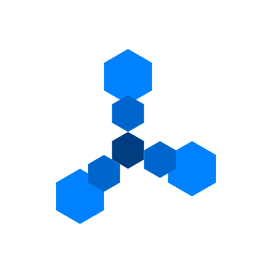
HexaPDF
Open Source Ruby Library for PDF Processing
Create & modify PDF documents, merge PDF files, reduce size of PDFs, add annotations, extract images & text via Open Source free Ruby library.
Portable Document Format (PDF) is a multi-platform file format that can be used to share and display documents in an electronic form independent of the software, hardware or operating system. HexaPDF is an open source PDF library that allows software developers to create powerful applications for working with PDF documents using Ruby code. It facilitates developers to create PDF files from scratch with minimum effort.
HexaPDF is a pure Ruby library that was designed to provide ease of use and improved performance. The library has included several important features related to PDF documents generation as well as manipulation such as opening & reading existing PDFs, modifying existing PDF files, meta information & text extraction, extracting images and files from PDFs, merging PDF files, encrypting or decrypting PDF files, optimizing PDF files for smaller file size and many more.
The library fully supports a high-level layer for composing a document of individual elements such as headers, paragraphs, links, emphasized text, and more. These elements are automatically adjusted, customized, and can be modified according to your needs. You can add additional element types with ease.
Getting Started with HexaPDF
For a smooth use of the HexaPDF library first important step is to install it. The recommended way for the installation is by using Rubygem. Please use the following command.
Install HexaPDF using Rubygem
$ gem install hexapdf
Create New PDFs using Ruby Library
The open source PDF library HexaPDF has provided complete functionality for creating new PDF documents from the scratch with just a couple of Ruby commands. You need an empty document instance for PDF creation. Once the empty PDF file is created now it is possible to add new pages to it, draw lines, curves, rectangles, insert text, and apply colors to it. You can also adjust line size and apply different colors and effects to it.
PDF Files Creation Using Ruby Library
require 'hexapdf'
doc = HexaPDF::Document.new
canvas = doc.pages.add.canvas
canvas.font('Helvetica', size: 100)
canvas.text("Hello World!", at: [20, 400])
doc.write("hello_world.pdf", optimize: true)
Merging PDF Files via Ruby
The Free PDF library HexaPDF makes is it easy for software programmers to combine their PDF documents using Ruby code. Merging PDF files can be performed using various ways. One simple way is to import pages from the source files into the target files. Which will preserve the page contents and then the merging command can be applied for merging files. For more complex merging please use the HexaPDF binary command.
Merging PDF Files using HexaPDF
# imports pages of the source files into the target file. Preserves the page contents themselves.
require 'hexapdf'
target = HexaPDF::Document.new
ARGV.each do |file|
pdf = HexaPDF::Document.open(file)
pdf.pages.each {|page| target.pages << target.import(page)}
end
target.write("2.merging.pdf", optimize: true)
Optimize PDF Size via Ruby Library
The open source PDF library HexaPDF helps software professionals to reduce the size of PDF documents inside Ruby applications. There are different kinds of techniques that can be used to optimize the size of PDF such as removing unused and deleted objects, using object and cross-reference streams and recompressing page content streams, font sub-setting, merging or object, and so on.
Read & Optimize PDF Files via Ruby
// Optimize PDF Size
require 'hexapdf'
HexaPDF::Document.open(ARGV.shift) do |doc|
doc.task(:optimize, compact: true, object_streams: :generate,
compress_pages: false)
doc.write('optimizing.pdf')
end
PDF Encryption & Decrypting Support
The HexaPDF library allows developers to secure their PDF documents by applying encryption using Ruby code. A PDF has built-in support for securing them by encrypting the content and assigning usage rights. During the PDF encryption, all strings and byte streams are encrypted and the metadata stream is exempted so that it can be extracted during the parsing of the PDF file. So anyone interested to view the PDF documents must provide the password.
Apply Digital Signature to PDF via Ruby
// Add Digital signatures to PDF
require 'hexapdf'
require HexaPDF.data_dir + '/cert/demo_cert.rb'
doc = HexaPDF::Document.open(ARGV[0])
doc.sign("signed.pdf", reason: 'Some reason',
certificate: HexaPDF.demo_cert.cert,
key: HexaPDF.demo_cert.key,
certificate_chain: [HexaPDF.demo_cert.sub_ca,
HexaPDF.demo_cert.root_ca])
end