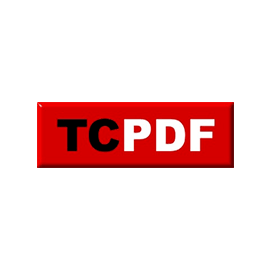
TCPDF
PHP Library for PDF Document Creation & Editing
Open Source PHP API to Generate PDF documents, Font subletting, JPEG or PNG and SVG images Native support, 1D and 2D Barcodes support.
The TCPDF is an open source PHP library that gives software developers the capability to generate PDF documents using PHP commands without any external dependencies. The great thing about TCPDF is that it is considered to be the only PHP-based library that includes complete support for UTF-8 Unicode and right-to-left languages.
The library supports several important features such as creating PDF documents, font subletting, images & graphic support, native support for JPEG, PNG, and SVG images, 1D and 2D barcode support, managing PDF page header and footer, encryption and decryption support, PDF annotations, table of content, text rendering modes, custom page formats, custom margins, page units and much more.
The library is considered to be one of the most popular and most used PHP libraries in the world because it has been included in the most popular PHP-based CMS and applications, including Joomla, Drupal, Moodle, phpMyAdmin, and so on.
Getting Started with TCPDF
TCPDF is available at packagist.org, so you can use the composer to download this library and all dependencies. Please use the following command for a smooth installation.
Install TCPDF Command
Install Package TCPDF
Generate PDF Documents using PHP Library
The free PHP library TCPDF has included the functionality that enables software programmers to programmatically create PDF documents inside their own PHP applications. You can use utf-8 encoding as well as support for Right-To-Left languages. Several important features like selecting font type and size, custom margins, units of measure, add barcode, add a table, insert pages, move the page, delete pages, and much more.
Create PDF Documents via PHP
// Include the main TCPDF library (search for installation path).
require_once('tcpdf_include.php');
// create new PDF document
$pdf = new TCPDF(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// set document information
$pdf->setCreator(PDF_CREATOR);
$pdf->setAuthor('Nicola Asuni');
$pdf->setTitle('TCPDF Example 038');
$pdf->setSubject('TCPDF Tutorial');
$pdf->setKeywords('TCPDF, PDF, example, test, guide');
Barcode Supports in PDF Files via PHP
The open source PHP library provides functionality for including Barcode inside PDF documents using a couple of lines of PHP code. Developers can use different types of 1D Barcode (CODE 39, CODE 128 AUTO, EAN 8, UPC-E, MSI, CODABAR, CODE 11, RMS4CC) and 2D Barcodes (QR-Code, Datamatrix ECC200, and PDF417) with ease. It also provides supports for features like setting Barcode height, Barcode alignment, set margins, apply checksum, & more.
Create PDF Documents via PHP
$pdf->SetFont('helvetica', '', 10);
// define barcode style
$style = array(
'position' => '',
'align' => 'C',
'stretch' => false,
'fitwidth' => true,
'cellfitalign' => '',
'border' => true,
'hpadding' => 'auto',
'vpadding' => 'auto',
'fgcolor' => array(0,0,0),
'bgcolor' => false, //array(255,255,255),
'text' => true,
'font' => 'helvetica',
'fontsize' => 8,
'stretchtext' => 4
);
// PRINT VARIOUS 1D BARCODES
// CODE 39 - ANSI MH10.8M-1983 - USD-3 - 3 of 9.
$pdf->Cell(0, 0, 'CODE 39 - ANSI MH10.8M-1983 - USD-3 - 3 of 9', 0, 1);
$pdf->write1DBarcode('CODE 39', 'C39', '', '', '', 18, 0.4, $style, 'N');
$pdf->Ln();
// CODE 39 + CHECKSUM
$pdf->Cell(0, 0, 'CODE 39 + CHECKSUM', 0, 1);
$pdf->write1DBarcode('CODE 39 +', 'C39+', '', '', '', 18, 0.4, $style, 'N');
$pdf->Ln();
// CODE 39 EXTENDED
$pdf->Cell(0, 0, 'CODE 39 EXTENDED', 0, 1);
$pdf->write1DBarcode('CODE 39 E', 'C39E', '', '', '', 18, 0.4, $style, 'N');
$pdf->Ln();
Add Custom Headers/Footers in PDF
The Headers and footers are very useful parts of a PDF document that helps users to organize their PDF files as well as easier to read. The open source library TCPDF makes developer’s jobs easy by including functionality for adding custom headers & footers to PDF documents with just a couple of lines of PP code. It supports features like setting fonts for headers and footers, setting margins, auto page breaks, adding images inside the header/footer, adding page numbers, and so on.
Set Custom Headers/Footers in PDF via PHP
// Extend the TCPDF class to create custom Header and Footer
class MYPDF extends TCPDF {
//Page header
public function Header() {
// Logo
$image_file = K_PATH_IMAGES.'logo_example.jpg';
$this->Image($image_file, 10, 10, 15, '', 'JPG', '', 'T', false, 300, '', false, false, 0, false, false, false);
// Set font
$this->SetFont('helvetica', 'B', 20);
// Title
$this->Cell(0, 15, '<< TCPDF Example 003 >>', 0, false, 'C', 0, '', 0, false, 'M', 'M');
}
// Page footer
public function Footer() {
// Position at 15 mm from bottom
$this->SetY(-15);
// Set font
$this->SetFont('helvetica', 'I', 8);
// Page number
$this->Cell(0, 10, 'Page '.$this->getAliasNumPage().'/'.$this->getAliasNbPages(), 0, false, 'C', 0, '', 0, false, 'T', 'M');
}
}
Create a Table of Contents
The open source PHP library TCPDF provides functionality for creating a table of contents inside their applications. The uses of a Table of Contents help readers to understand the structure of the documents and can quickly find the information they are looking for. To create a Table of contents you need to add a new page for TOC and can write the TOC title and/or other elements on the TOC page. You can also define styles for various bookmark levels for various HTML elements with ease.
How to Add TOC in PDF via PHP
// add a new page for TOC
$pdf->addTOCPage();
// write the TOC title
$pdf->SetFont('times', 'B', 16);
$pdf->MultiCell(0, 0, 'Table Of Content', 0, 'C', 0, 1, '', '', true, 0);
$pdf->Ln();
$pdf->SetFont('dejavusans', '', 12);
// add a simple Table Of Content at first page
// (check the example n. 59 for the HTML version)
$pdf->addTOC(1, 'courier', '.', 'INDEX', 'B', array(128,0,0));
// end of TOC page
$pdf->endTOCPage();
// ---------------------------------------------------------
//Close and output PDF document
$pdf->Output('example.pdf', 'I');
Manage PDF Annotations via PHP Library
Annotations are a complete set of objects which can be added to PDF pages without altering the page content. It further helps in the explanation to its content or to expand upon what is already present. The open source PHP library TCPDF provides supports for a variety of annotations creation such text annotations, link annotations, marking text, stamp annotations, and so on.
Add Text PDF Annotations via PHP
// set font
$pdf->SetFont('times', '', 16);
// add a page
$pdf->AddPage();
$txt = 'Example of Text Annotation.
Move your mouse over the yellow box or double click on it to display the annotation text.';
$pdf->Write(0, $txt, '', 0, 'L', true, 0, false, false, 0);
// text annotation
$pdf->Annotation(83, 27, 10, 10, "Text annotation example\naccented letters test: àèéìòù", array('Subtype'=>'Text', 'Name' => 'Comment', 'T' => 'title example', 'Subj' => 'example', 'C' => array(255, 255, 0)));
// ---------------------------------------------------------
//Close and output PDF document
$pdf->Output('example.pdf', 'I');