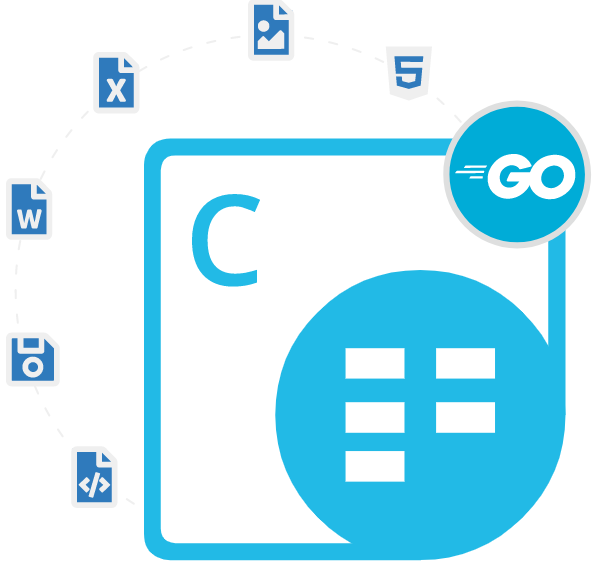
Aspose.Cells Cloud SDK for Go
Go API to Create & Convert Excel Spreadsheets
Create, modify, manipulate, print, parse, split, merge & convert Excel Spreadsheet documents in the cloud using Go Excel library.
Aspose.Cells Cloud SDK for Go is a powerful and reliable cloud-based solution that allows software developers to work with Excel files in the cloud. One of the main benefits of using the Aspose.Cells Cloud SDK for Go is that it is cloud-based. This means that you can easily access your Excel files from anywhere and on any device. So users don't need to install any software or worry about file compatibility issues and everything is safely handled in the cloud. The SDK can be used to develop software applications for a wide range of operating systems, such as Windows, Linux, Mac OS, and many others.
Aspose.Cells Cloud SDK for Go has included a rich set of features that can help software developers in automating many Excel-related tasks, such as performing complex calculations, formatting cells, adding charts, and much more. It is very easy to handle and has provided support for some popular Microsoft Excel file formats such as XLS, XLSX, XLSB, XLSM, XLT, XLTX, XLTM, ODS, XML, CSV, TSV, TXT (TabDelimited), HTML, MHTML, DIF, PDF, XPS, TIFF, SVG, SXC, FODS and many more. Using Aspose.Cells Cloud SDK software developers can protect their Excel files with a password as well as unprotect files that have been password protected.
Aspose.Cells Cloud SDK for Go is very easy to handle and has included several advanced features enabling software developers to manipulate Microsoft Excel spreadsheets, such as applying auto filtering, pivot tables handling, managing conditional formatting, converting ListObject or table to a range of cells, manage rows and cells, search and replace text in Excel Worksheet, adding a background in the workbook, inserting shape on a worksheet, add a pivot table on an Excel worksheet, hide rows on an Excel worksheet, auto-fit columns on the workbook and many more.
Getting Started with Aspose.Cells Cloud SDK for Go
The recommend way to install Aspose.Cells Cloud SDK for Go is using GitHub. Please use the following command for a smooth installation.
Install Aspose.Cells Cloud SDK for Go via GitHub
go get -u github.com/aspose-cells-cloud/aspose-cells-cloud-go/cellsapi
You can also download it directly from Aspose product release page.Protect Excel File via Go API
Aspose.Cells Cloud Go SDK has included a very useful feature allows software developers to protect Excel Spreadsheet from unauthorized access inside their own Go applications. The library has included various features to protecting Excel spreadsheets, such as add digital signature for Excel workbook, protect Excel files without using storage, encrypt Excel workbooks, decrypt Excel workbooks, unprotect Excel workbooks, unlock Excel files without using storage and so on. The following example shows how software developers can add digital signature to their Excel workbook inside Go applications.
Add Digital Signature for Excel Workbook via GO
package main
import (
"os"
asposecellscloud "github.com/aspose-cells-cloud/aspose-cells-cloud-go/v22"
)
func main() {
instance := asposecellscloud.NewCellsApiService(os.Getenv("ProductClientId"), os.Getenv("ProductClientSecret"))
uploadOpts := new(asposecellscloud.UploadFileOpts)
uploadOpts.Path = "roywang.pfx"
file, err := os.Open("roywang.pfx")
if err != nil {
return
}
_, _, err = instance.UploadFile(file, uploadOpts)
if err != nil {
return
}
requestOpts := new(asposecellscloud.CellsWorkbookPostDigitalSignatureOpts)
requestOpts.Digitalsignaturefile = "roywang.pfx"
requestOpts.Folder = "CellsTests"
requestOpts.Name = "Book1.xlsx"
requestOpts.Password = "123456"
_, _, err = instance.CellsWorkbookPostDigitalSignature(requestOpts)
if err != nil {
return
}
}
Export Excel Workbook & Internal Objects via Go API
Aspose.Cells Cloud Go SDK has included very powerful features for exporting an Excel workbook and its internal objects to other supported file formats inside Go applications. It allows exporting workbook, list-object, chart, shape, picture and many other objects from excel file to a specific format, such as PDF, OTS, XPS, DIF, PNG, JPEG, BMP, SVG, TIFF, EMF, NUMBERS, FODS and so on. The following examples demonstrates how to export the Excel workbook to PDF format using Go commands.
Export the Excel workbook to PDF format via Go API
outputFile := "Book1.pdf"
pdfSaveOptions := &models.PdfSaveOptions{
OnePagePerSheet: true,
Quality: "Print",
SecurityOptions: &models.PdfSecurityOptions{
// set PDF security options if needed
},
}
exportRequest := &cellsapi.PostWorkbookSaveAsRequest{
Name: "Book1.xlsx",
Newfilename: outputFile,
SaveOptions: pdfSaveOptions,
Folder: "input",
Format: "pdf",
}
_, _, err = cellsApi.PostWorkbookSaveAs(context.Background(), exportRequest)
if err != nil {
fmt.Println("Error:", err)
return
}
Create & Manage Excel Workbook via Go API
Aspose.Cells Cloud Go SDK enables software developers to create and modify Excel workbook without using Microsoft Office Excel or any other application. The library has included various features for working with Excel worksheets, such as generate an empty Excel workbook, Excel workbook creation from a template file, add new worksheet to existing workbook, rename worksheet, insert chart to existing workbook, generate Excel workbook from a smart marker template and many more.
Create a new Excel workbook via Go API
ctx := context.Background()
request := &models.PutWorkbookCreateRequest{
Name: "MyWorkbook.xlsx",
}
response, _, err := cellsApi.PutWorkbookCreate(ctx, request)
if err != nil {
fmt.Println("Error creating workbook:", err)
return
}
fmt.Println("Workbook created successfully:", response.Status)
Create & Manage Rows/Column via Go API
Aspose.Cells Cloud Go SDK makes it easy for software developers to work with Excel worksheets rows and columns using Go commands. The library supports adding empty rows or columns on an Excel worksheet, deleting selected rows or columns from worksheet, copying rows or columns on worksheet, hiding rows or columns on an Excel worksheet, auto-fit rows or columns on an Excel workbook, grouping rows or columns on an Excel Worksheet and many more.
Copy a Row from One Location to Another in a Worksheet using Go SDK
package main
import (
"fmt"
"os"
"github.com/aspose-cells-cloud/aspose-cells-cloud-go/v21.9/api"
"github.com/aspose-cells-cloud/aspose-cells-cloud-go/v21.9/model"
"github.com/aspose-cells-cloud/aspose-cells-cloud-go/v21.9/api/cellsapi"
)
func main() {
// Set up authentication and initialization
configuration := cellsapi.NewConfiguration()
configuration.AppKey = "your_app_key"
configuration.AppSid = "your_app_sid"
cellsAPI := api.NewCellsApiWithConfig(configuration)
// Copy the row from source location to destination location
sourceWorksheet := "Sheet1"
sourceRowIndex := int32(1)
destinationRowIndex := int32(2)
copyOptions := &model.CopyOptions{
ColumnNumber: nil,
DestinationWorksheet: nil,
Range: "",
RowNumber: &destinationRowIndex,
Worksheet: &sourceWorksheet,
}
_, err := cellsAPI.PostWorksheetRows(context.Background(), "test.xlsx", sourceWorksheet, sourceRowIndex, 1, copyOptions)
if err != nil {
fmt.Println("Error: ", err)
os.Exit(1)
}
fmt.Println("Row copied successfully")
}