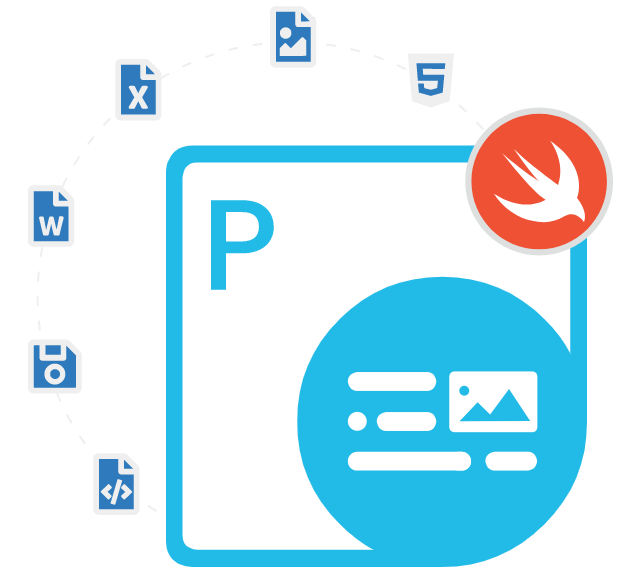
Aspose.PDF Cloud Swift SDK
Swift REST SDK to Create, Manipulate & Convert PDFs
PDF Swift Cloud API that enables software developers to develop Swift applications for generating, editing, manipulating & Converting PDF files in cloud.
What is One Aspose.PDF Cloud Swift SDK?
Aspose.PDF Cloud Swift SDK is a powerful and reliable PDF processing solution that enables software developers to create, edit, manipulate, and convert PDF documents inside their own Swift applications. The API has included very strong support for importing and exporting data from PDF documents as well as converting multiple file formats to PDF such as EPUB, Web, teX, MHT, HTML, PS, XPS, SVG, DOC, PCL, XML, Markdown and many more.
Aspose.PDF Cloud Swift SDK is very easy to handle and offers a wide range of features for working with PDF documents, such as PDF generation from scratch, PDF documents conversion to other supported file formats, adding PDF annotation, PDF form filling support, Importing data into PDF Documents, tables management in PDF Documents, insert image in PDF, add headers and footers, manage bookmarks and links in PDF, work with stamp, manage annotations, encrypting and decrypting PDF Documents, export data from PDF documents and so on.
Aspose.PDF Cloud Swift SDK has included very strong support for converting PDF documents to other supported file formats, such as MS Word (Doc, DocX), HTML, TIFF, SVG, ePUB, PPTX, TeX, MobiXML, XFA, XML, XPS, PDF/A, XLS and many more. With its user-friendly interface and comprehensive documentation, Aspose.PDF Cloud Swift SDK simplifies the process of integrating PDF processing capabilities into your Swift applications and enables software developers to handle even the most complex PDF processing tasks with ease.
Getting Started with Aspose.PDF Cloud Swift SDK
The recommend way to install Aspose.PDF Cloud Swift SDK is using CocoaPods. Please use the following commands for a smooth installation.
Install Aspose.PDF Cloud Swift SDK via CocoaPods
//First install CocoaPods
$ gem install cocoapods
// To integrate AsposePdfCloud into your Xcode project using CocoaPods, specify it in your Podfile:
source 'https://github.com/CocoaPods/Specs.git'
platform :ios, '10.0'
use_frameworks!
target '' do
pod 'AsposePdfCloud', '~> 20.12'
end
// Run the following command to complete the process
$ pod install
You can also download it directly from Aspose PDF product page.
Create PDF File from Other Files via Swift API
Aspose.PDF Cloud Swift SDK has included very useful features for generating high-quality PDF documents using a variety of methods. These methods include converting EPUB, Web, MHT, HTML, PS, XPS, DOC, PCL, XML, images, and other file formats to PDF, as well as creating PDF documents from scratch using Swift code. The Swift SDK also provides extensive support for setting document properties, such as title, author, subject, and keywords, import text, adding headers and footers to the PDF document and many more.
How to Create a Simple PDF File from XML via Swift API?
func createPDF(fromXML xmlData: Data, outputPath: String) {
// Parse the XML data and extract the relevant content and formatting information
// Create a PDF context
UIGraphicsBeginPDFContextToFile(outputPath, CGRect.zero, nil)
// Start a new PDF page
UIGraphicsBeginPDFPage()
// Draw the PDF content using Core Graphics
// ...
// End the PDF context and save the file
UIGraphicsEndPDFContext()
}
PDF Conversion to Other File Formats via Swift API
Aspose.PDF Cloud Swift SDK allows software developers to convert PDF documents to a variety of file formats, including DOC, DOCX, HTML, TIFF, SVG, ePUB, PPTX, TeX, MobiXML, XFA, XML, XPS, PDF/A, XLS and many more. This conversion process is fast and accurate, ensuring that the output file is an exact replica of the original PDF document. This feature is particularly useful when you need to extract data from PDF documents or when you want to make PDF documents accessible to users who don't have PDF readers installed on their devices.
How to Convert PDF File to PPTX via Swift API?
import AsposePdfCloud
// Set up credentials
let clientId = "your_client_id"
let clientSecret = "your_client_secret"
let apiBaseUrl = "https://api.aspose.cloud"
let config = Configuration(clientId: clientId, clientSecret: clientSecret)
let api = PdfApi(configuration: config)
// Convert PDF to PPTX
let fileName = "input.pdf"
let destFileName = "output.pptx"
let format = "pptx"
let outputFolder = "output"
let storage = "your_storage_name"
let folder = "your_folder_name"
let request = PostPdfInRequest(document: InputStream(data: pdfData), format: format, folder: folder, storage: storage, outPath: outputFolder+"/"+destFileName)
let response = try api.postPdfIn(request: request)
// Download converted file
let downloadRequest = GetDownloadFileRequest(path: response.path!)
let downloadResponse = try api.downloadFile(request: downloadRequest)
let pptxData = downloadResponse.body
Add & Manage PDF Annotation via Swift API
Aspose.PDF Cloud Swift SDK also offers very powerful annotation related features, allowing software developers to add text, link, circle, strikeout, line, images, and other annotations to PDF documents. This feature is particularly useful when you need to highlight important information or add comments to a document. The Swift SDK can be used to easily add annotations to PDF documents, customize their appearance, and save them for future reference.
How to Get PDF Page Annotations using Swift Commands?
// Get your ClientId and ClientSecret from https://dashboard.aspose.cloud (free registration required).
let pdfAPI = PdfAPI(clientId: "MY_CLIENT_ID", clientSecret: "MY_CLIENT_SECRET");
let pageNumber = 2
pdfAPI.getPageAnnotations(name: name, pageNumber: pageNumber, folder: self.tempFolder) {
(response, error) in
guard error == nil else {
// errror handle
return
}
if let response = response {
// do
}
}
Encrypt and Decrypt PDFs via Swift SDK
Aspose.PDF Cloud Swift SDK has included very powerful features for PDF documents encryption and decryption inside Swift cloud applications. The Swift SDK has included several important features for handling PDF document encryption and decryption, such as certify a PDF document, encrypt a PDF document by applying password, add signature fields to a PDF, read signature fields from PDF Document, decrypt PDF documents and many more. The following example shows how software developers can encrypt a PDF documents in the cloud using Swift code.
How to Encrypt PDF Documents via Swift API?
import AsposePDFCloud
let config = Configuration()
config.appKey = "your_app_key"
config.appSID = "your_app_SID"
let pdfApi = PdfApi(configuration: config)
let encryptOptions = EncryptDocument(
encryptionAlgorithm: EncryptionAlgorithm.aes,
ownerPassword: "owner_password",
permissions: Permissions.allowAll,
userPassword: "user_password")
let localFile = "local_file.pdf"
let encryptedFile = "encrypted_file.pdf"
pdfApi.putEncryptDocument(
name: localFile,
encryption: encryptOptions,
outPath: encryptedFile) { (response, error) in
if let error = error {
print("Error while encrypting document: \(error)")
} else {
print("Document encrypted successfully.")
}
}