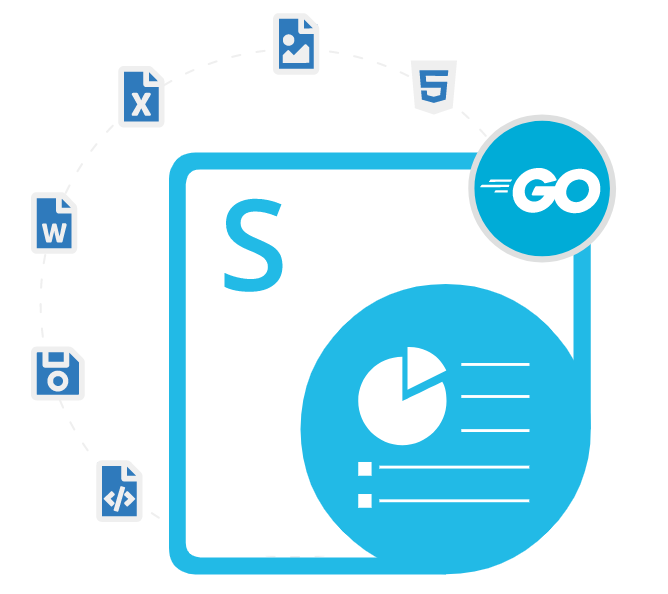
Aspose.Slides Cloud SDK for Go
GO SDK - Create & Convert PowerPoint Presentations
Leading Cloud-Based SDK allows Software Developers to Create, Read, Edit, Protect & Convert PowerPoint PPT/PPTX Presentations in the cloud.
What is Aspose.Slides Cloud SDK for Go?
Aspose.Slides Cloud SDK for Go iis a powerful tool for developers. It lets you work with PowerPoint presentations in the cloud using the Go programming language. This SDK is a game-changer, making it simple to add advanced PowerPoint features to your Go-based apps. You can create, edit, and remove slides, insert text, shapes, and multimedia elements, tweak formatting, and do much more with ease. The Go cloud SDK is based on the top of Aspose.Slides REST API and work smoothly without installing any third-party software. If you’re a developer looking to enhance your applications with advanced PowerPoint features, consider using Aspose.Slides Cloud SDK for Go. This cloud-based SDK provides a budget-friendly option without requiring costly software or hardware investments.
The Aspose.Slides Cloud SDK for Go is designed to work super-fast, thanks to cool features like asynchronous API calls and multitasking, making sure things run smoothly and quickly. It lets developers like you make and tweak PowerPoint presentations right within your own apps. You get handy tools like building presentations from scratch, adding new slides, combining or dividing presentations, copying slides contents, managing themes, adding & extract images from presentations, adding &extract shapes, manage hyperlinks, even pulling out specific slides from presentations and so on. Its compatibility across different platforms, user-friendly interface, top-notch performance, and affordability make it a smart pick for developers seeking to boost their apps with advanced PowerPoint capabilities.
Getting Started with Aspose.Slides Cloud SDK for Go
The recommend way to install Aspose.Slides Cloud SDK for Go is using GitHub.. To install the SDK on your system, please run the following command:
Install Aspose.Slides Cloud SDK for Go via GitHub
slides get github.com/aspose-slides-cloud/aspose-slides-cloud-slides
You can also download it directly from Aspose product release page.Create & Manage Presentation via Go API
Aspose.Slides Cloud SDK for Go has provided complete support for creating and modifying PPT and PPTX presentations inside Go applications. The SDK supports creating presentations in various popular presentation file formats such as PPT, PPTX, PPS, PPSX, PPTM, PPSM, POTX, POTM, ODP, OTP, and many more. There are several other important features part of the library for managing presentations in the cloud, such as adding new slides to existing presentations, adding & update slide notes, creating a presentation from HTML, creating a new presentation using a template, deleting unwanted slides from presentation, split or merge existing presentation, add animation to presentations, and many more.
Add, Manage & Convert Presentation’s Slides via Go API
Aspose.Slides Cloud SDK for Go has included complete support for handling slides inside PowerPoint presentations. There are several important features part of the library for working with slides, such as adding new slides to presentations, extracting slides from a presentation, deleting unwanted slides, copying slides from one presentation to another, moving slides to another position in a presentation, managing background presentation’s slide, manage slide comments, add comments to slide, get slide information, get the number of slide from a presentation and many more.
How to Add Comments to Presentation’s Slide via Go API?
cfg := asposeslidescloud.NewConfiguration()
cfg.AppSid = "MyClientId"
cfg.AppKey = "MyClientSecret"
api := asposeslidescloud.NewAPIClient(cfg)
dto := asposeslidescloud.NewSlideComment()
dto.Text = "Comment text"
dto.Author = "Author Name"
childComment := asposeslidescloud.NewSlideComment()
childComment.Text = "Child comment text"
childComment.Author = "Author Name"
childComments := []asposeslidescloud.ISlideCommentBase { childComment }
dto.ChildComments = childComments
comments, _, e := api.SlidesApi.CreateComment("MyPresentation.pptx", 3, dto, nil, "", "", "")
if e != nil {
fmt.Printf("Error: %v.", e)
return
}
fmt.Printf("The slide has %v comments", len(comments.GetList()))
Export PowerPoint Presentations via Go SDK
Aspose.Slides Cloud SDK for Go has provided some powerful features for converting PowerPoint presentations to various other support file formats using Go commands. Software developers to can convert presentations to PDF, XPS, TIFF,HTML, SWF, JPEG, PNG, GIF, BMP, FODP, XAML, MP4 and many more. It is also possible to convert selected slides, split or merge PowerPoint presentations, specify height or width of pages or images in an output document and many more. The following example demonstrates how to convert presentation’s to PDF file format.
How to Export Presentation to PDF via Go SDK ?
cfg := asposeslidescloud.NewConfiguration()
cfg.AppSid = "my_client_id"
cfg.AppKey = "my_client_key"
api := asposeslidescloud.NewAPIClient(cfg)
source, e := ioutil.ReadFile("MyPresentation.pptx")
if e != nil {
fmt.Printf("Error: %v.", e)
return
}
result, _, e := api.SlidesApi.Convert(source, "pdf", "", "", "", []int32 { 2, 4 }, nil)
if e != nil {
fmt.Printf("Error: %v.", e)
return
}
fmt.Printf("The converted file was saved to %v.", result.Name())
Insert & Manage Shapes in Presentation via Go API
Aspose.Slides Cloud SDK for Go has provided a complete set of features for working with shapes inside PowerPoint presentations using Go commands. It provides support for adding new shapes to presentations, managing shape properties, working with SmartArt graphics, working with Math formulas, extracting Shapes from a slide, adding & managing hyperlinks, working with geometry paths, working With zoom frames, adding and managing WordArt, importing shapes From SVG and many more. The following example demonstrates how to extract shapes from the Presentation slide using Go API.
How to Extract Shapes from Presentations using Go API?
cfg := asposeslidescloud.NewConfiguration()
cfg.AppSid = "MyClientId"
cfg.AppKey = "MyClientSecret"
api := asposeslidescloud.NewAPIClient(cfg)
// Get all shapes from the first slide.
allShapes, _, e := api.SlidesApi.GetShapes("MyPresentation.pptx", 1, "", "", "", "", "")
if e != nil {
fmt.Printf("Error: %v.", e)
return
}
// Get all charts from the first slide.
charts, _, e := api.SlidesApi.GetShapes("MyPresentation.pptx", 1, "", "", "", "Chart", "")
if e != nil {
fmt.Printf("Error: %v.", e)
return
}
// Print information about the shapes and charts.
shapeCount := len(allShapes.GetShapesLinks())
chartCount := len(charts.GetShapesLinks())
fmt.Printf("The slide contains %v shapes, including %v charts", shapeCount, chartCount)