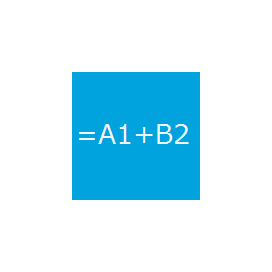
ReoGrid
C# .NET Library to Create & Convert XLSX Spreadsheets
Open Source C# API allows Developers to Create, Read, Modify & Export Microsoft Excel XLSX Spreadsheet, Manage Worksheets, Row and Column Settings and So on.
What is ReoGrid?
A fantastic tool is available for you if you work with software applications and need to handle Excel files effortlessly. This open-source .NET spreadsheet component is super handy. It’s lightweight, so it won’t slow you down, and it can do a lot in a short time, saving you money too. In fact, it’s lightning-fast, performing tasks related to spreadsheets up to 300 times quicker than other similar products out there.
The ReoGrid API is versatile, supporting various platforms like Windows Form and WPF. It comes packed with features essential for creating and managing Excel Spreadsheets. You can do things like starting a new workbook from the ground up, handling worksheets, rows, and columns, setting cell styles, managing borders, working with data formats and cell values, utilizing formulas, incorporating and managing images, using floating objects, including charts or graphs, and many other functions.
ReoGrid has a smart way of handling memory which helps it work more efficiently. It breaks big spreadsheets into smaller sections, which speeds up how it handles memory. Also, it has a clever border algorithm that knows exactly where to draw borders quickly.
Getting Started with ReoGrid
The following steps update the compiler and set the appropriate environment variables.
Install PIP Command
PM> Install-Package unvell.ReoGrid.dll
Excel Workbook Creation via .NET API
The open source library ReoGrid enables software developers to generate a new Excel workbook with just a couple of lines of C# code. The library also allows to add a new worksheet, assign names to worksheets, delete unwanted sheets, move worksheets,s and so on. The library also makes it easy to access worksheets and supports managing cell data, styles, borders, outlines, ranges, formula calculation, etc.
How to Add Worksheet to Workbook via .NET API?
private void btnAddWorksheet_Click(object sender, EventArgs e)
{
// create worksheet
var newSheet = this.grid.CreateWorksheet();
// set worksheet background color
newSheet.SetRangeStyles(RangePosition.EntireRange, GetRandomBackColorStyle());
// add worksheet into workbook
this.grid.AddWorksheet(newSheet);
// set worksheet as current focus
grid.CurrentWorksheet = newSheet;
}
Export Excel XLSX File to CSV & HTML
The open source component ReoGrid enables software developers to export Excel XLSX spreadsheets to other supported file formats inside their own .NET applications. Developers can easily load CSV files, export worksheets as CSV format, export worksheets as HTML or PDF, output spreadsheets to printers,s and so on. It is also possible to select a specified range of a row or grid rather than an entire worksheet and export it to other supported formats. It also supports exporting worksheets to RGF files with just a couple of lines of code.
How to Convert Excel XLSX File to CSV via .NET API?
// load from stream
void LoadCSV(Stream s);
// load from file
void LoadCSV(string path);
// load from stream and convert string by specified encoding
void LoadCSV(Stream s, Encoding encoding);
// load from path and convert string by specified encoding
public void LoadCSV(string path, Encoding encoding);
//Export as CSV
worksheet.ExportAsCSV(Stream steam);
worksheet.ExportAsCSV(string filepath);
Adding Charts to a Worksheet via .NET
The ReoGrid component has provided very strong support for handling charts inside an Excel worksheet. It allows to display chart on a worksheet and saving into or loading from an Excel file. There are different types of charts supported inside a worksheet such as Line Chart, Column Chart, Bar Chart Pro, Area Chart, Pie Chart, Doughnut Chart, and so on. You can also easily modify your charts with ease.
How to Add Column Chart to Excel XLSX File via C# API?
var worksheet = this.grid.CurrentWorksheet;
worksheet["A2"] = new object[,] {
{ null, 2008, 2009, 2010, 2011, 2012 },
{ "City 1", 3, 2, 4, 2, 6 },
{ "City 2", 7, 5, 3, 6, 4 },
{ "City 3", 13, 10, 9, 10, 9 },
{ "Total", "=SUM(B3:B5)", "=SUM(C3:C5)", "=SUM(D3:D5)",
"=SUM(E3:E5)", "=SUM(F3:F5)" },
};
// Create three ranges, data source range, row title range and column title range
var dataRange = worksheet.Ranges["B3:F5"];
var rowTitleRange = worksheet.Ranges["A3:A6"];
var categoryNamesRange = worksheet.Ranges["B2:F2"];
worksheet.AddHighlightRange(rowTitleRange);
worksheet.AddHighlightRange(categoryNamesRange);
worksheet.AddHighlightRange(dataRange);