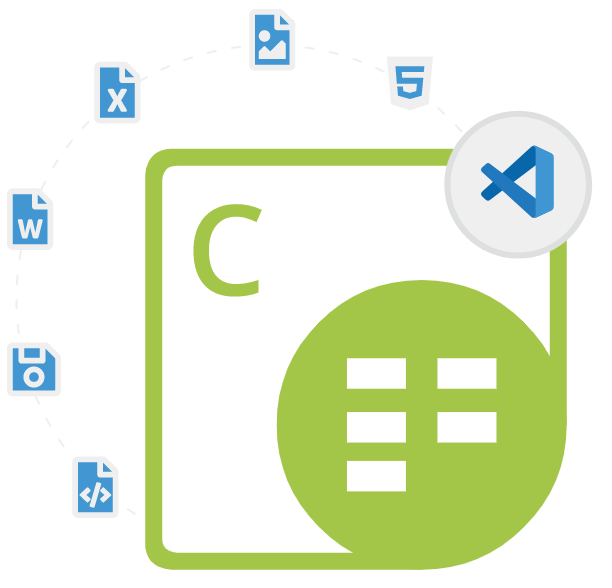
Aspose.Cells for .NET
C# .NET API to Create & Convert Excel Spreadsheets
Advanced Spreadsheets document processing API to create, modify, convert, & render Spreadsheets files without using Microsoft PowerPoint or other third-party software.
Aspose.Cells for .NET is an advanced and feature-rich library that gives software programmers the ability to create and manage Excel spreadsheets without installing Microsoft office or Excel on their machines. The library supports various popular spreadsheet (XLS, XLSX, XLSM, XLSB, XLTX, XLTM, CSV, SpreadsheetML, ODS) file formats your business use every day. Apart from that the library also supports exporting Excel spreadsheets to PDF, DOCX, PPTX, JSON, XPS, HTML, MHTML, JSON, Plain Text, and popular image formats including TIFF, JPG, PNG, BMP, and SVG.
Aspose.Cells for .NET has included numerous features for handling Spreadsheet documents creation and its management inside .NET applications, such as adding a new workbook to an existing spreadsheet file, adding a copy of an existing worksheet, adding images and charts, setting gradient background for charts, creating comments, auto-filters, and page breaks, work with Excel formulas and calculations, creating Pivot Tables, add new workbooks, merge existing workbooks, importing images and charts, importing formula from a designer spreadsheet, and many more.
Aspose.Cells for .NET provides a wide range of additional functionality, including the ability to create and manipulate charts, pivot tables, and named ranges, as well as support for working with data validation, data protection, and conditional formatting. The library can be used with any kind of application whether it’s an ASP.NET web application or a Windows desktop application. With its wide range of features, support for a wide range of file formats, and extensive documentation, Aspose.Cells is an excellent choice for any developer looking to work with Excel files in their .NET applications.
Getting Started with Aspose.Cells for .NET
The recommend way to install Aspose.Cells for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Cells for .NET via NuGet
NuGet\Install-Package Aspose.Cells -Version 23.1.1
You can also download it directly from Aspose product release page.Excel Spreadsheet Creation via C#.NET API
Aspose.Cells for .NET fully supports conversion between numerous file formats. It allows software developers to load Excel Spreadsheets in one file format and save it in numerous other supported file formats inside their .NET applications. The library allows Excel Spreadsheet conversion to PDF, HTML, PowerPoint, XPS, HTML, MHTML, JSON, Plain Text and popular image formats including TIFF, JPG, PNG, BMP and SVG. The library also allows converting Excel workbook to Ods,Sxc and Fods (OpenOffice / LibreOffice calc).
Creating a New Workbook via .NET API
string dataDir = RunExamples.GetDataDir(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
try
{
// Create a License object
License license = new License();
// Set the license of Aspose.Cells to avoid the evaluation limitations
license.SetLicense(dataDir + "Aspose.Cells.lic");
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
// Instantiate a Workbook object that represents Excel file.
Workbook wb = new Workbook();
// When you create a new workbook, a default "Sheet1" is added to the workbook.
Worksheet sheet = wb.Worksheets[0];
// Access the "A1" cell in the sheet.
Cell cell = sheet.Cells["A1"];
// Input the "Hello World!" text into the "A1" cell
cell.PutValue("Hello World!");
// Save the Excel file.
wb.Save(dataDir + "MyBook_out.xlsx");
Protect Excel Spreadsheet via C# .NET API
Aspose.Cells for .NET enables software developers to protect or unprotect their spreadsheets documents inside their .NET applications. The library has included several important functions for protecting their spreadsheet files and data inside it, such as prevent others from accessing data in Excel files by applying password, Protect and unprotect workbook or worksheet, add a digital signature and many more .The library also supports preventing viewing hidden worksheets, adding, moving, deleting, or hiding worksheets, and renaming worksheets.
Password Protect or Unprotect Shared Workbook via .NET API
Workbook wb = new Workbook();
//Protect the Shared Workbook with Password
wb.ProtectSharedWorkbook("1234");
//Uncomment this line to Unprotect the Shared Workbook
//wb.UnprotectSharedWorkbook("1234");
//Save the output Excel file
wb.Save("outputProtectSharedWorkbook.xlsx");
Excel Formulas Calculations Support via C#
Aspose.Cells for .NET has included the ability to work with Excel formulas and calculating results using C#.NET commands. The library has provided a comprehensive set of functions for working with Excel formulas, making it easy to create and manipulate formulas, as well as evaluate and recalculate them. It supports direct calculation of formulas, calculating formulas repeatedly, calculation of Excel 2016 MINIFS and MAXIFS functions, calculation of IFNA function, calculating the array formula of a data table and many more.
Set Simple Formula for Named Range via C# API
string dataDir = RunExamples.GetDataDir(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
// Create an instance of Workbook
Workbook book = new Workbook();
// Get the WorksheetCollection
WorksheetCollection worksheets = book.Worksheets;
// Add a new Named Range with name "NewNamedRange"
int index = worksheets.Names.Add("NewNamedRange");
// Access the newly created Named Range
Name name = worksheets.Names[index];
// Set RefersTo property of the Named Range to a formula. Formula references another cell in the same worksheet
name.RefersTo = "=Sheet1!$A$3";
// Set the formula in the cell A1 to the newly created Named Range
worksheets[0].Cells["A1"].Formula = "NewNamedRange";
// Insert the value in cell A3 which is being referenced in the Named Range
worksheets[0].Cells["A3"].PutValue("This is the value of A3");
// Calculate formulas
book.CalculateFormula();
// Save the result in XLSX format
book.Save(dataDir + "output_out.xlsx");
Combine Multiple Excel File & Workbooks via C# API
It is often required to combine various Excel files or workbooks into a single spreadsheet file. Aspose.Cells for .NET makes it easy for software developers to combine multiple workbooks containing images, charts, text and other data into a single workbook using a couple of lines of .NET code. The library also supports combining multiple worksheets into a single worksheet with ease.
Combine Multiple Workbooks via .NET API
string dataDir = RunExamples.GetDataDir(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
// Define the first source
// Open the first excel file.
Workbook SourceBook1 = new Workbook(dataDir+ "SampleChart.xlsx");
// Define the second source book.
// Open the second excel file.
Workbook SourceBook2 = new Workbook(dataDir+ "SampleImage.xlsx");
// Combining the two workbooks
SourceBook1.Combine(SourceBook2);
dataDir = dataDir + "Combined.out.xlsx";
// Save the target book file.
SourceBook1.Save(dataDir);