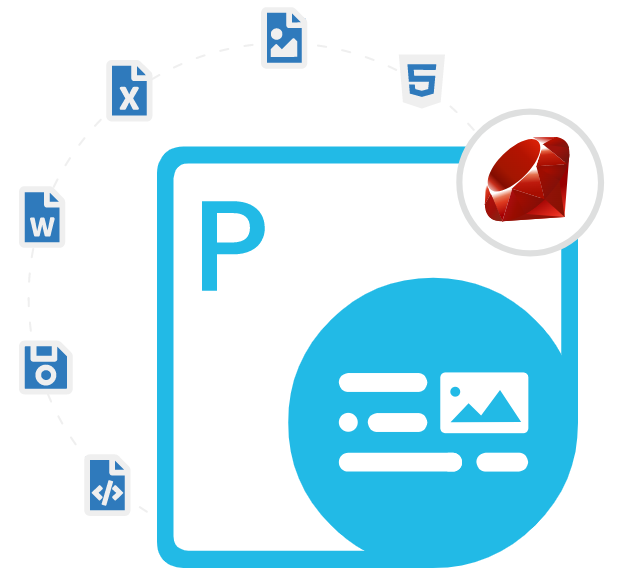
Aspose.PDF Cloud Ruby SDK
Python REST API for PDF Creation & Conversion
Generate, Edit, Protect, Process, Split, Merge, Manipulate & Convert PDF Documents to Other Formats via Python REST API inside Python apps without using Adobe Acrobat.
What is Aspose.PDF Cloud Ruby SDK?
Aspose.PDF Cloud Ruby SDK is an advanced tool that gives software developers the capability to work with PDF documents in the cloud. The Ruby software development kit makes it easy for programmers to create powerful applications for creating, editing, manipulating, and converting PDF files using simple and straightforward API calls. The SDK is platform-independent and can be used on any operating system or programming language that supports Ruby. It can be used to create PDF documents from different formats such as Empty PDF, HTML, XML, PCL, SVG, XPS, JPEG, TIFF, and more.
Aspose.PDF Cloud Ruby SDK is very easy to handle and has provided a wide range of features for working with PDF files such as creating PDF files from scratch, generating PDFs from existing (Microsoft Word, Excel, or PowerPoint) documents, PDF editing options, adding or deleting new PDF pages, inserting or deleting text to PDF, insert images to PDF files, Manage colors & styles, manage PDF fonts, merge multiple PDF files into a single file, split a PDF file into multiple files, split based on page count, page range, add digital signatures to PDF files and many more.
Aspose.PDF Cloud Ruby SDK provides a simple and intuitive REST API that is easy to use for developers of all levels. The SDK has provided very powerful features for converting PDF files to other supported file formats such as HTML, DOCX, JPEG, PNG, SVG, TIFF, and XPS using the SDK. The library can be used to work with various types of annotations such as line annotations, circle annotations, square annotations, underline annotations, and squiggly annotations. Whether software professionals need to create new PDF documents or edit, manipulate or convert existing PDF files, the Aspose.PDF Cloud Ruby SDK is an excellent choice for their next project.
Getting Started with Aspose.PDF Cloud Ruby SDK
The recommend way to install Aspose.PDF for Python is using RubyGems. Please use the following command for a smooth installation.
Install Aspose.PDF Cloud Ruby SDK using RubyGems
gem install ./aspose_pdf_cloud-23.2.0.gem
You can download the library directly from Aspose.PDF product page
Create PDF Files via Ruby API
Aspose.PDF Cloud Ruby SDK is a Powerful PDF documents processing API that allows software developers to create new PDF documents from the scratch with just a couple of lines of Python code. The library also provided several other features for PDF documents manipulation, such as opening existing PDF files, adding pages to existing PDF files, inserting text from other pages, loading PDF files from the stream, adding images to PDFs, splitting large PDF files into smaller one, combine multiple PDF files into a single one, delete unwanted pages from PDFs, print PDF files and many more.
Create PDF File from HTML via Ruby API
class Document
include AsposePDFCloud
include AsposeStorageCloud
def initialize
# Get App key and App SID from https://cloud.aspose.com
AsposeApp.app_key_and_sid("APP_KEY", "APP_SID")
@pdf_api = PdfApi.new
end
def upload_file(file_name)
@storage_api = StorageApi.new
response = @storage_api.put_create(file_name, File.open("../../../data/" << file_name,"r") { |io| io.read } )
end
def create_pdf_from_html
file_name = "newPDFFromHTML.pdf"
template_file = "sample.html"
upload_file(template_file)
response = @pdf_api.put_create_document(file_name, {template_file: template_file, template_type: "html"})
end
end
document = Document.new()
puts document.create_pdf_from_html
Split & Merge PDF Files via Ruby API
Aspose.PDF Cloud Ruby SDK has included some powerful features for handling PDF documents inside Ruby applications. The Ruby library allows software developers to merge or split their PDF documents with just a couple of lines of Ruby code in the cloud. It supports features like merging multiple PDF documents, splitting large PDF files, signing PDF documents, appending PDF documents, and many more. The following example shows how software developers can merge multiple PDF files in the cloud.
How to Merge Multiple PDF Files via Ruby REST API
PdfApi pdfApi = new PdfApi("API_KEY", "APP_SID");
String fileName = "sample-merged.pdf";
String storage = "";
String folder = "";
MergeDocuments body = new MergeDocuments();
body.List = new System.Collections.Generic.List { "sample.pdf", "input.pdf" };
try
{
// Upload source file to aspose cloud storage
pdfApi.UploadFile("sample.pdf", System.IO.File.ReadAllBytes(Common.GetDataDir() + "sample.pdf"));
pdfApi.UploadFile("input.pdf", System.IO.File.ReadAllBytes(Common.GetDataDir() + "input.pdf"));
// Invoke Aspose.PDF Cloud SDK API to merge pdf files
DocumentResponse apiResponse = pdfApi.PutMergeDocuments(fileName, storage, folder, body);
if (apiResponse != null && apiResponse.Status.Equals("OK"))
{
Console.WriteLine("Merge Multiple PDF Files, Done!");
Console.ReadKey();
}
}
catch (Exception ex)
{
System.Diagnostics.Debug.WriteLine("error:" + ex.Message + "\n" + ex.StackTrace);
}
Add & Manage PDF Pages via Ruby API
Aspose.PDF Cloud Ruby SDK has provided very useful features for handling PDF pages using Ruby API. The library has included very powerful features for working with PDF pages, such as adding new pages to PDF documents, counting PDF pages and the get the value, retrieving information about a particular PDF page, getting PDF document words count, removing unwanted pages from PDF documents, change position of pages inside PDF file, sign PDF page, convert PDF page into image format and many more. The following example demonstrates how software developers can export a PDF page to PNG image format via Ruby commands.
How to Delete Page from PDF via Ruby?
require 'aspose_pdf_cloud'
class Page
include AsposePDFCloud
include AsposeStorageCloud
def initialize
# Get App key and App SID from https://cloud.aspose.com
AsposeApp.app_key_and_sid("APP_KEY", "APP_SID")
@pdf_api = PdfApi.new
end
def upload_file(file_name)
@storage_api = StorageApi.new
response = @storage_api.put_create(file_name, File.open("../../../data/" << file_name,"r") { |io| io.read } )
end
# Delete document page by its number.
def delete_page
file_name = "sample-input.pdf"
upload_file(file_name)
page_number = 1
response = @pdf_api.delete_page(file_name, page_number)
end
end
page = Page.new()
puts page.delete_page
Manage PDF Header/Footers & Bookmarks via Ruby
Header and footer is a very important part of PDF documents that empower users to place important information about the document and makes it easy for readers to navigate the documents. Mostly it makes developer's life easy by including material that they want to appear on every page of a document. Aspose.PDF Cloud Ruby SDK has included complete support for adding Header and Footer to a PDF Document. The library lets users insert text, images, and other PDF files into Header and Footer using Ruby code. Software developers also easily add a new bookmark, update a bookmark, get all or specific bookmarks from PDF files and so on.
How to Get Specific Bookmark from PDF file via Ruby API?
class Bookmark
include AsposePDFCloud
include AsposeStorageCloud
def initialize
# Get App key and App SID from https://cloud.aspose.com
AsposeApp.app_key_and_sid("APP_KEY", "APP_SID")
@pdf_api = PdfApi.new
end
def upload_file(file_name)
@storage_api = StorageApi.new
response = @storage_api.put_create(file_name, File.open("../../../data/" << file_name,"r") { |io| io.read } )
end
# Read document bookmarks.
def read_document_bookmarks
file_name = "Sample-Bookmark.pdf"
upload_file(file_name)
response = @pdf_api.get_document_bookmarks(file_name)
end
end
bookmark = Bookmark.new()
puts bookmark.read_document_bookmarks