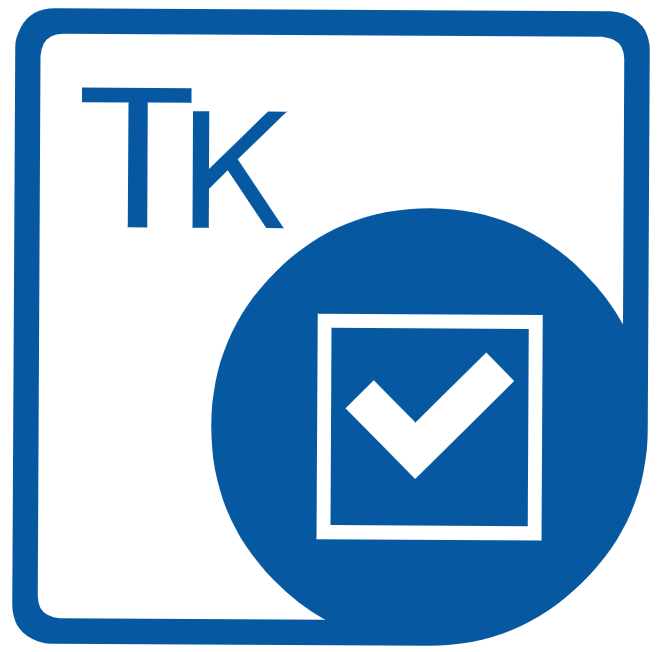
Aspose.Tasks for C++
C++ API to Read, Write & Convert Microsoft Project Files
A Leading C++ Project Management Library Allows Software Developers to Create, Modify, Manipulate, Read and Convert Microsoft Project MPP, MPS Files to PDF, Excel, XPS & Image Formats inside C++ Apps.
What is Aspose.Tasks for C++?
In the dynamic field of project management, effectiveness is paramount. Having the appropriate tools at your disposal can make all the difference when managing complex corporate strategies, software development cycles, or large-scale construction projects. Aspose.Tasks for C++ stands out as a game-changer since it provides software developers with a powerful toolset that allows them to work with Microsoft Project files without installing Microsoft Project. It supports cross-platform development and is designed to work with Windows, Linux, and macOS systems. It is easy to incorporate into your development workflow and guarantees reliable performance on all of these platforms.
Aspose.Tasks for C++ is designed to empower software developers with the capability to create, edit, manipulate, and convert Microsoft Project files programmatically using C++ language. This library provides a comprehensive set of features, enabling software developers to read, write, and render Microsoft Project documents, facilitating seamless integration of project management functionalities into their applications. The API facilitates advanced data manipulation, allowing developers to extract valuable insights from project files.
One of the standout features of Aspose.Tasks for C++ is its ability to handle various Microsoft Project file formats, including MPP, XML, and MPT. This versatility ensures compatibility with a wide range of project files, allowing developers to work with different versions of Microsoft Project effortlessly. The library offers a plethora of functionalities to streamline project management tasks. Developers can manipulate tasks, resources, calendars, and other project elements programmatically, providing full control over project structures and schedules. It is a versatile library that simplifies project management tasks by offering features such as reading and modifying project files, resource management, and report generation, empowering developers to build robust solutions tailored to their project management needs.
Getting Started with Aspose.Tasks for C++
The recommend way to install Aspose.Tasks for C++ is via NuGet. Please use the following command for a smooth installation.
Install Aspose.Tasks for C++ via NuGet
install-Package Aspose.Tasks.Cpp
You can download the directly from Aspose.Tasks Release pageNew Project Generation via C++ API
Aspose.Tasks for C++ makes it easy for software developers to create new empty projects from scratch inside their own C++ applications without using Microsoft Project. Software developer can create Project files in different file formats such as MPP and XML. There are several important features part of the library, such as opening and read existing project files, modifying project files, convert project files to other supported file format and many more. The following example demonstrates how C++ developers can create any empty project file with just a couple of lines of code and save it in XML format.
How to Create an Empty Project File using C++ API?
// Create empty project
System::SharedPtr project = System::MakeObject();
// Save project as xml
project->Save(dataDir + u"EmptyProjectSaveXML_out.xml", Aspose::Tasks::Saving::SaveFileFormat::XML);
Convert Project Data to PDF via C++
Aspose.Tasks for C++ is a powerful library designed to facilitate the manipulation and conversion of Microsoft Project files in various formats. One of the key functionalities it offers is the ability to convert Project data into PDF format. This feature proves invaluable for project managers and stakeholders who need to share project information in a universally accessible format. It also allows saving project data to multiple PDF files, customize the text style for project data, customizing the date format, setting fonts and many more. Below is a simple example demonstrating how to convert a Microsoft Project file (MPP) to PDF using C++ commands.
How to Save Project to PDF File using C++ Applications?
// Read the input Project file
System::SharedPtr project = System::MakeObject(dataDir + u"CreateProject2.mpp");
// Save the Project as PDF
project->Save(dataDir + u"SaveProjectAsPDF_out.pdf", Aspose::Tasks::Saving::SaveFileFormat::PDF);
Reading Project Files via C++ API
One of the primary features of Aspose.Tasks for C++ is its ability to load and read Microsoft Project files inside C++ applications without using Microsoft Project. With just a few lines of code, you can extract essential information from project files. The library also supports accessing project properties and iterate through various tasks. Moreover, you can read and write default project properties as well as calendar properties. The following example demonstrates how software developers can load and read project file inside their own C++ applications.
How to Load and Read Project Files using C++ Applications?
// Load a project file
auto project = System::MakeObject(u"input.mpp");
// Access project properties
auto projectInfo = project->get_ProjectProperties();
auto projectName = projectInfo->get_Name();
// Iterate through tasks
auto tasks = project->get_RootTask()->get_ChildTasks();
for (const auto& task : tasks) {
auto taskName = task->get_Name();
auto startDate = task->get_Start()->ToShortDateString();
auto endDate = task->get_Finish()->ToShortDateString();
// Process task details
}
Project Resources Allocation in C++ Apps
Managing resources is essential for effective project management. Aspose.Tasks for C++ allows software developers to handle resources effortlessly, whether it's adding new resources or assigning them to tasks. This library provides tools to assign resources, set work or material costs, and optimize resource usage, ensuring that projects are completed within budget and on time. The following example shows how to work with project resources inside C++ applications.
How to Access and Manage Project Resources inside C++ Applications?
// Access project resources
auto resources = project->get_Resources();
// Add a new resource
auto newResource = System::MakeObject(u"John Doe");
resources->Add(newResource);
// Assign resources to tasks
task1->get_Resources()->Add(newResource);
// Save the modified project
project->Save(u"output.mpp", SaveFileFormat::MPP);