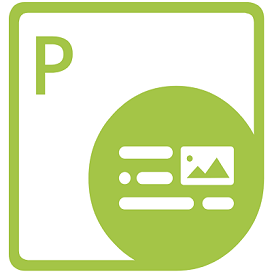
Aspose.PDF for .NET
C# .NET API for PDF Files Creation & Conversion
A Powerful PDF C# .NET API to Generate, Edit, Render, Manipulate, & Convert PDF Files to Word, Excel, PowerPoint & Images Formats without using Adobe Acrobat.
Aspose.PDF for .NET library can be very handy for developers who are interested to create and manipulate PDF documents inside their own applications with just a couple of lines of C# .NET code. The library is designed to be easy to use and provides a wide range of features that make it suitable for a variety of use cases. With its rich set of features and easy-to-use APIs, Aspose.PDF is a valuable tool for any developer working with PDF documents in a .NET environment (WinForms, WPF, ASP.NET, and .NET Compact Framework).
Aspose.PDF for .NET is a highly flexible and powerful library that is well-suited for a wide range of use cases. Whether you are inserted in creating new PDF documents, manipulating existing ones, or converting PDFs to other formats, this library provides everything you need to get the job done. The library can be used to convert text, images, SVG, HTML to PDF as well as export PDF to numerous document formats with excellent performance and good quality.
The library has included support for a wide variety of functions for handling PDF documents, such as creating PDFs from the scratch, compressing PDF files, table creation, and manipulation, using graph objects in PDFs, custom font handling, security controls support, insert or remove bookmarks, use PDF table of contents, add or delete attachments, manage PDF annotations, insert text and images, extract or inset pages in PDFs, merging multiple PDFs into a single document, splitting a PDF into multiple pages, pages to image conversion, print PDF documents and so on.
Getting Started with Aspose.PDF for .NET
The recommend way to install Aspose.PDF for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Pdf via NuGet Command
Install-Package Aspose.Pdf
You can download the library directly from Aspose.PDF product page
Generate PDF Documents via .NET API
One of the key features of Aspose.PDF for .NET is its ability to create PDF documents from scratch with just a couple of lines of C# code. Software developers can use the library to add text, images, forms, annotations, new pages, attachments, bookmarks, and other elements to a PDF document, as well as control the layout and formatting of the content. Additionally, the library provides a rich set of APIs for manipulating existing PDF documents, such as merging multiple PDFs into a single document, splitting a PDF into multiple pages, and extracting text and images from a PDF.
How to Create PDF using C#?
// Initialize document object
Document pdf_doc = new Document();
// Add page
Page page = pdf_doc.Pages.Add();
// Place the text of choice
page.Paragraphs.Add(new Aspose.Pdf.Text.TextFragment("Text of choice"));
// PDF file created at a specified location
pdf_doc.Save("created_one.pdf");
Convert PDF to Other File Formats via C# API
Converting PDF documents to other file formats is one of the most popular and important tasks for many software developers. Aspose.PDF for .NET makes their job easy by providing complete functionality for programmatically converting PDF documents to other supported file formats with just a few lines of .NET code. The library supports a wide range of output formats, including Microsoft Word, Excel, PowerPoint, images, HTML, and many more. It is also possible to save a PDF document to file, stream, send to Web or save as PDF/A document. The library makes it easy for software developers to work with PDF documents in other applications and platforms.
Convert PDF to Word via C#.NET API
public static void ConvertPDFtoDOCX()
{
// load PDF with an instance of Document
var document = new Document("template.pdf");
// save document in DOC format
document.Save("output.doc", Aspose.Pdf.SaveFormat.DocX);
}
Merge or Split PDF Documents via C# .NET
Aspose.PDF for .NET library enables software developers to merge multiple PDF files into a single PDF document or split large PDF files into smaller ones inside their own .NET applications. The library has provided various functions for merging and splitting PDF files, such as adding one document to the end of another PDF file, splitting PDF pages into individual PDF files, splitting the range of PDF pages into individual PDF file, and so on.
Split PDF into Multiple Files via C# API
// The path to the documents directory.
string dataDir = RunExamples.GetDataDir_AsposePdf_Pages();
// Open document
Document pdfDocument = new Document(dataDir + "SplitToPages.pdf");
int pageCount = 1;
// Loop through all the pages
foreach (Page pdfPage in pdfDocument.Pages)
{
Document newDocument = new Document();
newDocument.Pages.Add(pdfPage);
newDocument.Save(dataDir + "page_" + pageCount + "_out" + ".pdf");
pageCount++;
}
Images Conversion to PDF via .NET API
Aspose.PDF for .NET library has provided complete support for various images conversion to PDF using C# commands. Software developers can convert some of the most popular image formats, such as - BMP, CGM, DICOM, EMF, JPG, PNG, SVG, and TIFF formats with ease. To convert an image first you need to Initialize a new document class object and load the image. After that just call save and convert to image format and save it in the PDF file format. In some cases, the library also supports setting the height, width, and margins of a page as well as the image.
convert JPG Image to PDF via .NET API
// Load input JPG file
String path = dataDir + "Aspose.jpg";
// Initialize new PDF document
Document doc = new Document();
// Add empty page in empty document
Page page = doc.Pages.Add();
Aspose.Pdf.Image image = new Aspose.Pdf.Image();
image.File = (path);
// Add image on a page
page.Paragraphs.Add(image);
// Save output PDF file
doc.Save(dataDir + "ImagetoPDF.pdf");