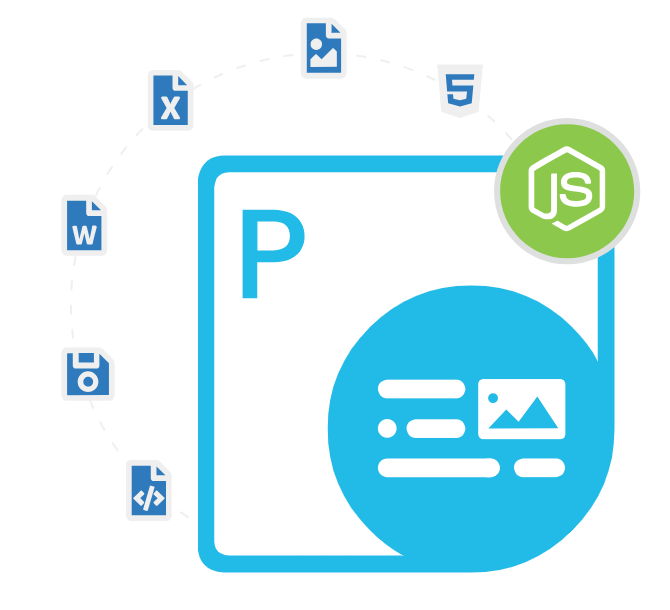
Aspose.PDF Cloud Node.js SDK
Node.js REST API for PDF File Creation & Conversion
Leading Node.js Library for Working with PDF Documents in Cloud. It enables Developers to create, Read, Edit, Merge/Split, Render and Convert PDF Files inside Node.js Apps.
What is Aspose.PDF Cloud Node.js SDK?
PDF processing is a fundamental aspect of many software applications, whether for generating reports, converting file formats, or securing documents. However, handling PDFs can be challenging due to the complexity of the format. This is where Aspose.PDF Cloud Node.js SDK simplifies these tasks, empowering software developers to build robust cloud-based PDF applications without needing extensive knowledge of PDF structures. It is a powerful wrapper for the Aspose.PDF Cloud API, designed to perform a wide range of PDF operations from a Node.js environment. It connects to the cloud-based PDF service provided by Aspose, allowing developers to process PDFs without installing or managing any additional software.
Aspose.PDF Cloud Node.js SDK provides a powerful toolkit for software developers to work with PDFs in cloud-based environments using Node.js. The SDK allows for a wide range of PDF manipulations including creation, editing, encryption, conversion, and annotations through REST API calls. It can be integrated into any platform that supports REST APIs. The Node.js SDK is just one of many supported environments, allowing for easy integration into web apps, mobile applications, or even server-less environments. With just a couple of lines of code software developers can create new PDF document from the scratch, convert PDF to other format, extract text from PDF file, encrypt or decrypt PDF files, merging or splitting PDFs, creating annotation in PDF documents and so on.
One of the major benefits of Aspose.PDF Cloud Node.js SDK is its ability to scale with your application’s growth. Since all PDF processing is done in the cloud, you don’t need to worry about local resource constraints. This allows developers to build applications that can handle thousands of requests without performance bottlenecks. As hosted in the cloud, software developers don't need to maintain servers or worry about software updates. Developers looking to create cloud-based PDF apps can benefit greatly from the SDK. It takes care of the complex PDF processing tasks in the cloud, allowing developers to concentrate on creating scalable apps with advanced features, rather than dealing with infrastructure issues.
Getting Started with Aspose.PDF Cloud Node.js SDK
Before we dive into coding, you'll need to install the SDK and set up the environment. You can easily install the Aspose.PDF Cloud Node.js SDK using NPM, please use the following command for a smooth installation.
Install Aspose.PDF Cloud Node.js SDK via NPM
npm install asposepdfcloud
You can also download the library directly from Aspose.PDF product page
Creating a PDF Document via REST API
Aspose.PDF Cloud Node.js SDK enables software developers to create cloud-based applications for working with PDF documents. One of the simplest tasks these cloud-based PDF applications can do is to create a new PDF document dynamically with ease. Developers can define various parameters such as add new page, define page size, insert new image, text content, and other elements. The following example shows how to create new PDF document and add new page or text to it inside Node.js applications.
How to Creation new PDF Documents inside Node.js?
const pdf = require('aspose-pdf-cloud');
// Create a new PDF file
const pdfDoc = new pdf.Document();
// Add a page to the PDF file
const page = pdfDoc.addPage();
// Add some text to the page
page.paragraphs.push(new pdf.Paragraph('Hello, World!'));
// Save the PDF file to a file
pdfDoc.save('output.pdf');
Convert PDF to Various Formats in Node.js
Cloud-based PDF applications often need to convert PDFs into other formats for different use cases like editing, viewing, merging, sharing and so on. Aspose.PDF Cloud Node.js SDK supports conversion between PDF and various other file formats such as Word, Excel, PowerPoint, XPS, HTML, XML, and images. This feature is particularly useful when you need to export PDF content to other file types for editing or sharing. Here is an example that shows, how software developers can convert a PDF file to JPEG file format inside Node.js applications.
How to Convert PDF File to JPEG File Formats inside Node.js Apps?
const { PdfApi } = require("asposepdfcloud");
const { PdfAType } = require("asposepdfcloud/src/models/movieAnnotation");
pdfApi = new PdfApi("XXXX", "XXXXXXX")
console.log('running example');
PdfApi.getPageConvertToJpeg("4pages.pdf", 1, null, null, null)
.then((result) => {
console.log(result.response);
});
Extract Content from PDF via Node.js API
Extracting data from a PDF is useful for building applications that require content analysis, searching, or text mining. With Aspose.PDF Cloud Node.js SDK, software developers can extract text or images from specific pages or the entire document with just a couple of lines of JavaScript code. This is essential for applications that require data analysis or content reusability. Here is an example that shows how users can extract text from a PDF document inside Node.js applications.
How to Extract from PDF Document inside Node.js Environment?
async function extractTextFromPdf() {
const response = await pdfApi.getTextExtract("sample.pdf", 1);
console.log("Extracted text from PDF:", response);
}
extractTextFromPdf();
Merge Multiple PDFs inside Node.js Apps
In business workflows, merging multiple PDFs into a single file is a common requirement. The ability to merge several PDF documents into one is another valuable feature of the Aspose.PDF Cloud Node.js SDK. Using the REST API, Software developers can easily load and merge multiple documents into one inside Node.js applications. This is useful for creating comprehensive reports or compiling related documents. Here is an example that shows how software developers can merge multiple PDF documents inside Node.js applications.
How to Merge Multiple PDF Files Inside Node.js Apps?
async function mergePdfs() {
const filesToMerge = ["doc1.pdf", "doc2.pdf"];
await pdfApi.putMergeDocuments(filesToMerge);
console.log("PDF documents merged successfully!");
}
mergePdfs();