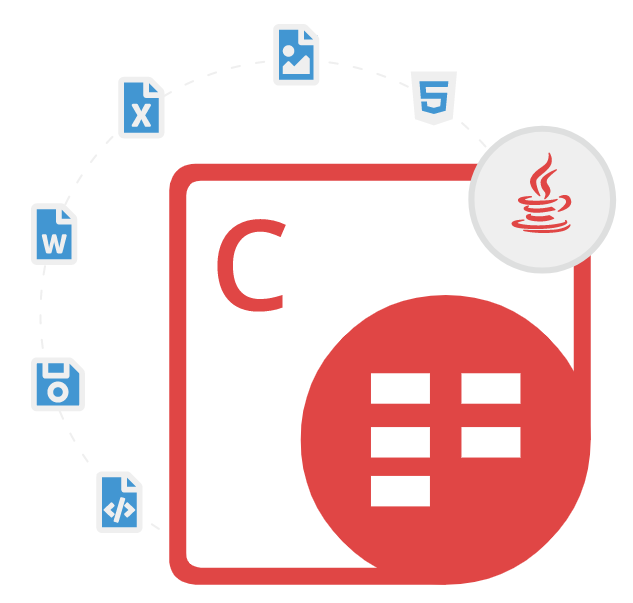
Aspose.Cells for Java
Java API to Generate & Process Excel Spreadsheets
A Pure Java Class Library allows Developers to Read, Write, Edit, Render, Manipulate, Print and Convert Excel Spreadsheet Files without using Microsoft Excel.
What is Aspose.Cells for Java?
Aspose.Cells for Java is a very powerful and pure Java class library for working with Excel spreadsheets and other popular file formats without installing Microsoft Excel or any third-party application. The library can be used to create various types of applications such as Java Web Applications or Desktop Applications. Aspose.Cells for Java can read and write spreadsheet files in a variety of formats, including Microsoft Excel (XLS, XLSX, XLSM, XLSB), OpenDocument Format (ODS), PDF, HTML, CSV, and many more.
Aspose.Cells for Java allows developers to create and evaluate complex formulas in spreadsheets, including support for external references, named ranges, and custom functions. The library is very feature rich and has included several important features for working with spreadsheets documents such as opening and reading files with different formats, adding new worksheets, merging existing worksheets, merging different workbooks, encrypting and decrypting workbooks and worksheets, printing and previewing workbooks, render spreadsheets, manage rows and columns, apply formulas and many more.
Aspose.Cells for Java easily import data from a variety of sources, including databases, CSV files, and other spreadsheet formats. Additionally, data can be exported to a variety of formats, including PDF, HTML, and CSV. The library allows applying a wide range of formatting options to cells, including font size and style, background color, borders, and more. It also supports handling worksheets and charts within a spreadsheet, including adding, deleting, and moving worksheets, and adding, editing, and formatting chart elements.
Getting Started with Aspose.Cells for Java
Maven is the easiest way to download and install Aspose.Cells for Java. First, you need to specify Aspose Maven Repository configuration/location in your Maven pom.xml as below:
Maven repository for Aspose.Cells for Java
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
//Define Aspose.Cells for Java API Dependency
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-cells</artifactId>
<version>23.1</version>
</dependency>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-cells</artifactId>
<version>23.1</version>
<classifier>javadoc</classifier>
</dependency>
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.60</version>
</dependency>
</dependencies>
You can also download it directly from Aspose product release page.Create New Excel Spreadsheet via Java API
Aspose.Cells for Java has provided complete functionality for creating new Excel spreadsheets from the scratch with just a couple of line of Java code. You can also open an existing spreadsheet file by providing a path to it or through stream and make some changes to it and save it back with the new changes. The library also supports opening files of different Microsoft Excel versions. It is also possible to dynamically generate Excel file and populate it with data from a database or from any other supported source. You can also apply formatting of your choice to it, add new worksheets, set page size and set document properties like title, author name, company name and son on.
Creating a Workbook via Java API
String dataDir = Utils.getDataDir(CreatingWorkbook.class);
// Creating a file input stream to reference the license file
FileInputStream fstream = new FileInputStream("Aspose.Cells.lic");
// Create a License object
License license = new License();
// Applying the Aspose.Cells license
license.setLicense(fstream);
// Instantiating a Workbook object that represents a Microsoft Excel
// file.
Workbook wb = new Workbook();
// Note when you create a new workbook, a default worksheet, "Sheet1", is by default added to the workbook. Accessing the
// first worksheet in the book ("Sheet1").
Worksheet sheet = wb.getWorksheets().get(0);
// Access cell "A1" in the sheet.
Cell cell = sheet.getCells().get("A1");
// Input the "Hello World!" text into the "A1" cell
cell.setValue("Hello World!");
// Save the Microsoft Excel file.
wb.save(dataDir + "MyBook.xls", FileFormatType.EXCEL_97_TO_2003);
wb.save(dataDir + "MyBook.xlsx");
wb.save(dataDir + "MyBook.ods");
Saving Excel File to Other File Formats via Java
Aspose.Cells for Java has included a great feature for creating Excel files and saving it in various different ways inside Java applications. A developer needs to specify the file format in which their files should be saved, such as PDF, CSV, XLSX, XLSM, XPS, XLTM, XLAM, Excel template file, TSV, HTML, MHTML, ODS, SpreadSheetML, Tagged Image File Format (TIFF), SVG, Data Interchange Format and many more. Let’s support a developer want to save a file to a particular location, he need to provide the file name with complete path and file format. It is also possible to save an entire workbook into text format.
Save Excel File to PDF via Java API
String dataDir = Utils.getSharedDataDir(SaveInPdfFormat.class) + "loading_saving/";
// Creating an Workbook object with an Excel file path
Workbook workbook = new Workbook();
// Save in PDF format
workbook.save(dataDir + "SIPdfFormat_out.pdf", FileFormatType.PDF);
// Print Message
System.out.println("Worksheets are saved successfully.");
Add & Convert Tables in Excel File via Java API
Aspose.Cells for Java has provided complete support for handling tables inside Excel worksheet using Java commands. The library support various important features for working tables such as creating new table, converting an Excel table to a range of data, create table by using border lines for a range, Convert Table to ODS, apply formatting to content inside the table, propagate formulas inside the table, accessing table from Cell and Adding Values inside it and many more.
Convert Table to Range with Options via Java Library
String dataDir = Utils.getSharedDataDir(ConvertTableToRangeWithOptions.class) + "Tables/";
// Open an existing file that contains a table/list object in it
Workbook workbook = new Workbook(dataDir + "book1.xlsx");
TableToRangeOptions options = new TableToRangeOptions();
options.setLastRow(5);
// Convert the first table/list object (from the first worksheet) to normal range
workbook.getWorksheets().get(0).getListObjects().get(0).convertToRange(options);
// Save the file
workbook.save(dataDir + "ConvertTableToRangeWithOptions_out.xlsx");
Extract Images & Text from Excel Worksheet via Java API
Aspose.Cells for Java makes it easy for software engineers to manage images and text inside their Excel spreadsheets using Java code. The library allows software developers to extract images as well as text from Excel file and save them to the place of their choice. The library also supports generating thumbnail image of a worksheet with just a couple of lines of Java code.
Extract Images from Excel File via Java API
String dataDir = Utils.getSharedDataDir(ExtractImagesfromWorksheets.class) + "TechnicalArticles/";
// Open a template Excel file
Workbook workbook = new Workbook(dataDir + "book3.xlsx");
// Get the first worksheet
Worksheet worksheet = workbook.getWorksheets().get(0);
// Get the first Picture in the first worksheet
Picture pic = worksheet.getPictures().get(0);
// Set the output image file path
String fileName = "aspose-logo.jpg";
// Note: you may evaluate the image format before specifying the image path
// Define ImageOrPrintOptions
ImageOrPrintOptions printoption = new ImageOrPrintOptions();
// Specify the image format
printoption.setImageType(ImageType.JPEG);
// Save the image
pic.toImage(dataDir + fileName, printoption);