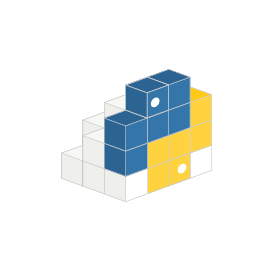
PyExcelerate
Python API for Creating Excel XLSX Spreadsheets
Create, Edit & Convert Excel OOXML File Format, Write Bulk Data, Merge and Style Cells via Open Source Python Spreadsheet Library.
What is PyExcelerate?
PyExcelerate enables software programmers to read and write Excel XLSX spreadsheet files. It is also known as an accelerated Excel XLSX writing library for Python because it is by far the fastest option for Python spreadsheet solutions. It creates 10,000 rows and 10 columns in just 0.17 seconds.
PyExcelerate supports writing bulk data, writing cell data, selecting cells by name, merging cells, styling cells, styling ranges, styling rows, styling columns, setting row heights and column widths and more.
Getting Started with PyExcelerate
First of all, you need to have Python 2.6 or higher installed on your system. After meeting the prerequisites, please use the following command.
PIP Command to Install PyExcelerate
pip install pyexcelerate
Python API to Generate & Modify Spreadsheet
PyExcelerate API enables software developers to generate an Excel-compatible XLSX spreadsheet from their own Python applications. Developers can also modify an existing sheet; write data to cells, select cells by name, merge cells, style rows or cells, and much more.
How to Write Spreadsheet Cell Data via Python?
from datetime import datetime
from pyexcelerate import Workbook
wb = Workbook()
ws = wb.new_sheet("sheet name")
ws.set_cell_value(1, 1, 15) # a number
ws.set_cell_value(1, 2, 20)
ws.set_cell_value(1, 3, "=SUM(A1,B1)") # a formula
ws.set_cell_value(1, 4, datetime.now()) # a date
wb.save("output.xlsx")
Writing Bulk Data to a Range
PyExcelerate API gives the developer the capability to write bulk data to an Excel range of cells. It is an important feature for adding a huge amount of data in less time. The API also gives developers the power to directly write data to ranges, which is quicker than writing cell-by-cell.
How to Write Bulk Data to a Rangevia via Python API?
from pyexcelerate import Workbook
wb = Workbook()
ws = wb.new_sheet("test")
ws.range("B2", "C3").value = [[1, 2], [3, 4]]
wb.save("output.xlsx")
Styling Cells in Excel Spreadsheet
Styles are very important part of a the way content are going to be appear on the screen and can be used to change the look of your data. The Open Source PyExcelerate API allows styling with custom colors, font, fill & more. Sometimes styling cells cause non-negligible overhead because it may increase the execution time.
How to Style Spreadsheet Cells via Python API?
from pyexcelerate import Workbook, Color
from datetime import datetime
wb = Workbook()
ws = wb.new_sheet("sheet name")
ws.set_cell_value(1, 1, 1)
ws.get_cell_style(1, 1).font.bold = True
ws.get_cell_style(1, 1).font.italic = True
ws.get_cell_style(1, 1).font.underline = True
ws.get_cell_style(1, 1).font.strikethrough = True
ws.get_cell_style(1, 1).fill.background = Color(0, 255, 0, 0)
ws.set_cell_value(1, 2, datetime.now())
ws.get_cell_style(1, 1).format.format = 'mm/dd/yy'
wb.save("output.xlsx")