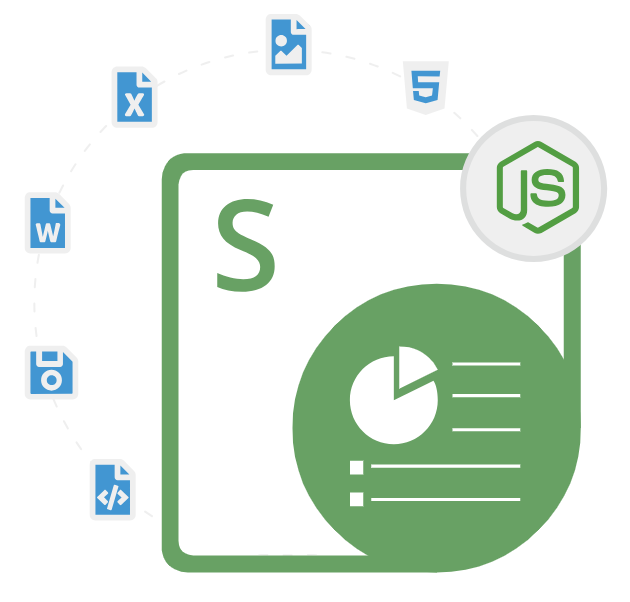
Aspose.Slides for Node.js via .NET
Node.js API to Create, Edit & Convert PowerPoint Presentations
An Advanced Node.js PowerPoint Presentation Library to Create, Edit, Split/Merge, Clone, Manipulate and Convert PPT, PPTX Files to PDF, JPG, HTML, GIF, SVG & many more inside Node.js Apps.
In today's fast-paced digital landscape, dynamic presentations are a key element in conveying information effectively. With the rise of Node.js and .NET technologies, software developers are constantly seeking efficient solutions to integrate presentation creation and manipulation into their applications seamlessly. Enter Aspose.Slides for Node.js via .NET – a powerful toolkit designed to streamline the process of working with presentations in Node.js environments, leveraging the robust capabilities of .NET. The API supports a wide range of PowerPoint formats, including PPT, PPTX, PPS, PPSX, and even older formats like PPTM and PPSM.
Aspose.Slides for Node.js via .NET offers a robust solution for software developers seeking to incorporate presentation generation and manipulation capabilities into their Node.js applications. The API is very easy to use and empowers software developers to automate the generation, modification, reading, manipulation and conversion of PowerPoint presentations within Node.js applications. By harnessing the extensive features of the .NET platform, this toolkit offers a comprehensive set of tools for handling presentations, slides, shapes, text, images, charts, and much more.
Aspose.Slides for Node.js via .NET offers robust support for PowerPoint document conversion. Software developers can easily convert PowerPoint presentations to popular presentation formats, such as PPT, PPTX, and ODP as well as various other formats such as PDF, JPG, HTML, GIF, SVG, and many other popular file formats. With its comprehensive feature set, seamless integration with Node.js via .NET, and robust support for document conversion, Aspose.Slides empowers software developers to create dynamic, visually stunning presentations with ease.
Getting Started with Aspose.Slides for Node.js via .NET
The recommend way to install Aspose.Slides for Node.js via .NET is using npm. Please use the following command for a smooth installation.
Install Aspose.Slides for Node.js via .NET via npm
npm install aspose.slides.via.net
You can also download it directly from Aspose product release page.Create New Presentations(PPT, PPTX) inside Node.js
With Aspose.Slides for Node.js via .NET, software developers can programmatically generate new PowerPoint presentations from scratch with just a couple of lines of code inside Node.js environment. This feature allows for dynamic content generation and customization. Moreover, developers can also modify existing PowerPoint presentations by adding, removing, or updating slides, shapes, text, images, and many more. The following example shows how developers can generate new PPTX presentation inside their Node.js applications.
How to Generate New PPTX Presentation inside Node.js?
const asposeSlides = require('aspose.slides.via.net');
const { Presentation, SaveFormat, ShapeType } = asposeSlides;
var pres = new Presentation();
try
{
var slide = pres.slides.get(0);
slide.shapes.addAutoShape(ShapeType.Rectangle, 50, 150, 300, 200);
pres.save("pres.pptx", SaveFormat.Pptx);
}
finally
{
if (pres != null) pres.dispose();
}
Convert PowerPoint Presentation to PDF in Node.js
Aspose.Slides for Node.js via .NET has included a powerful set of features for converting PowerPoint Presentation to other supported file formats inside Node.js environment. The API enables software developers to convert PowerPoint (PPT, PPTX) presentations to various popular file formats such as PDF, images (PNG, JPEG, TIFF, etc.), HTML, and many more. The following example demonstrates how to convert a PowerPoint presentation to a PDF document inside Node.js applications.
How to Convert a PowerPoint Presentation to PDF Document?
const asposeSlides = require('aspose.slides.via.net');
const { Presentation, SaveFormat } = asposeSlides;
var pres = new Presentation("pres.pptx");
try
{
pres.save("pres.pdf", SaveFormat.Pdf);
}
finally
{
if (pres != null) pres.dispose();
}
Manipulate Existing PowerPoint Presentations
Aspose.Slides for Node.js via .NET is a robust and feature-rich API that provides extensive functionalities to work with PowerPoint presentations programmatically. It allows software developers to load and modify existing PowerPoint presentations inside Node.js applications. There are several important features part of the API, such as adding, removing, or updating slides, shapes, text, images, and so on. The following example shows how developers can load an existing presentation and apply various properties inside Node.js environment.
How to Load and Modify Existing Presentation inside Node.js Apps?
// Open an existing presentation
using (Presentation presentation = new Presentation("existing_presentation.pptx"))
{
// Access slides and shapes
ISlide slide = presentation.Slides[0];
IAutoShape shape = slide.Shapes[0];
// Modify content
shape.TextFrame.Text = "Updated Text";
// Save changes
presentation.Save("updated_presentation.pptx", SaveFormat.Pptx);
}
Add & Format Text/Images to Presentation in Node.js
Aspose.Slides for Node.js via .NET has provided complete support for adding and managing text and images inside PowerPoint presentation in Node.js environment. Software developers can add text to slides and customize its formatting, including font size, color, style, alignment, bullet points, and more. Moreover, it enables software developers to add, remove, and customize shapes (such as rectangles, circles, lines) and images on slides with ease. The following example demonstrates how to add and format text on slide inside Node.js application.
How to Add and Format Text on a Slide inside Node.js?
// Add text to a slide
ISlide slide = presentation.Slides[0];
ITextFrame textFrame = slide.Shapes.AddTextFrame("Hello, World!");
// Format text
textFrame.Paragraphs[0].Portions[0].FontHeight = 24;
textFrame.Paragraphs[0].Portions[0].FontBold = true;
textFrame.Paragraphs[0].Portions[0].FontColor.Color = Color.Red;