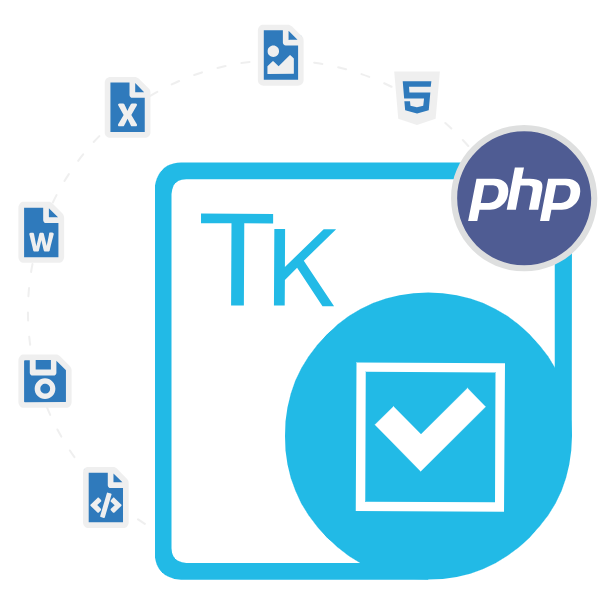
Aspose.Tasks Cloud PHP SDK
PHP API to Create, Manage & Convert Microsoft Project Files
A Powerful PHP Library enables Developers to Work with Microsoft Project MPP, MPX, & XML Files. It enables Developers to Create, Manage, Manipulate, and Convert Microsoft Project Data to PDF, XPS, Images & more.
Project management is at the heart of many business operations, and efficient management of tasks, resources, and schedules is critical to success. Aspose.Tasks Cloud PHP SDK offers a robust solution for software developers looking to integrate powerful project management features into their PHP applications. This SDK enables the seamless creation, manipulation, and conversion of Microsoft Project files in the cloud, eliminating the need for complex setups and heavy local processing.
Aspose.Tasks Cloud PHP SDK is a powerful cloud-based API that enables software developers to work with Microsoft Project files (MPP) programmatically. With the PHP SDK, you can easily integrate project management capabilities into your PHP applications, allowing you to work with project files with ease. There are several important features part of the library, such as read and write Microsoft Project files (MPP) in PHP, import and export project data, manage tasks and task dependencies, assign resources to tasks, generate reports from project data and much more.
Aspose.Tasks Cloud PHP SDK is easy to handle and facilitates collaboration by allowing multiple users to access and update project data concurrently. One of the standout features of the SDK is its ability to convert project files into various formats, including PDF, XLSX, CSV, and HTML. It includes advanced scheduling features, allowing developers to work with calendars and recurrences. With the SDK, you can streamline your project workflows, improve collaboration, and ensure that your projects stay on track, all within a secure and scalable environment.
Getting Started with Aspose.Tasks Cloud PHP SDK
The recommend way to install Aspose.Tasks Cloud PHP SDK is via Composer. Please use the following commands for a smooth installation.
Install Aspose.Tasks Cloud PHP SDK via Composer
composer require aspose/tasks-sdk-php
You can download the directly from Aspose.Tasks Release pageCreate & Manage Project File via PHP
Aspose.Tasks Cloud PHP SDK allows software developers to create and manage Microsoft Project files (MPP, MPX) and Primavera files (XER) effortlessly inside PHP applications. Software developers can create new project files, update existing ones, and extract valuable data, such as tasks, resources, and assignments. This is a very useful feature and can greatly help those businesses that manage multiple projects and need to keep their schedules and resources synchronized. The following code example show, how software developers can creates a new project file inside PHP applications.
How to Create a New Project File inside PHP Apps?
require_once('vendor/autoload.php');
use Aspose\Tasks\TasksApi;
use Aspose\Tasks\Model\Requests\CreateNewProjectRequest;
use Aspose\Tasks\Model\Project;
$tasksApi = new TasksApi('Your Client Id', 'Your Client Secret');
try {
$request = new CreateNewProjectRequest();
$request->setName('NewProject.mpp');
$project = $tasksApi->createNewProject($request);
echo "Project created successfully with ID: " . $project->getProjectId();
} catch (Exception $e) {
echo "Error occurred: " . $e->getMessage();
}
Project File Export to PDF & Other Formats
One of the standout features of the Aspose.Tasks Cloud PHP SDK is its ability to export project files into various formats with just a couple of lines of code inside PHP appications. The API supports conversion to some popular formats, such as PDF, XLSX, CSV, HTML, XML, TXT, TIF, SVG, PNG, JPEG & so on. This flexibility ensures that project data can be easily shared across different platforms and stakeholders. For instance, you can generate a PDF report of your project timeline to present to clients or export the data to an Excel file for further analysis. Here is a simple example that demonstrates, how software developers can convert MS Project MPP file to PDF inside PHP applications.
How to MS Export Project MPP File to PDF via PHP REST API?
// Get your ClientId and ClientSecret from https://dashboard.aspose.cloud (free registration required).
$config = new Configuration();
$config->setAppSid("MY_CLIENT_ID");
$config->setAppKey("MY_CLIENT_SECRET");
$api = new TasksApi(null, $config);
// upload file to cloud
$fullName = 'template.mpp';
$upload_result = $api->uploadFile($Path = realpath(__DIR__ . '/../../..') . '/TestData/' . $fullName, $fullName);
// save as pdf file
$request = new Requests\GetReportPdfRequest($fullName, Model\ReportType::MILESTONES, self::$storageName, $folder));
$result = $api->getReportPdf($request);
Scheduling and Calendars Support
Aspose.Tasks Cloud PHP SDK has included complete support for advanced scheduling features, allowing software developers to work with calendars and recurrences inside their PHP applications. You can define work hours, set up holidays, and manage resource calendars to reflect the actual working conditions. The SDK also supports automatic calculation of task schedules based on dependencies, making it easier to adapt to changes in project timelines.
Task and Resource Management
Aspose.Tasks Cloud PHP SDK makes it easy for software professionals to Manage tasks and resources within a project using PHP REST API. It supports CRUD (Create, Read, Update, Delete) operations on tasks, task links, and resources. You can add new tasks, update existing ones, assign resources, and even manage dependencies between tasks. The SDK also enables you to track task progress and set constraints, ensuring that your project timelines are always under control. The following example shows how software developers can add a new task to existing project using PHP code.
How to Add a New Task to Existing Project inside PHP Apps?
// For complete examples and data files, please go to https://github.com/aspose-tasks-cloud/aspose-tasks-cloud-php/
$remoteName = "AddTask.mpp";
$folder = $this->uploadFile("Project2016.mpp", $remoteName, '');
$response = $this->tasks->postTask(new Requests\PostTaskRequest($remoteName, "New task name", 4, null, self::$storageName, $folder));
Assert::assertEquals(201, $response->getCode());
Assert::assertNotNull($response->getTaskItem());
$newTaskUid = $response->getTaskItem()->getUid();
$response = $this->tasks->getTask(new Requests\GetTaskRequest($remoteName, $newTaskUid, self::$storageName, $folder));
Assert::assertEquals(200, $response->getCode());
Assert::assertNotNull($response->getTask());