, add one specific page of an external PDF, implement kerning, add whole pages of other PDFs, and more. The library also gives users the ability to create custom PDF reports.
Adding Graphics to PDF Documents
Graphics and images are always very useful for sharing better information and adding more value to a piece of content. The PDFjs library facilitates JavaScript professionals to insert graphics of their choice inside their JavaScript applications. You can use images types of images like JPEG or PNG inside a PDF file. You can also draw an ellipse, triangle, circle, etc.
How to Add JPEG to PDF via JavaScript Library?
// Adding JPEG image to PDF via PDFjs
module.exports = function(doc, {image, lorem}) {
doc.image(image.jpeg, {
width: 64, align: 'center', wrap: false, x: 10, y: 30
})
doc.text(lorem.shorter)
doc.image(image.jpeg)
doc.image(image.jpeg, {
width: 128, align: 'left'
})
doc.image(image.jpeg, {
height: 55, align: 'center'
})
doc.image(image.jpeg, {
width: 128, align: 'right'
})
doc.text(lorem.shorter)
}
Manage Headers & Footers in PDF Documents via JavaScript
The PDFjs library facilitates JavaScript developers to manage header & footer inside their PDF Documents with just a couple of lines of code. It has provided several important features for handling PDF headers & footers such as adding page numbers, adjusting font, applying font color, adjusting line height, applying text alignment, and more. The following code example shows how to add a header & footer inside a PDF file.
How to Add Headers & Footers to PDF via JavaScript Library?
module.exports = function(doc, {lorem, image}) {
// header
const header = doc.header()
header.text('text')
let cell = header.cell({ padding: 20, backgroundColor: 0xdddddd })
cell.text('TESTING')
cell.image(image.pdf)
// footer
const footer = doc.footer()
footer.text('text')
cell = footer.cell({ padding: 20, backgroundColor: 0xdddddd })
cell.image(image.complexPdf)
cell.text('TESTING')
// body
doc.text('Hello')
doc.pageBreak()
doc.text(lorem.long, { fontSize: 20 })
}