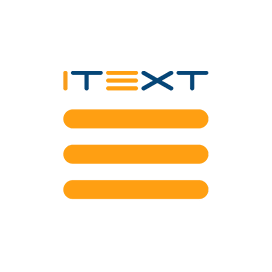
iText for .NET
Open Source .NET Library for PDF Document Processing
Read, Write, Manipulate, Merge and Convert HTML to PDF files from .NET apps via Open Source C# .NET APIs.
What is iText for .NET Library?
iText for .NET is an open source .NET library that gives developers the capability to create PDF solution of their own choice. It was previously known as iTextSharp and is capable of creating a smart PDF document workflow.
iText for .NET allows creating PDF documents programmatically without human intervention while supporting several important features such as PDF creation from scratch, HTML to PDF export, PDF redaction, multi-language support, PDF manipulation, XFDF & SVG handling, PDF data extraction, PDF tagging & parsing and more.
Getting Started with iText for .NET
iText for .NET is dual-licensed as AGPL/Commercial software. AGPL is a free / open-source software license. It is highly recommend using NuGet to add iText 7 Community to your project.
NuGet command
Install Package itext7
.NET API to Create & Manipulate PDF Documents
iText for .NET allows software programmers to create as well as modify a PDF document inside their .NET applications. The Portable Document Format (PDF) is one of the World’s most used document formats and still very popular. Once the PDF document is created, you can also modify it with ease. The API gives you’re the power to insert new pages, add new content, to an existing page and much more.
How to Create PDF Document via C# API?
// Initialize PDF writer
PdfWriter pdfWriter = new PdfWriter("fileformat.pdf");
// Creatre a new PDF document
PdfDocument pdfDocument = new PdfDocument(pdfWriter);
// Create a new document
Document document = new Document(pdfDocument);
// Add text to the document
document.Add(new Paragraph("FileFormat.com - File Format Developer Guide"));
// Close document
document.Close();
Convert HTML to PDF Documents using .NET
iText 7 allows .NET programmers to easily convert HTML to PDF document by using Html2Pdf add on. Html2Pdf add-on enable software developers to parse HTML or XHTML snippets and the related CSS to PDF. With just few lines of code and lesser time Html2Pdf can provide you great results and will convert HTML files into rich, smart PDF documents.
How to Convert HTML to PDF using C# .NET?
// Open text file
FileStream htmlSource = File.Open("fileformat.html", FileMode.Open);
// Create PDF file
FileStream pdfDest = File.Open("fileformat.pdf", FileMode.OpenOrCreate);
// Intialize conversion properties
ConverterProperties converterProperties = new ConverterProperties();
// Convert HTML to PDF
HtmlConverter.ConvertToPdf(htmlSource, pdfDest, converterProperties);
Merging Multiple PDF Files via .NET API
The open source library iText for .NET allows software programmers to merge multiple PDF files inside their.NET apps. It is very important to maintain the size and orientation of all the pages within the original files. The following C# code example shows how easily can developers combine different PDF files with just a couple of lines of code.
How to Merge Multiple PDF Files via C# .NET API?
PdfDocument pdf = new PdfDocument(new PdfWriter(dest));
PdfMerger merger = new PdfMerger(pdf);
//Add pages from the first document
PdfDocument firstSourcePdf = new PdfDocument(new PdfReader(SRC1));
merger.merge(firstSourcePdf, 1, firstSourcePdf.getNumberOfPages());
//Add pages from the second pdf document
PdfDocument secondSourcePdf = new PdfDocument(new PdfReader(SRC2));
merger.merge(secondSourcePdf, 1, secondSourcePdf.getNumberOfPages());
firstSourcePdf.close();
secondSourcePdf.close();
pdf.close();