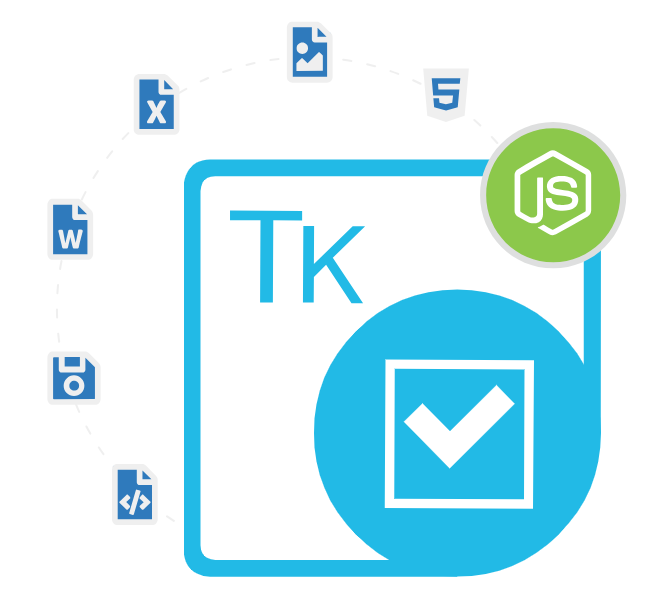
Node.js Project Management API
Node.js Project Management API to Generate & Convert Project Files
Leading Project Management REST SDK allows Developers to Create Project Management Solutions for Creating, Editing, Reading, Converting Project File to PDF, Generating Reports and so on.
As software developers look for more efficient ways to build project management applications, the Aspose.Tasks Cloud Node.js SDK emerges as a robust tool. It is a specialized cloud API designed for project management automation. By offering developers control over project files like Microsoft Microsoft Project (MPP, MPT, MPX) and Primavera P6 (XML, XER), the SDK allows software developers to create, edit, read, view, and manage project data from your Node.js applications. There are several important features part of the API, such as read and write Microsoft Project (MPP) files, create and manage tasks within a project, create and assign resources such as team members, equipment, or materials, create and assign resources to task, track resource usage, add and manage baselines, create custom calendars, generate Gantt charts, export Gantt Chart to PDF and many more.
Aspose.Tasks Cloud Node.js SDK is a part of the Aspose.Tasks Cloud suite, designed to interact with Microsoft Project files and project data via cloud-based APIs. It empowers software developers to programmatically create, modify, manipulate, convert, and analyze project files without the need for Microsoft Project software on the client-side. The SDK offers seamless integration with any Node.js-based application, making it a perfect solution for cloud-driven project management. With just a couple of lines of code software developers can automate tedious and time-consuming tasks, such as generating reports, analyzing project data, and creating Gantt charts inside their own applications. Furthermore, the SDK offers comprehensive documentation and code samples, making it easy for developers to get started with integrating this SDK into their projects. Aspose.Tasks is a game-changer for software developers looking to enhance their app development process. By harnessing the power of this innovative SDK, software developers can unlock new possibilities, streamline their workflow, and deliver exceptional results to their clients and users.
Getting Started with Aspose.Tasks Cloud Node.js SDK
The recommend way to install Aspose.Tasks Cloud Node.js SDK is via NPM. Please use the following commands for a smooth installation.
Install Aspose.Tasks Cloud Node.js SDK via NPM
npm i @asposecloud/aspose-tasks-cloud
You can download the directly from Aspose.Tasks Release pageCreate and Manipulate Project Files
Aspose.Tasks Cloud Node.js SDK allows software developers to create, modify, and save project files in various formats, Microsoft Project (MPP, MPT, MPX) and Primavera P6 (XML, XER). This feature is essential for developing project management tools that need to automate scheduling, task management, and resource allocation. Here is an example that demonstrates how software developers can create a new Microsoft Project (MPP) file. You can also use this API to load and modify existing project files.
How to Create a New Projects inside Node.js Applications?
const { TasksApi, CreateNewProjectRequest } = require("asposetaskscloud");
const tasksApi = new TasksApi(process.env.CLIENT_ID, process.env.CLIENT_SECRET);
const createProjectRequest = new CreateNewProjectRequest("MyNewProject.mpp");
tasksApi.createNewProject(createProjectRequest)
.then(response => {
console.log("Project created successfully:", response);
})
.catch(error => {
console.error("Error creating project:", error);
});
MS Project File Format Conversion
Aspose.Tasks Cloud Node.js SDK has provided the ability to load and convert project files into various file formats, including PDF, HTML, and images. This is particularly useful when sharing project details with non-technical stakeholders or exporting reports. With just a couple of lines of code the API supports converting cloud project documents (MPP, MPT, MPX, XML, XER) to various other file formats, such as HTML, BMP, JPEG, PNG, SVG, TIFF, TXT, CSV, XLSX, XPS, and PDF. Here is an example that shows how software developers can load and convert Project File to PDF format inside Node.js applications.
How to Convert Project File to PDF using Node.js REST API?
const tasksApi = new TasksApi("MY_CLIENT_ID", "MY_CLIENT_SECRET");
const request: GetReportPdfRequest = { name: "template.mpp", folder: "documents", storage: "", type: ReportType.Milestones}
tasksApi.getReportPdf(request)
.then((result) => {
// Deal with a result
console.log(result.response.statusCode);
console.log(result.body.buffer);
})
.catch(function(err) {
// Deal with an error
console.log(err.reponse.statusCode);
console.log(err.body);
});
Project Task Management in Node.js
Aspose.Tasks Cloud Node.js SDK provides robust support for project task management, allowing software developers to create, read, update, and retrieve tasks inside Node.js applications. You can define task start dates, end dates, durations, priorities, and dependencies between tasks. This flexibility enables developers to manage and automate task handling in their projects. Here is an example that shows, how software developers can add or retrieve project tasks inside Node.js applications.
How to Add and Retrieve Project Tasks inside Node.js?
const taskItem = new TaskItem();
taskItem.name = "Develop Backend API";
taskItem.startDate = "2024-10-01T00:00:00";
taskItem.duration = "3d"; // Duration of 3 days
tasksApi.createTask(projectName, taskItem)
.then(response => {
console.log('Task added:', response.task);
return tasksApi.getTasks(projectName);
})
.then(tasks => {
console.log('Tasks retrieved:', tasks.tasks);
})
.catch(error => {
console.error('Error handling tasks:', error);
});
Calendar Management inside Project via Node.js
Project calendars are essential for defining working and non-working days, resource availability, and project milestones. The Aspose.Tasks Cloud Node.js SDK makes it easy for software developers to manage multiple calendars within a project, ensuring that project schedules align with actual working hours and holidays. The following code example demonstrates how to create a project calendar. Calendars can also be edited and assigned to tasks and resources to ensure accurate scheduling.
How to Create a Project Calendar inside Node.js Apps?
const calendarItem = new CalendarItem();
calendarItem.name = "Project Calendar";
calendarItem.isBaseCalendar = true;
tasksApi.createCalendar(projectName, calendarItem)
.then(response => {
console.log('Calendar created:', response.calendar);
})
.catch(error => {
console.error('Error creating calendar:', error);
});