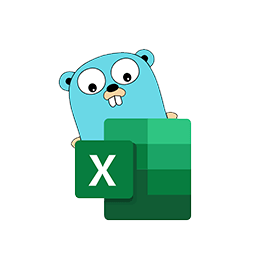
Excelize
Open Source Go Library for Managing Spreadsheets
Read, Create, Merge & Filter Data in Microsoft Excel XLSX / XLSM / XLTM Spreadsheet Files via Open Source Go API.
What is Excelize?
Excelize is an Open source pure Go library that provides functionality for reading and writing Microsoft Excel XLSX / XLSM / XLTM spreadsheet files. Developers can read and write spreadsheet documents generated by Microsoft Excel™ 2007 and later inside their own applications. The library is very user-friendly and supports the reading of large spreadsheet files. It has provided a streaming API for generating or reading data from a worksheet with huge amounts of data.
The library fully supports several important features related to Excel spreadsheet files manipulation and processing, such as reading and writing Excel files, working with cell ranges, Excel rows management, adding comments, merging cells, copying rows, conditional formatting, insert texts, manage tables in Excel files, add images, add new sheets and many more.
Getting Started with Excelize
The recommended way to install Excelize is from GitHub, please use the following command for faster installation.
Install via GitHub Command
go get github.com/360EntSecGroup-Skylar/excelize
Generate Excel XLSX File via Go Library
Excelize enables programmers to generate an Excel XLSX spreadsheet inside their own Go applications. Once you have created the sheet you can also create a new sheet, set the value of a cell, define the number of rows and columns, Set an active sheet of the workbook, and more. You can also assign a name to a sheet and save the file to the path of your choice, set font type and text size for a created sheet.
How to Create Excel XLSX File via Go API?
package main
import (
"fmt"
"github.com/xuri/excelize/v2"
)
func main() {
f := excelize.NewFile()
// Create a new sheet.
index := f.NewSheet("Sheet2")
// Set value of a cell.
f.SetCellValue("Sheet2", "A2", "Hello world.")
f.SetCellValue("Sheet1", "B2", 100)
// Set active sheet of the workbook.
f.SetActiveSheet(index)
// Save spreadsheet by the given path.
if err := f.SaveAs("Book1.xlsx"); err != nil {
fmt.Println(err)
}
}
Insert Chart to an XLSX File
Excel Charts are visual representations of data from a worksheet that can convey more understanding of the data by summarizing large amounts of data within a small section of your worksheet. Charts allow users to visually display data in different formats such as Bar, Column, Pie, Line, Area, or Radar, etc. Excelize library supports chart generation as well as control with just a few lines of code inside your own applications. It allows building charts based on data in a worksheet or generating charts without any data.
How to Add Chart to Excel Spreadsheet File via Go API?
package main
import (
"fmt"
"github.com/xuri/excelize/v2"
)
func main() {
categories := map[string]string{
"A2": "Small", "A3": "Normal", "A4": "Large",
"B1": "Apple", "C1": "Orange", "D1": "Pear"}
values := map[string]int{
"B2": 2, "C2": 3, "D2": 3, "B3": 5, "C3": 2, "D3": 4, "B4": 6, "C4": 7, "D4": 8}
f := excelize.NewFile()
for k, v := range categories {
f.SetCellValue("Sheet1", k, v)
}
for k, v := range values {
f.SetCellValue("Sheet1", k, v)
}
if err := f.AddChart("Sheet1", "E1", `{
"type": "col3DClustered",
"series": [
{
"name": "Sheet1!$A$2",
"categories": "Sheet1!$B$1:$D$1",
"values": "Sheet1!$B$2:$D$2"
},
{
"name": "Sheet1!$A$3",
"categories": "Sheet1!$B$1:$D$1",
"values": "Sheet1!$B$3:$D$3"
},
{
"name": "Sheet1!$A$4",
"categories": "Sheet1!$B$1:$D$1",
"values": "Sheet1!$B$4:$D$4"
}],
"title":
{
"name": "Fruit 3D Clustered Column Chart"
}
}`); err != nil {
fmt.Println(err)
return
}
// Save spreadsheet by the given path.
if err := f.SaveAs("Book1.xlsx"); err != nil {
fmt.Println(err)
}
}
Creating PivotTable in Excel File
Excelize provides the capability for creating a pivot table inside an Excel spreadsheet with ease. Pivot tables are one of the most powerful features of Microsoft Excel. It is a powerful tool to calculate, summarize, and analyze your data in an Excel sheet. You can create a simple pivot table by using the AddPivotTable function. Moreover, you can easily assign or edit data source to a pivot table.
How to Generate PivotTable in Excel File inside Go Apps?
func main() {
f := excelize.NewFile()
// Create some data in a sheet
month := []string{"Jan", "Feb", "Mar", "Apr", "May",
"Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"}
year := []int{2017, 2018, 2019}
types := []string{"Meat", "Dairy", "Beverages", "Produce"}
region := []string{"East", "West", "North", "South"}
f.SetSheetRow("Sheet1", "A1", &[]string{"Month", "Year", "Type", "Sales", "Region"})
for row := 2; row < 32; row++ {
f.SetCellValue("Sheet1", fmt.Sprintf("A%d", row), month[rand.Intn(12)])
f.SetCellValue("Sheet1", fmt.Sprintf("B%d", row), year[rand.Intn(3)])
f.SetCellValue("Sheet1", fmt.Sprintf("C%d", row), types[rand.Intn(4)])
f.SetCellValue("Sheet1", fmt.Sprintf("D%d", row), rand.Intn(5000))
f.SetCellValue("Sheet1", fmt.Sprintf("E%d", row), region[rand.Intn(4)])
}
if err := f.AddPivotTable(&excelize.PivotTableOptions{
DataRange: "Sheet1!$A$1:$E$31",
PivotTableRange: "Sheet1!$G$2:$M$34",
Rows: []excelize.PivotTableField{
{Data: "Month", DefaultSubtotal: true}, {Data: "Year"}},
Filter: []excelize.PivotTableField{
{Data: "Region"}},
Columns: []excelize.PivotTableField{
{Data: "Type", DefaultSubtotal: true}},
Data: []excelize.PivotTableField{
{Data: "Sales", Name: "Summarize", Subtotal: "Sum"}},
RowGrandTotals: true,
ColGrandTotals: true,
ShowDrill: true,
ShowRowHeaders: true,
ShowColHeaders: true,
ShowLastColumn: true,
}); err != nil {
fmt.Println(err)
}
if err := f.SaveAs("Book1.xlsx"); err != nil {
fmt.Println(err)
}
}
Add Images to XLSX Spreadsheets
The Excelize library provides functionality for adding images to an Excel XLSX spreadsheet with just a couple of lines of Go commands. You can also modify images according to your needs. You can insert a picture with scaling as well as with printing support.
How to Add Image to Excel Worksheet File via Go API?
package main
import (
"fmt"
_ "image/gif"
_ "image/jpeg"
_ "image/png"
"github.com/xuri/excelize/v2"
)
func main() {
f, err := excelize.OpenFile("Book1.xlsx")
if err != nil {
fmt.Println(err)
return
}
defer func() {
// Close the spreadsheet.
if err := f.Close(); err != nil {
fmt.Println(err)
}
}()
// Insert a picture.
if err := f.AddPicture("Sheet1", "A2", "image.png", ""); err != nil {
fmt.Println(err)
}
// Insert a picture to worksheet with scaling.
if err := f.AddPicture("Sheet1", "D2", "image.jpg",
`{"x_scale": 0.5, "y_scale": 0.5}`); err != nil {
fmt.Println(err)
}
// Insert a picture offset in the cell with printing support.
if err := f.AddPicture("Sheet1", "H2", "image.gif", `{
"x_offset": 15,
"y_offset": 10,
"print_obj": true,
"lock_aspect_ratio": false,
"locked": false
}`); err != nil {
fmt.Println(err)
}
// Save the spreadsheet with the origin path.
if err = f.Save(); err != nil {
fmt.Println(err)
}
}