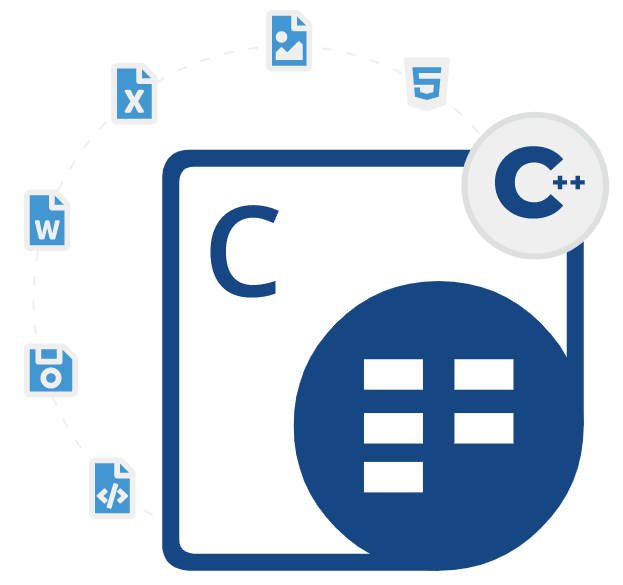
Aspose.Cells for C++
C++ API to Generate & Export Excel Spreadsheets
Read, Write, Edit, Render, Manipulate, Print and Convert Excel spreadsheet files to PDF, HTML, Images and other File Formats without using Microsoft Excel using C++ API.
What is Aspose.Cells for C++?
Aspose.Cells for C++ is a powerful C++ Spreadsheet API designed for developers who need to create Excel spreadsheets, edit existing files, or automate Excel-related tasks without relying on Microsoft Office. This native C++ library supports a wide range of spreadsheet formats including XLS, XLSX, XLSM, XLSB, ODS, PDF, HTML, and CSV. Whether you're working with legacy documents or modern Excel formats, Aspose.Cells for C++ offers seamless integration and compatibility. The library provides robust features for data validation, formula calculation, chart creation, pivot table management, and styling workbooks—making it ideal for developing both 32-bit and 64-bit applications in C++ environments like Microsoft Visual Studio.
With Aspose.Cells for C++, developers can effortlessly generate Excel spreadsheets, convert Excel files to multiple output formats such as PDF, XPS, HTML, ODS, and popular image types like PNG, JPEG, BMP, SVG, and TIFF. This flexibility makes it easy to render Excel files into shareable, read-only formats—perfect for reports, invoices, or documentation. The API also supports advanced capabilities such as copying themes, grouping data, and customizing visual elements, allowing C++ developers to deliver rich spreadsheet functionality with minimal effort. Whether your goal is to automate reporting or build a custom spreadsheet processing solution, Aspose.Cells for C++ offers everything you need in a high-performance, standalone Excel library.
Getting Started with Aspose.Cells for C++
The recommend way to install Aspose.Cells for C++ is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Cells for C++ via NuGet
NuGet\Install-Package Aspose.Cells.Cpp -Version 23.1.0
You can also download it directly from Aspose product release page.Convert Excel Workbook to PDF & Other Formats via C++
Aspose.Cells for C++ has included support for Excel spreadsheet files conversion to various other supported file formats inside C++ applications with just two lines of code. The library has provided conversion from various popular file formats such as XLSM, XLTX, XLTM, XLAM, PDF, XPS, and image (PEG, PNG, BMP, TIFF, GIF, EMF, SVG) file formats. The library has provided various functions for converting Excel workbook to PDF and other file formats, such as directly using IWorkbook class' Save method, the advanced IPdfSaveOptions class or using the get or set methods at the document creation time.
How to Convert Excel Workbook to PDF via .NET API?
StringPtr srcDir = new String("..\\Data\\01_SourceDirectory\\");
// Output directory path.
StringPtr outDir = new String("..\\Data\\02_OutputDirectory\\");
// Path of input Excel file
StringPtr sampleConvertExcelWorkbookToPDF = srcDir->StringAppend(new String("sampleConvertExcelWorkbookToPDF.xlsx"));
// Path of output Pdf file
StringPtr outputConvertExcelWorkbookToPDF = outDir->StringAppend(new String("outputConvertExcelWorkbookToPDF_DirectConversion.pdf"));
// Load the sample Excel file.
intrusive_ptr workbook = Factory::CreateIWorkbook(sampleConvertExcelWorkbookToPDF);
// Save the Excel Document in PDF format
workbook->Save(outputConvertExcelWorkbookToPDF, SaveFormat_Pdf);
Open & Load Different File Formats via C++ API
Aspose.Cells for C++ enables software developers to protect or unprotect their spreadsheets documents inside their .NET applications. The library has included several important functions for protecting their spreadsheet files and data inside it, such as prevent others from accessing data in Excel files by applying password, Protect and unprotect workbook or worksheet, add a digital signature and many more .The library also supports preventing viewing hidden worksheets, adding, moving, deleting, or hiding worksheets, and renaming worksheets.
How to Password Protect or Unprotect Shared Workbook via .NET API?
Workbook wb = new Workbook();
//Protect the Shared Workbook with Password
wb.ProtectSharedWorkbook("1234");
//Uncomment this line to Unprotect the Shared Workbook
//wb.UnprotectSharedWorkbook("1234");
//Save the output Excel file
wb.Save("outputProtectSharedWorkbook.xlsx");
Create and Manage Charts in Spreadsheet via C++ API
Aspose.Cells for C++ allows computer programmers to insert and manage charts inside Excel spreadsheet files using C++ commands. The library allows developers to visualize information in charts same like Microsoft Excel. It provides support for some common charts types supported by MS-Excel and other leading spreadsheet applications, such as pyramid chart, line chart, bubble chart and many more. Developers can also render Excel charts to images and PDF formats without needing any additional tools or applications. The library also allows to read and process Microsoft Excel 2016 charts which are not available in Microsoft Excel 2013 or prior versions.
How to Add Pyramid Chart to Excel Worksheet via C++ API?
// Output directory path
StringPtr outDir = new String("..\\Data\\02_OutputDirectory\\");
// Path of output excel file
StringPtr outputChartTypePyramid = outDir->StringAppend(new String("outputChartTypePyramid.xlsx"));
// Create a new workbook
intrusive_ptr workbook = Factory::CreateIWorkbook();
// Get first worksheet which is created by default
intrusive_ptr worksheet = workbook->GetIWorksheets()->GetObjectByIndex(0);
// Adding sample values to cells
worksheet->GetICells()->GetObjectByIndex(new String("A1"))->PutValue(50);
worksheet->GetICells()->GetObjectByIndex(new String("A2"))->PutValue(100);
worksheet->GetICells()->GetObjectByIndex(new String("A3"))->PutValue(150);
worksheet->GetICells()->GetObjectByIndex(new String("B1"))->PutValue(4);
worksheet->GetICells()->GetObjectByIndex(new String("B2"))->PutValue(20);
worksheet->GetICells()->GetObjectByIndex(new String("B3"))->PutValue(50);
// Adding a chart to the worksheet
int chartIndex = worksheet->GetICharts()->Add(Aspose::Cells::Charts::ChartType::ChartType_Pyramid, 5, 0, 20, 8);
// Accessing the instance of the newly added chart
intrusive_ptr chart = worksheet->GetICharts()->GetObjectByIndex(chartIndex);
// Adding SeriesCollection (chart data source) to the chart ranging from "A1" cell to "B3"
chart->GetNISeries()->Add(new String("A1:B3"), true);
// Saving the Excel file
workbook->Save(outputChartTypePyramid);
Add Hyperlinks & Manipulate Data in Excel Files via C++ API
Aspose.Cells for C++ has included several important features for handling data inside Excel spreadsheet files using C++ API. The library allows to access data in spreadsheet cell, adding and retrieve data from cells, rows or columns, inserting hyperlinks to a cell with ease. It also possible to apply conditional formatting in worksheet and create named range in an Excel Workbook. The library also facilitates developers to search data, manipulate named range in a workbook, apply style and formatting, precedents or dependents and so on.
How to Add Hyperlinks to Excel Cell via C++ API?
/Path of output excel file
StringPtr outputAddHyperlinksToTheCells = outPath->StringAppend(new String("outputAddHyperlinksToTheCells.xlsx"));
//Create a new workbook
intrusive_ptr workbook = Factory::CreateIWorkbook();
//Get the first worksheet
intrusive_ptr wsc = workbook->GetIWorksheets();
intrusive_ptr ws = wsc->GetObjectByIndex(0);
//Add hyperlink in cell C7 and make use of its various methods
intrusive_ptr hypLnks = ws->GetIHyperlinks();
int idx = hypLnks->Add(new String("C7"), 1, 1, new String("http://www.aspose.com/"));
intrusive_ptr lnk = hypLnks->GetObjectByIndex(idx);
lnk->SetTextToDisplay(new String("Aspose"));
lnk->SetScreenTip(new String("Link to Aspose Website"));
//Save the workbook
workbook->Save(outputAddHyperlinksToTheCells);