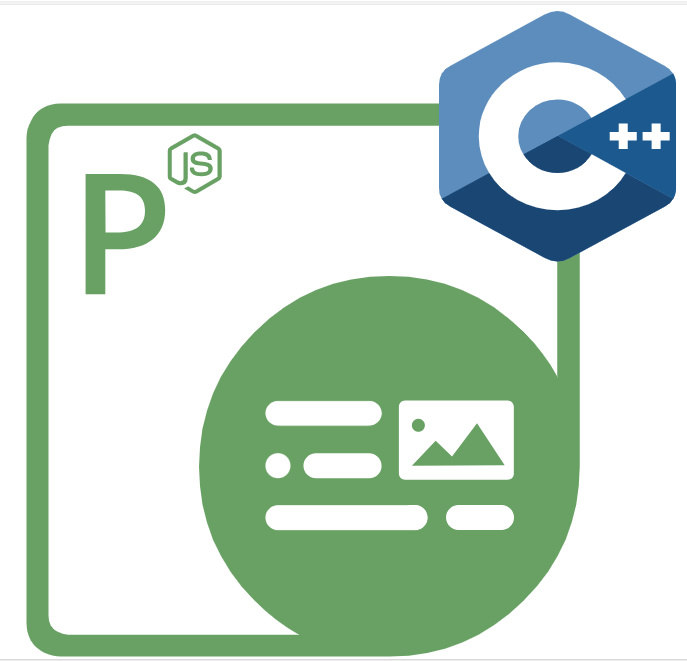
Aspose.PDF for Node.js via C++
Node.js API for PDF File Creation & Conversion
A Very Powerful Node.js Library for PDF Documents Processing Online. It Allows to create, Read, Modify, Render and Convert PDF Files inside Node.js Apps.
What is Aspose.PDF for Node.js via C++ ?
In the digital documentation, PDF files stand as a ubiquitous format for sharing information across platforms. From business reports to academic papers, PDFs encapsulate content in a standardized, portable format. However, managing PDFs programmatically can often pose challenges, especially when it comes to tasks like conversion, extraction, or modification. As businesses strive for seamless document handling, developers seek robust solutions that integrate seamlessly with their preferred programming languages and environments. This is where Aspose.PDF for Node.js via C++ steps in, offering a robust solution for software developers and programmers to seamlessly integrate PDF manipulation capabilities into their Node.js applications.
Aspose.PDF for Node.js via C++ is a sophisticated and easy to use library that empower software developers with the tools they need to handle PDF files efficiently. Leveraging the power of C++ under the hood, this library provides blazing-fast performance while ensuring seamless integration with Node.js applications. Whether you're extracting text, applying passwords to PDFs, creating new PDF files, converting existing PDFs to other formats, adding or deleting images, merging or splitting PDF files, adding or removing attachments from PDF, or adding annotations dynamically, Aspose.PDF equips you with a comprehensive set of features to accomplish your tasks with ease.
The integration of Aspose.PDF with Node.js via C++ opens up a world of possibilities for developers, allowing them to harness the power of C++ while enjoying the flexibility and simplicity of Node.js development. Moreover, it doesn't just stop at basic PDF operations; it extends its capabilities to advanced tasks like adding watermarks, merging or splitting PDFs, and even performing OCR (Optical Character Recognition) on scanned documents. With its rich feature set, seamless integration, and commitment to developer satisfaction, Aspose.PDF empowers software developers to unlock the full potential of PDF manipulation in their Node.js applications.
Getting Started with Aspose.PDF for Node.js via C++
To install and use Aspose.PDF with Node.js via C++ from a -*ZIP archive, follow the following instructions
- Extract the files from the ZIP archive
- Encrypt your *.lic file using ‘encrypt_lic.html
- Edit ‘settings.json’ and setup your settings
You can also download the library directly from Aspose.PDF product page
Convert PDF Files inside Node.js
Aspose.PDF for Node.js via C++ makes it easy for software professional to quickly and easily convert their PDF documents to the most popular formats in the Node.js environment. It allows seamless conversion of PDF files to various popular file formats including DOCX, XLSX, EPUB, HTML, images (JPEG, PNG, TIFF), and many more. This feature enables software developers to adapt PDF content to different platforms and use cases effortlessly. The following example shows, how software developers can convert existing PDF documents to XLSX file formats inside Node.js applications.
How to Convert PDF documents to XLSX Format inside Node.js Apps?
const AsposePdf = require('.//AsposePDFforNode.cjs');
const pdf_file = 'Aspose.pdf';
AsposePdf().then(AsposePdfModule => {
/*Convert a PDF-file to XlsX and save the "ResultPDFtoXlsX.xlsx"*/
const json = AsposePdfModule.AsposePdfToXlsX(pdf_file, "ResultPDFtoXlsX.xlsx");
console.log("AsposePdfToXlsX => %O", json.errorCode == 0 ? json.fileNameResult : json.errorText);
});
Extract Text from PDF in Node.js
Extracting text as well as images from the PDF document is a very demanding and useful task. Aspose.PDF for Node.js via C++ have provided numerous useful functions enabling software developers to extract text from PDF documents with precision, making it easy to process and analyze content programmatically. This feature is essential for tasks like text search, content analysis, and data extraction. The following code example demonstrates how users can extract text from their PDF documents inside Node.js applications.
How to Extract Text from PDF Document inside Node.js?
const AsposePdf = require('.//AsposePDFforNode.cjs');
const pdf_file = 'Aspose.pdf';
AsposePdf().then(AsposePdfModule => {
/*Extract text from a PDF-file*/
const json = AsposePdfModule.AsposePdfExtractText(pdf_file);
console.log("AsposePdfExtractText => %O", json.errorCode == 0 ? json.extractText : json.errorText);
});
Protect PDF Documents inside Node.js Apps
Aspose.PDF for Node.js via C++ provides features for securing PDF documents with just a couple of lines of code inside Node.js environment. There are several important features part of the library such as securely digitally sign PDF, setting passwords, restricting permissions, and encrypting or decrypting contents of a PDF documents. Below is an example demonstrating how software developers can encrypt a PDF document with a password inside Node.js applications.
How to Encrypt PDF File with Owner Password in Node.js?
const AsposePdf = require('asposepdfnodejs');
const pdf_file = 'Aspose.pdf';
AsposePdf().then(AsposePdfModule => {
/*Encrypt a PDF-file with passwords "user" and "owner", and save the "ResultEncrypt.pdf"*/
const json = AsposePdfModule.AsposePdfEncrypt(pdf_file, "user", "owner", AsposePdfModule.Permissions.PrintDocument, AsposePdfModule.CryptoAlgorithm.RC4x40, "ResultEncrypt.pdf");
console.log("AsposePdfEncrypt => %O", json.errorCode == 0 ? json.fileNameResult : json.errorText);
});
Optimize PDF Documents inside Node.js
Aspose.PDF for Node.js via C++ makes it easy for software developers to optimize PDF documents content for the Web. It Optimize PDF documents for reduced file size, improved performance, and compatibility inside Node.js applications. The library can also modify existing PDF documents by adding annotations, images, watermarks, and more. Here's an example demonstrating how to software developers can optimize PDF documents inside Node.js applications.
How to Optimize PDF Document for Web inside Node.js Apps?
const AsposePdf = require('.//AsposePDFforNode.cjs');
const pdf_file = 'Aspose.pdf';
AsposePdf().then(AsposePdfModule => {
/*Optimize a PDF-file and save the "ResultOptimize.pdf"*/
const json = AsposePdfModule.AsposePdfOptimize(pdf_file, "ResultOptimize.pdf");
console.log("AsposePdfOptimize => %O", json.errorCode == 0 ? json.fileNameResult : json.errorText);
});
Dynamic PDF Creation inside Node.js
Aspose.PDF for Node.js via C++ makes it easy for software developers to generate new PDF documents from scratch or dynamically create PDFs based on data inside Node.js applications. The library also supports adding pages, text, images, and other elements programmatically inside PDF documents.