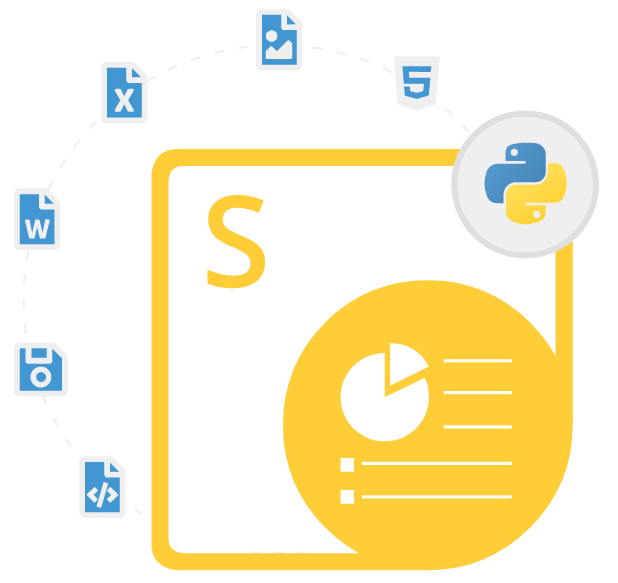
Aspose.Slides for Python via .NET
Python API to Create & Convert PowerPoint Presentations
A PowerPoint Python API for PPT/PPTX presentation creation, editing, spliting, merging, & conversion without using Microsoft Office PowerPoint.
What is Aspose.Slides for Python via .NET?
If you’re a software developer working with Microsoft PowerPoint presentations in Python, Aspose.Slides for Python via .NET can be a handy tool. This library lets you manage PowerPoint presentations directly in your Python apps. By integrating with .NET, it gives you the power to work with Aspose.Slides seamlessly. With this library, you can perform various tasks like reading, writing, editing, displaying, and controlling PowerPoint presentations using simple Python commands. It can be used on multiple platforms, including Windows, Linux, and macOS.
Aspose.Slides for Python via .NET is built to be user-friendly and offers a wide array of features. It’s perfect for creating and editing PowerPoint presentations. With this tool, you can generate and read presentations in different file formats like PPT, PPTX, PPS, POT, PPSX, PPTM, PPSM, POTX, POTM, ODP, PDF, and more. Additionally, you can export presentations to various popular file formats such as PDF, PDF/A, XPS, JPEG, PNG, BMP, TIFF, GIF, SVG, HTML, and others.
The Aspose.Slides for Python through .NET library offers a range of tools for managing PowerPoint presentations. With this library, you can do things like start presentations from scratch, insert or delete slides, adjust slide settings, add shapes, edit shape details, tweak font styles, change colors, align text, and incorporate multimedia like audio and video into your presentations. Aspose.Slide library is very easy to use and provides a wide range of features making it an ideal choice for creating and modifying PowerPoint presentations.
Getting Started with Aspose.Slides for Python via .NET
The recommend way to install Aspose.Slides for Python via .NET is using pip. Please use the following command for a smooth installation.
Install Aspose.Slides for Python via .NET via pip
pip install aspose.slides
You can also download it directly from Aspose product release page.PowerPoint Presentation Generation via Python
Aspose.Slides for Python via .NET is a very useful solution for creating and managing PowerPoint presentations inside their own Python applications. The library has included several important features for handling presentations such as add slides to existing presentations, examine presentation, merge multiple presentations, insert images inside presentations, insert notes to presentations, merge specific slides from presentations, merge slides to presentation section, convert presentation to PDF and many more.
How to Merge Presentations via Python API?
import aspose.slides as slides
with slides.Presentation("pres.pptx") as pres1:
with slides.Presentation("Presentation1.pptx") as pres2:
for slide in pres2.slides:
pres1.slides.add_clone(slide)
pres1.save("combined.pptx", slides.export.SaveFormat.PPTX)
Add & Manage Slides in Presentations via Python API
Aspose.Slides for Python via .NET has included complete support for handling slides inside PowerPoint presentation using Python commands. Software developers can perform various operations for managing slides inside their PowerPoint presentations, such as adding slides to presentation, access slide in presentation, delete unwanted slides from the presentation, clone slides, compare slides, adjust slide layout, convert slides to image file formats, convert slides with custom sizes, set slide masters, copy slide contents, add slide transition, set transition effects and many more.
Create Simple Slide Transition effect via python API
import aspose.slides as slides
# Instantiate Presentation class to load the source presentation file
with slides.Presentation(path + "AccessSlides.pptx") as presentation:
# Apply circle type transition on slide 1
presentation.slides[0].slide_show_transition.type = slides.slideshow.TransitionType.CIRCLE
# Apply comb type transition on slide 2
presentation.slides[1].slide_show_transition.type = slides.slideshow.TransitionType.COMB
# Write the presentation to disk
presentation.save("SampleTransition_out.pptx", slides.export.SaveFormat.PPTX)
Add Comments to Presentations in Python Apps
Comments are very useful part of the presentations that helps users to provide feedback or communicate with their teammates when review presentations. Aspose.Slides for Python via .NET has provided complete support for adding and managing comments on presentations inside Python applications. The library has included various features for handling presentations, such as adding comments to a slide in a PowerPoint presentation, access an existing comment on a slide, reply to comments, add a modern comment to a slide, delete all comments and authors, delete specific comments on a slide and many more.
How to Delete Specific Comments on a Slide via Python API?
import aspose.pydrawing as draw
import aspose.slides as slides
from datetime import date
with slides.Presentation() as presentation:
slide = presentation.slides[0]
# add comments...
author = presentation.comment_authors.add_author("Author", "A")
author.comments.add_comment("comment 1", slide, draw.PointF(0.2, 0.2), date.today())
author.comments.add_comment("comment 2", slide, draw.PointF(0.3, 0.2), date.today())
# remove all comments that contain "comment 1" text
for commentAuthor in presentation.comment_authors:
toRemove = []
for comment in slide.get_slide_comments(commentAuthor):
if comment.text == "comment 1":
toRemove.append(comment)
for comment in toRemove:
commentAuthor.comments.remove(comment)
presentation.save("pres.pptx", slides.export.SaveFormat.PPTX)
Work With Hyperlinks in Presentations via Python API
Aspose.Slides for Python via .NET has provided some useful features for handling hyperlinks inside PowerPoint presentations using Python API. The library allows software developers to add URL hyperlinks to texts, adding URL hyperlinks to shapes or frames, adding URL hyperlinks to media files, formatting hyperlinks, using hyperlinks to create table of contents, mutable hyperlink, supported properties in IHyperlinkQueries and many more. The following example demonstrates how to add a website hyperlink to a text using Python code.
How to Add a Website Hyperlink to a Text via Python API?
import aspose.slides as slides
with slides.Presentation() as presentation:
shape1 = presentation.slides[0].shapes.add_auto_shape(slides.ShapeType.RECTANGLE, 100, 100, 600, 50, False)
shape1.add_text_frame("Aspose: File Format APIs")
shape1.text_frame.paragraphs[0].portions[0].portion_format.hyperlink_click = slides.Hyperlink("https://www.aspose.com/")
shape1.text_frame.paragraphs[0].portions[0].portion_format.hyperlink_click.tooltip = "More than 70% Fortune 100 companies trust Aspose APIs"
shape1.text_frame.paragraphs[0].portions[0].portion_format.font_height = 32
presentation.save("presentation-out.pptx", slides.export.SaveFormat.PPTX)