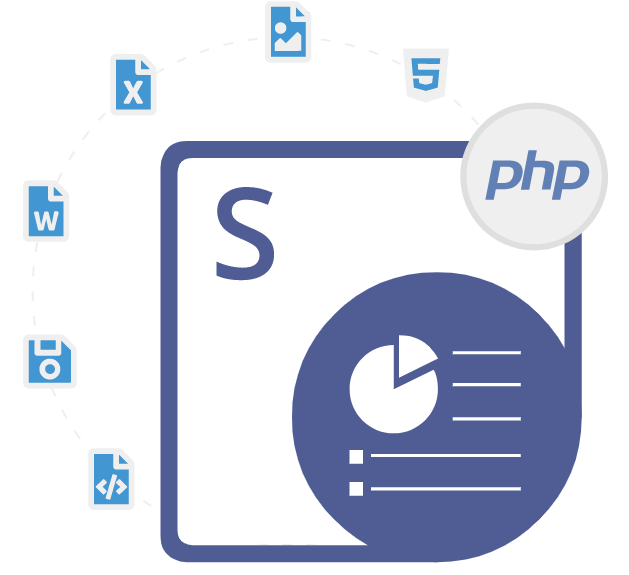
Aspose.Slides for PHP via Java
PHP API to Create & Convert PowerPoint Presentations
A leading PHP PowerPoint presentation API enables programmers to generate, read, edit, merge, protect & convert PowerPoint PPT/PPTX files.
Aspose.Slides for PHP via Java is a leading PowerPoint presentations library that enables software developers to work with Microsoft PowerPoint presentations using PHP code by utilizing the features of Java. It is a wrapper around the Aspose.Slides for Java library that enables PHP developers to take advantage of the powerful features and functions provided by Aspose.Slides. The library makes it easy for software developers to create, modify, read, view, merge, protect, convert, & render PowerPoint presentation files without using Microsoft PowerPoint or any 3rd party software.
Aspose.Slides for PHP via Java is built on top of the Aspose.Slides for Java library, which provides a comprehensive set of features for working with PowerPoint presentations. Some of these features include generating PowerPoint presentations from scratch, Load and modifying existing PowerPoint presentations, converting PowerPoint presentations to other formats, inserting and managing slides in presentations, working with animations and transitions, inserting and modifying shapes or text, adding images or charts to slide, work with tables and many more. It can be used on any platform (Windows, Linux, MacOS, etc.) where PHP 7 or higher is installed. Oracle JDK 7 or higher is another requirement.
Aspose.Slides for PHP via Java has included support for various leading presentation file formats such as PPT, PPTX, PPS, POT, PPSX, PPTM, PPSM, POTX, POTM, and ODP. The library allows software developers to convert presentations to some popular file formats such as PDF, Word, JPG, HTML, GIF, SVG, and many other formats. It also supports encrypting and decrypting presentations, password-protecting presentations, and removing passwords with ease. Overall, Aspose.Slides for PHP via Java is a great choice for creating, editing, and working with PowerPoint presentations.
Getting Started with Aspose.Slides for PHP via Java
The recommend way to install Aspose.Slides for PHP via Java is using composer. Use packagist to install our PHP library for Presentation processing from the PHP Package repository.
Install Aspose.Slides for PHP via Java via composer
composer require aspose/slides
You can also download it directly from Aspose product release page.Create PowerPoint PPT & PPTX Files via PHP API
Aspose.Slides for PHP via Java has included some powerful features for creating and managing PowerPoint presentations inside their own PHP applications. The library allows software developers to create presentation from scratch, add new slides to existing presentations, inserting audio and videos files to slides, adding images inside presentations, Reading or modifying a presentation’s document properties, applying protection on presentation, copying or cloning slides to the same or another presentation.
Create Presentations & Add a Side to it via PHP API
<?php
require_once("http://localhost:8080/JavaBridge/java/Java.inc");
require_once("lib/aspose.slides.php");
use aspose\slides;
use aspose\slides\Presentation;
use aspose\slides\ShapeType;
use aspose\slides\SaveFormat;
$pres = new Presentation();
try
{
// Gets the first slide
$slide = $pres->getSlides()->get_Item(0);
// Adds an autoshape with type set to line
$slide->getShapes()->addAutoShape(ShapeType::Line, 50, 150, 300, 0);
$pres->save("NewPresentation_out.pptx", SaveFormat::Pptx);
}
finally
{
if ($pres != null) $pres->dispose();
}
?>
Add Multimedia Files to Presentations via PHP API
Aspose.Slides for PHP via Java library gives software developers the capability to insert multimedia files inside their PowerPoint presentations using PHP code. The library has included several important features for handling multimedia files such as Adding new video frames to presentations, managing existing video frames, inserting new audio frames to presentations, managing existing audio files, deleting unwanted video or audio frames, and so on. It is also possible to customize the video or audio frame by setting various properties such as position, size, loop, and volume.
How Add Audio or Video File to a Presentation Slide via PHP API
<php use Aspose\Slides\VideoFrame;
use Aspose\Slides\AudioFrame;
$videoFrame = $presentation->getSlides()->get_Item(0)->getShapes()->addVideoFrame(50, 50, 320, 240, '/path/to/video.mp4');
$audioFrame = $presentation->getSlides()->get_Item(1)->getShapes()->addAudioFrame(100, 100, 100, 100, '/path/to/audio.mp3');
// customize the video or audio
$videoFrame->setLooping(true);
$audioFrame->setVolume(50);
//Finally Save Presentation to a file or stream.
$presentation->save('/path/to/new/presentation.pptx', Java('com.aspose.slides.SaveFormat')->Pptx);
?>
Presentation Conversion to Other Formats via PHP API
Aspose.Slides for PHP via Java is a very feature-rich API that makes software developer's jobs easy by providing them with complete support for creating and converting PowerPoint presentations file formats to several other supported file formats, such as PDF, PDF/A, XPS, HTML, PNG, JPEG, Word, TIFF, GIF, SWF flash, Video and many more. It is also possible to convert a slide to SVG images and other image formats. The API also provided support for converting OpenOffice ODP presentations to other file formats same as PowerPoint types. The following example demonstrates how to export a PPTX presentation file to PDF file format using PHP commands.
Convert Presentation to PDF in PHP Apps
<php
require_once("http://localhost:8080/JavaBridge/java/Java.inc");
require_once("lib/aspose.slides.php");
use aspose\slides;
use aspose\slides\Presentation;
use aspose\slides\ShapeType;
use aspose\slides\SaveFormat;
// Instantiate a Presentation object that represents a PPT file
$pres = new Presentation("PowerPoint.ppt");
try
{
// Save the presentation as PDF
$pres->save("PPT-to-PDF.pdf", SaveFormat::Pdf);
}
finally
{
if ($pres != null) $pres->dispose();
}
?>
Merge PowerPoint presentations using PHP API
Aspose.Slides for PHP via Java has included a very useful feature for PowerPoint presentation merging and splitting inside PHP applications. The API enables software developers to merge PowerPoint presentations in the same format such as PPT to PPT or PPTX to PPTX, etc. as well as merge presentations in different file formats such as PPT to PPTX or PPTX to ODP, etc. It provides support for merging entire presentations, merging selected slides into a presentation. Using Aspose.Slides for PHP also support merging other files such as images, like JPG to JPG or PNG to PNG or documents like PDF to PDF or HTML to HTML, and so on.
Copy Slide from Source Presentation to Merged Presentations via PHP API
<php use Aspose\Slides\Examples\PHP\Merging\SimplePresentationMerge;
use Aspose\Slides\Examples\Utils\Utils;
require_once("../vendor/autoload.php");
use com\aspose\slides\Presentation;
use com\aspose\slides\SaveFormat;
// Instantiate first presentation
$presentation1 = new Presentation("presentation1.pptx");
// Instantiate second presentation
$presentation2 = new Presentation("presentation2.pptx");
// Create new presentation object
$mergedPresentation = new Presentation();
// Copy slides from first presentation to merged presentation
foreach ($presentation1->getSlides() as $slide) {
$mergedPresentation->getSlides()->addClone($slide);
}
// Copy slides from second presentation to merged presentation
foreach ($presentation2->getSlides() as $slide) {
$mergedPresentation->getSlides()->addClone($slide);
}
// Save merged presentation
$mergedPresentation->save("mergedPresentation.pptx", SaveFormat::Pptx);
?>