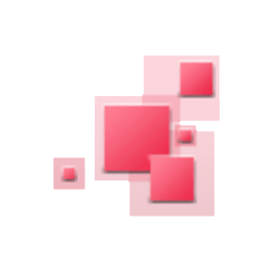
PDFsharp
Open Source .NET API for PDF Processing
Create, Manipulate, Convert & Process PDF Documents via Free C# .NET Library. Combine Multiple PDF Files, Add PDF Annotations with a Couple of Lines of C# Code.
What is PDFsharp?
Open Source .NET library that can be used to create, render, merge, split, modify, print and extract text or meta-data of PDF files. The PDFsharp API supports creating PDF documents on the fly from any .NET language. It also supports importing data from various sources via XML files or direct interfaces. It supports numerous options for page layout, text formatting, and document design.
PDFsharp provides graphical implementation based either on GDI+ or WPF. The API makes developer’s job easy by providing features for using one source code for drawing on a PDF page as well as in a window or on the printer. It supports several important features for processing PDF files such as modifying PDF, merge or split PDFs, XPS to PDF conversion, PDF rendering, PDF data extraction, Font embedding and subsetting, Unicode support and many more.
Getting Started with PDFsharp
PDFsharp is dual-licensed as AGPL/Commercial software. AGPL is a free/open-source software license.
It is highly recommended using NuGet to add PDFsharp to your project,
NuGet command
Install-Package PdfSharp
With Visual Studio you can install the NuGet Package Manager to easily access NuGet packages. It works with VS 2012 Express as well as with the community editions of VS 2013 and VS 2015. In Visual Studio go to "Tools" => "Extensions and Updates..." to install the NuGet Package Manager if you do not have it yet. The NuGet Package Manager will download the package for you, install it, and add a reference to your project.
Generate & Modify PDF Documents via Free .NET API
Software developers can use PDFsharp API to create a new PDF document inside their own .NET applications. Once the document is created you can add an empty page as well as insert graphics or text with ease. It also facilitates developers to modify the existing document according to their needs and save it with the name of their choice. Using the following steps you can generate & manipulate PDF documents in C#.
- Initialize PdfDocument
- Add Page
- Get an XGraphics object for drawing
- Create a font
- Add Text
- Save Document
How to Create PDF using C# API?
// Create a new PDF document
PdfDocument pdfDocument = new PdfDocument();
// Create an empty page
PdfPage pdfPage = pdfDocument.AddPage();
// Get an XGraphics object for drawing
XGraphics xGraphics = XGraphics.FromPdfPage(pdfPage);
// Create a font
XFont xFont = new XFont("Verdana", 20, XFontStyle.BoldItalic);
// Draw the text
xGraphics.DrawString("File Format Developer Guide", xFont, XBrushes.Black,
new XRect(0, 0, pdfPage.Width, pdfPage.Height),
XStringFormats.Center);
// Save the document...
pdfDocument.Save("fileformat.pdf");
Create PDF Annotations via .NET API
Annotations allow users to add custom content on PDF pages. PDF applications normally allow the creation & modification of various types of annotations, such as text, lines, notes or shapes, etc. PDFsharp enables programmers to create various types of PDF annotations inside their own applications. The library supports the creation of text annotations, links, and rubber stamp annotations.
How to Create a PDF Text Annotation via .NET Library?
// Create a PDF text annotation
PdfTextAnnotation textAnnot = new PdfTextAnnotation();
textAnnot.Title = "This is the title";
textAnnot.Subject = "This is the subject";
textAnnot.Contents = "This is the contents of the annotation.\rThis is the 2nd line.";
textAnnot.Icon = PdfTextAnnotationIcon.Note;
gfx.DrawString("The first text annotation", font, XBrushes.Black, 30, 50, XStringFormats.Default);
// Convert rectangle from world space to page space. This is necessary because the annotation is
// placed relative to the bottom left corner of the page with units measured in point.
XRect rect = gfx.Transformer.WorldToDefaultPage(new XRect(new XPoint(30, 60), new XSize(30, 30)));
textAnnot.Rectangle = new PdfRectangle(rect);
// Add the annotation to the page
page.Annotations.Add(textAnnot);
Combine Multiple PDF Documents via .NET
Do you have numerous PDF documents that need to be combined into one big document? PDFsharp API provides you the functionality for combining multiple PDF files into a single one with just a few lines of code. Developers can easily create a new document from existing PDF files. This may be useful for visual comparison or several other important tasks.
How to Combine PDF Documents via .NET API?
// Open the input files
PdfDocument inputDocument1 = PdfReader.Open(filename1, PdfDocumentOpenMode.Import);
PdfDocument inputDocument2 = PdfReader.Open(filename2, PdfDocumentOpenMode.Import);
// Create the output document
PdfDocument outputDocument = new PdfDocument();
// Show consecutive pages facing. Requires Acrobat 5 or higher.
outputDocument.PageLayout = PdfPageLayout.TwoColumnLeft;
XFont font = new XFont("Verdana", 10, XFontStyle.Bold);
XStringFormat format = new XStringFormat();
format.Alignment = XStringAlignment.Center;
format.LineAlignment = XLineAlignment.Far;
XGraphics gfx;
XRect box;
int count = Math.Max(inputDocument1.PageCount, inputDocument2.PageCount);
for (int idx = 0; idx < count; idx++)
{
// Get page from 1st document
PdfPage page1 = inputDocument1.PageCount > idx ?
inputDocument1.Pages[idx] : new PdfPage();
// Get page from 2nd document
PdfPage page2 = inputDocument2.PageCount > idx ?
inputDocument2.Pages[idx] : new PdfPage();
// Add both pages to the output document
page1 = outputDocument.AddPage(page1);
page2 = outputDocument.AddPage(page2);
// Write document file name and page number on each page
gfx = XGraphics.FromPdfPage(page1);
box = page1.MediaBox.ToXRect();
box.Inflate(0, -10);
gfx.DrawString(String.Format("{0} • {1}", filename1, idx + 1),
font, XBrushes.Red, box, format);
gfx = XGraphics.FromPdfPage(page2);
box = page2.MediaBox.ToXRect();
box.Inflate(0, -10);
gfx.DrawString(String.Format("{0} • {1}", filename2, idx + 1),
font, XBrushes.Red, box, format);
}
// Save the document...
const string filename = "CompareDocument1_tempfile.pdf";
outputDocument.Save(filename);