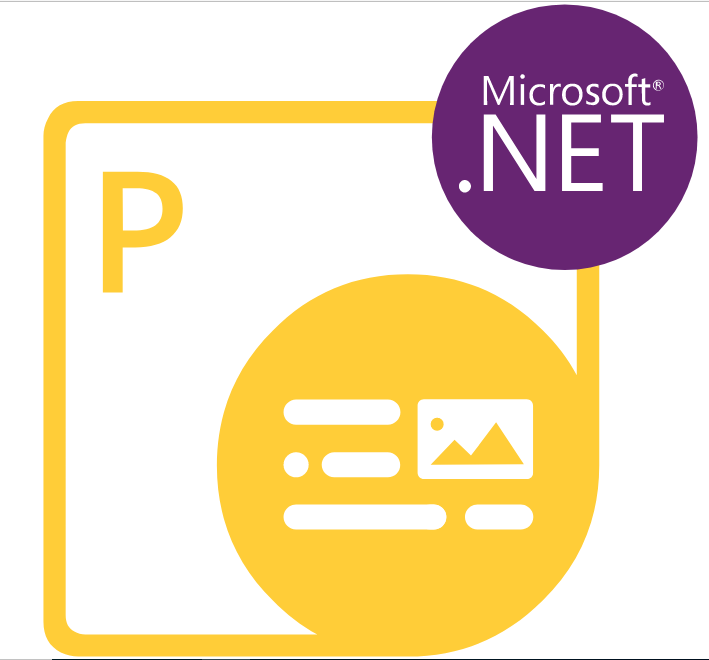
Aspose.PDF for Python via .NET
PDF Generation & Conversion via Python PDF API
Python PDF API to Create, Modify, Protect, Print, Process, split, merge, & Convert PDF Documents inside Python apps without using Adobe Acrobat.
Aspose.PDF for Python via .NET is a powerful PDF creation and manipulation API that enables software developers to work with PDF files in their Python applications via .NET libraries. Aspose.PDF for Python via .NET provides Python developers with access to these libraries through a .NET runtime. This means that Python developers can use Aspose.PDF to create, edit, and manipulate PDF files in their Python applications without needing Microsoft Office® or Adobe Acrobat Automation.
Aspose.PDF for Python via .NET has included support for a wide range of PDF processing features, including new PDF document creation from the scratch, loading & read PDF files, exporting PDFs to image formats, configuring PDF page properties, setting PDF width & height, handling text & paragraphs, PDF conversion to other file formats, extract text from PDF pages, search & replace text in PDFs, add & manage PDF attachments, insert new pages, split & merge PDF, move PDF pages, get the number of pages, get a particular page, insert TOC to existing PDF, optimize PDF Document for the Web and many more.
Aspose.PDF for Python has provided a very powerful PDF converter that enables software developers to export PDF documents to various other supported file formats such as Microsoft Word, Excel, PowerPoint, PDF/A, HTML, Images (BMP, JPEG, PNG), EPUB, Markdown, PCL, XPS, LATex/TeX, Text, PostScript and many more. The library comes with a comprehensive API documentation and sample code that developers can easily follow to get started with their PDF manipulation tasks. Whether users need to create new PDF files, extract data from PDF files, or convert PDF files to other formats, Aspose.PDF for Python via .NET is a great choice.
Getting Started with Aspose.PDF for Python via .NET
The recommend way to install Aspose.PDF for Python is using pip. Please use the following command for a smooth installation.
Install Aspose.PDF for Python via .NET using pip
pip install aspose-pdf
You can download the library directly from Aspose.PDF product page
PDF Documents Creation via Python API
Aspose.PDF for Python via .NET is a Powerful PDF documents processing API that allows software developers to create new PDF documents from the scratch with just a couple of lines of Python code. The library also provided several other features for PDF documents manipulation, such as open existing PDF files, add pages to existing PDF file, insert text from other pages, load PDF files from stream, add images to PDFs, split large PDF files into smaller one, combine multiple PDF files into a single one, delete unwanted pages from PDFs, print PDF files and many more.
Create a PDF file via Python
//import aspose.pdf as ap
# Initialize document object
document = ap.Document()
# Add page
page = document.pages.add()
# Initialize textfragment object
text_fragment = ap.text.TextFragment("Hello,world!")
# Add text fragment to new page
page.paragraphs.add(text_fragment)
# Save updated PDF
document.save("output.pdf")
Convert PDF Documents via Python API
Aspose.PDF for Python via .NET is a useful PDF generation API that allows software developers to covert PDF documents to numerous other supported file formats via Python API. Please remember that Aspose.PDF supports the largest number of popular document formats, both for loading and saving. The library has included support for PDF documents conversion to HTML, DOC, DOCX, PPTX,XLS, XLSX, XPS, SVG, XML, PS, PCL, MHT, HTML, EPUB, CGM, JPEG, EMF, PNG, BMP, GIF, TIFF, Text and many more. The library also supports converting from other formats to PDF with ease.
Convert PDF to DOCX in Python
import aspose.pdf as ap
input_pdf = DIR_INPUT + "sample.pdf"
output_pdf = DIR_OUTPUT + "convert_pdf_to_docx_options.docx"
# Open PDF document
document = ap.Document(input_pdf)
save_options = ap.DocSaveOptions()
save_options.format = ap.DocSaveOptions.DocFormat.DOC_X
# Set the recognition mode as Flow
save_options.mode = ap.DocSaveOptions.RecognitionMode.FLOW
# Set the Horizontal proximity as 2.5
save_options.relative_horizontal_proximity = 2.5
# Enable the value to recognize bullets during conversion process
save_options.recognize_bullets = True
# Save the file into MS Word document format
document.save(output_pdf, save_options)
Manage Pages & Attachments in PDFs via Python API
Aspose.PDF for Python via .NET library enables software developers to add pages and attachments to PDF documents inside Python applications. The library has include several important features for handling PDF pages, such as insert new pages to existing PDF, delete unwanted PDF pages, split large PDF to individual pages, moving bunch of Pages from one PDF document to another, change page size in PDF file, change page orientation, get page count, get Number of pages and so on.
Split PDF into Multiple Files via C# API
// The path to the documents directory.
string dataDir = RunExamples.GetDataDir_AsposePdf_Pages();
// Open document
Document pdfDocument = new Document(dataDir + "SplitToPages.pdf");
int pageCount = 1;
// Loop through all the pages
foreach (Page pdfPage in pdfDocument.Pages)
{
Document newDocument = new Document();
newDocument.Pages.Add(pdfPage);
newDocument.Save(dataDir + "page_" + pageCount + "_out" + ".pdf");
pageCount++;
}
Add & Manage PDF Annotations via Python
Annotation is a feature in PDF documents that allows users to add comments, notes, or other types of feedback to specific parts of the document. Annotations can be used for a variety of purposes, including collaboration, feedback, and review. Aspose.PDF for Python via .NET API allows software developers to insert PDF annotation inside their own Python applications. It supports features like adding, deleting and getting annotations from PDF documents with ease. There are many other types of annotations that you can add, and many other properties that you can set.
convert JPG Image to PDF via .NET API
// Load input JPG file
String path = dataDir + "Aspose.jpg";
// Initialize new PDF document
Document doc = new Document();
// Add empty page in empty document
Page page = doc.Pages.Add();
Aspose.Pdf.Image image = new Aspose.Pdf.Image();
image.File = (path);
// Add image on a page
page.Paragraphs.Add(image);
// Save output PDF file
doc.Save(dataDir + "ImagetoPDF.pdf");