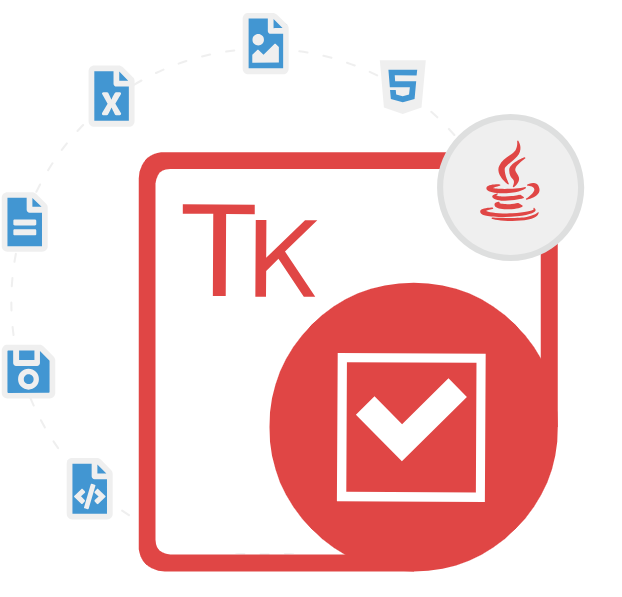
Aspose.Tasks for Java
Java Project Management API to Create & Convert Microsoft Project Files
The API allows to Create user-friendly Project Management Solutions to Read Project Data, Generate Reports & Export Project Data to PDF, XPS & Image Formats.
What is Aspose.Tasks for Java?
In the world of project management, having the correct tools can be a game-changer. Aspose.Tasks for Java is a robust solution that comes packed with features to simplify project tasks and boost efficiency. This library offers an easy-to-use API, allowing software developers to seamlessly add project management functions to their Java apps. With a few lines of code, java developers can read project data, update task information, and generate reports effortlessly. The Java API stands out for its skill in managing complicated project setups and fine points. It allows developers like you to carefully adjust tasks, resources, and dependencies, keeping your project data precise and current. This precise control is vital for apps requiring great accuracy in project planning and completion.
Aspose.Tasks for Java is a strong tool created for Java programmers to easily handle Microsoft Project files. With this library, you can effortlessly manage project data like creating, reading, updating, or converting project files. It’s a valuable resource for Java developers working in project management. The library supports various Microsoft Project file formats such as MPP, XML, and MPX. Moreover, the tool includes advanced functions like task scheduling, resource distribution, and creating Gantt charts. With these features, developers can build project management solutions that are lively and engaging, tailored to meet their users’ exact requirements. Ultimately, Aspose.Tasks stands out as a revolutionary tool for Java developers working on project management apps. Its wide range of features, ability to work with different file types, and user-friendly API make it a dependable option for anyone looking to boost project management effectiveness.
Getting Started with Aspose.Tasks for Java
The recommend way to install Aspose.Tasks for Java is via Maven repository. You can easily use Aspose.Words for Java API directly in your Maven Projects with simple configurations.
Aspose.Tasks for Java Maven Dependency
//Define the Aspose.Tasks for Java API dependency in your pom.xml as follows
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-tasks</artifactId>
<version>20.10</version>
<classifier>jdk18</classifier>
</dependency>
</dependencies>
You can download the directly from Aspose.Tasks Release pageCreate a New Project File via Java
Software Developers can create new empty projects from scratch using Aspose.Tasks for Java. This is useful when you need to generate project files dynamically. The Library supports a wide range of Microsoft Project file formats, including MPP, XML, and MPX. At present, Aspose.Tasks for Java provides the facility to create XML project files only. The following lines of code shows how software developers can create a simple project file in XML format.
How to Generate an Empty Project inside Java Applications?
public class CreateEmptyProjectFile {
public static void main(String[] args) {
// ExStart: CreateEmptyProjectFile
// For complete examples and data files, please go to https://github.com/aspose-tasks/Aspose.Tasks-for-Java
// The path to the documents directory.
String dataDir = Utils.getDataDir(CreateEmptyProjectFile.class);
// Create a project instance
Project newProject = new Project();
newProject.save(dataDir + "project1.xml", SaveFileFormat.Xml);
//Display result of conversion.
System.out.println("Project file generated Successfully");
// ExEnd: CreateEmptyProjectFile
}
}
Reading Project Files via Java API
One of the fundamental features of Aspose.Tasks for Java is the ability to read Microsoft Project files. The library allows software developers to load an existing project file with just a couple of lines of code and extract valuable information from project files. The Project class can be used to achieve this tasks, you need to provide path to MPP or XML document and returns a Project object which can be used to manipulate project data. The following example shows, how software developers can read a project file online inside Java applications.
How to Read Project File Online Using Java API?
String sharepointDomainAddress = "https://contoso.sharepoint.com";
String userName = "admin@contoso.onmicrosoft.com";
String password = "MyPassword";
ProjectServerCredentials credentials = new ProjectServerCredentials(sharepointDomainAddress, userName, password);
ProjectServerManager reader = new ProjectServerManager(credentials);
for (ProjectInfo p : (Iterable)reader.getProjectList())
{
System.out.println("Project Name:" + p.getName());
System.out.println("Project Created Date:" + p.getCreatedDate());
System.out.println("Project Last Saved Date:" + p.getLastSavedDate());
}
for (ProjectInfo p : (Iterable)reader.getProjectList())
{
Project project = reader.getProject(p.getId());
System.out.println("Project " + p.getName() + " loaded.");
System.out.println("Resources count:" + project.getResources().size());
}
Task Handling & Scheduling in Java Apps
Aspose.Tasks for Java supports create mew tasks, task scheduling and allows software developers to manage task dependencies effectively. It enables developers to modify project data effortlessly. You can update tasks, resources, and other details programmatically. There are several important features part of the library that allows users to handle project tasks, such as adding new tasks, getting and setting tasks properties, associating calendars with particular tasks, manage task duration in projects, managing critical and effort-driven tasks, and many more. The following examples increases and decreases the task duration to 1 week and half week respectively.
How to Manage Project’s Task Duration inside java Applications?
// Create a new project and add a new task
Project project = new Project();
Task task = project.getRootTask().getChildren().add("Task");
// Task duration in days (default time unit)
Duration duration = task.get(Tsk.DURATION);
System.out.println("Duration equals 1 day:" + duration.toString().equals("1 day"));
// Convert to hours time unit
duration = duration.convert(TimeUnitType.Hour);
System.out.println("Duration equals 8 hrs: "+ duration.toString().equals("8 hrs"));
// Increase task duration to 1 week and display if duration is updated successfully
task.set(Tsk.DURATION, project.getDuration(1, TimeUnitType.Week));
System.out.println("Duration equals 1 wk: " + task.get(Tsk.DURATION).toString().equals("1 wk"));
// Decrease task duration and display if duration is updated successfully
task.set(Tsk.DURATION, task.get(Tsk.DURATION).subtract(0.5));
System.out.println("Duration equals 0.5 wks: " + task.get(Tsk.DURATION).toString().equals("0.5 wks"));
Project Data Conversion to PDF via Java API
Aspose.Tasks for Java makes it easy for software developers to load and save project data to various important file formats inside Java applications. The Project class exposes the Save method which can be used to save a project in various formats. The Save method allows users to load and render project data to PDF file format with just a couple of lines of Java code. The following example demonstrates how to Render project data to PDF using Java commands.
How to Export Project Data to PDF inside Java Applications?
// The path to the documents directory.
String dataDir = Utils.getDataDir(SaveAsPdf.class);
// Read the input Project file
Project project = new Project(dataDir + "project6.mpp");
project.save(dataDir + "Project5.pdf", SaveFileFormat.PDF);
// Fitting contents to cell size
Project project1 = new Project(dataDir + "project6.mpp");
SaveOptions o = new PdfSaveOptions();
// Set the LegendOnEachPage property to false to hide legends
// Set the row height to fit cell content
o.setFitContent(true);
o.setTimescale(Timescale.Months);
o.setPresentationFormat(PresentationFormat.TaskUsage);
project1.save("result_months.pdf", o);
o.setLegendOnEachPage(false);
project1.save(dataDir + "result_months_WithoutLegend.pdf", o);
// Display result of conversion.
System.out.println("Process completed Successfully");