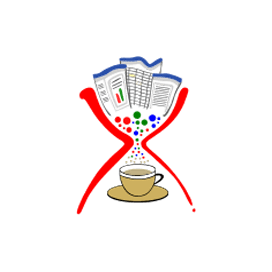
Apache POI XSLF
Open Source Java API for Managing PPTX Presentations
Create, Edit, Merge, Manipulate and Convert Microsoft PowerPoint OOXML Presentations via Open Source Leading Java Library.
What is Apache POI XLSF?
Apache POI XLSF is a Java implementation for reading, creating, or editing PowerPoint PPTX files. It provides the necessary functionality for working with PowerPoint 2007 OOXML file format, enabling developers to extract data such as text, images, embedded objects & more from PowerPoint PPTX presentations. Developers can also add shapes to a slide, manage hyperlinks & images, add videos, and convert PPTX to SVG.
Getting Started with Apache POI XLSF
First of all, you need to have the Java Development Kit (JDK) installed on your system. If you already have it then proceed to the Apache POI's download page to get the latest stable release in an archive. Extract the contents of the ZIP file in any directory from where the required libraries can be linked to your Java program. That is all!
Referencing Apache POI in your Maven-based Java project is even simpler. All you need is to add the following dependency in your pom.xml and let your IDE fetch and reference the Apache POI Jar files.
Apache POI Maven Dependency
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.0</version>
</dependency>
Java API to Create New Presentations & Append Slide to Existing File
Apache POI XLSF enables computer programmers to create new PowerPoint presentations in PPTX file format from scratch. Developers can also transform an existing presentation according to their needs. It provides the ability to read & modify the existing presentations as well as append slides to the existing presentation according to their need.
How to Create Presentation & Add Slide - Java?
// create a new PPTX file
FileOutputStream fileOutputStream = new FileOutputStream(new File("Slide.pptx"));
// create a new slide show
XMLSlideShow xmlSlideShow = new XMLSlideShow();
// save file
xmlSlideShow.write(fileOutputStream);
Create New Slide from a Predefined Slide Layout in Java Apps
Apache POI XLSF API has included support for adding new slides from a predefined slide layout inside PPTX presentation. Slide layouts contain formatting, positioning, and placeholder boxes for all of the content that appears on a slide. PowerPoint presentations have several slide layouts; first of all, you need to see which slide layouts are available for use. There are different slide masters and in each slide master, there are several slide layouts.
How to Set Title Layout Slide via Java API?
// create a new PPTX file
FileOutputStream fileOutputStream = new FileOutputStream(new File("Slidelayout.pptx"));
// create a new slide show
XMLSlideShow xmlSlideShow = new XMLSlideShow();
// initialize slide master object
XSLFSlideMaster xslfSlideMaster = xmlSlideShow.getSlideMasters().get(0);
// set Title layout
XSLFSlideLayout xslfSlideLayout = xslfSlideMaster.getLayout(SlideLayout.TITLE);
// create a new slide with title layout
XSLFSlide xslfSlide = xmlSlideShow.createSlide(xslfSlideLayout);
// select place holder
XSLFTextShape xslfTextShape = xslfSlide.getPlaceholder(0);
// set title
xslfTextShape.setText("Test");
// save file
xmlSlideShow.write(fileOutputStream);
// close stream
fileOutputStream.close();
Merge Multiple PPTX Presentations using Java
Do you have multiple PPTX presentations that you want to combine into one presentation? Apache POI XLSF APIs can surely help you and enables Java developers to merge their multiple PPTX files together.
How to Merge Presentation's Slides - Java?
// create a new PPTX file
FileOutputStream fileOutputStream = new FileOutputStream("MergegSlide.pptx");
// select two PPTX files
String[] inputFiles = {"Slide.pptx", "SlideLayout.pptx"};
// create a new slide show
XMLSlideShow slideShow = new XMLSlideShow();
// merge slides
for(String file : inputFiles){
FileInputStream inputstream = new FileInputStream(file);
XMLSlideShow xmlSlideShow = new XMLSlideShow(inputstream);
for(XSLFSlide srcSlide : xmlSlideShow.getSlides()) {
slideShow.createSlide().importContent(srcSlide);
}
}
// saving file
slideShow.write(fileOutputStream);
// close stream
fileOutputStream.close();