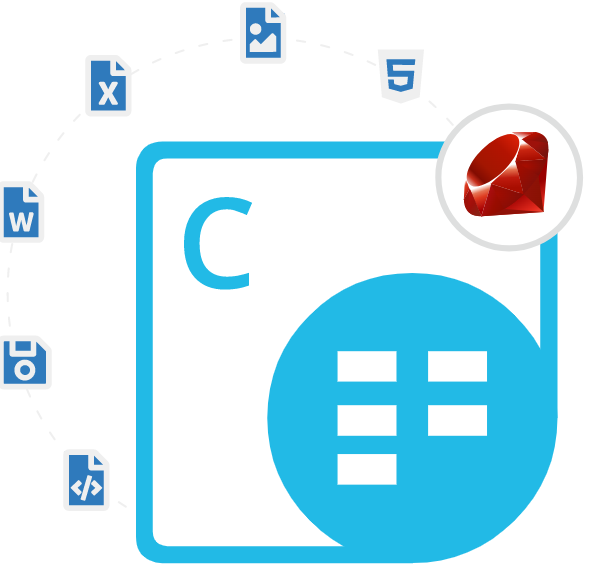
Aspose.Cells Cloud SDK for Ruby
Ruby REST API for Excel Spreadsheets
A powerful Ruby REST API to Create, Modify, Render, View, Manipulate & Convert Microsoft Excel & OpenOffice Spreadsheets in the cloud.
Aspose.Cells Cloud SDK for Ruby is a very dominant software development kit that enables software professionals to work with Microsoft Excel spreadsheets in the cloud without using Microsoft Office. The SDK is based on Aspose.Cells for Cloud, which is a REST-based API that provides a complete set of classes and methods for creating, manipulating, and converting Excel files. The library provides a simple and easy-to-use interface for interacting for creating, editing, and manipulating Excel spreadsheets in the cloud.
Aspose.Cells Cloud SDK for Ruby has provided support for a wide range of Microsoft Excel file formats such as XLS, XLSX, XLSB, XLSM, XLT, XLTX, XLTM, ODS, XML, CSV, TSV, TXT (TabDelimited), HTML, MHTML, DIF, PDF, XPS, TIFF, SVG, SXC, FODS and many more. Developers can easily create, edit, and manipulate charts in your Excel spreadsheets, including bar charts, line charts, pie charts, and more. The Library can be used to easily convert Excel files between different file formats, and work with files that have been created using different versions of Microsoft Excel.
Aspose.Cells Cloud SDK for Ruby is a powerful and flexible SDK that provides software developers with an easy way to work with Excel spreadsheets and supports a wide range of features, such as creating a new workbook, inserting new worksheets, renaming worksheets, adding Excel charts, customizing the appearance of your charts, creating Excel formulas, Add and update worksheet hyperlinks, insert ListObject at a specified place, user multiple types of list filters, add header & footer, insert images to worksheet, fetch worksheet pictures, split or merge rows/columns, encrypt Excel workbooks, add a digital signature for Excel workbook, find a text from an Excel workbook and many more.
Getting Started with Aspose.Cells Cloud SDK for Ruby
The recommend way to install Aspose.Cells Cloud SDK for Ruby is using RubyGem. Please use the following command for a smooth installation.
Install Aspose.Cells Cloud SDK for Ruby via RubyGem
gem install aspose_cells_cloud
You can also download it directly from Aspose product release page.Add & Manage Charts in Spreadsheet via Ruby API
Aspose.Cells Cloud SDK for Ruby has included complete support for working with Excel charts inside their own cloud applications. It allows the creation of various types of charts, such as bar charts, line charts, pie charts, and more. The library has included some useful features for handling charts in Microsoft Excel Worksheets, such as adding charts to worksheets, editing existing charts, manipulating charts, customizing the appearance of charts, deleting unwanted charts, converting chart to image, showing/hidding charts legends, get chart value, get chart axis, update chart properties and many more.
How to Add Charts in a Excel Worksheet via Ruby API?
class Chart
include AsposeCellsCloud
def initialize
# Get client_id and client_secret from https://cloud.aspose.com
@instance = AsposeCellsCloud::CellsApi.new($client_id,$client_secret,$api_version,$baseurl)
end
# Add new chart to worksheet.
def add_new_chart_to_worksheet
name = $MYDOC
sheet_name = $SHEET3
chart_type = 'Pie'
upper_left_row = 5
upper_left_column = 5
lower_right_row = 10
lower_right_column = 10
area = $CELLAREA
is_vertical = true
category_data = nil
is_auto_get_serial_name = nil
title = nil
folder = $TEMPFOLDER
result = @instance.upload_file( folder+"/"+name, ::File.open(File.expand_path("data/"+name),"r") {|io| io.read(io.size) })
expect(result.uploaded.size).to be > 0
result = @instance.cells_charts_put_worksheet_add_chart(name, sheet_name, chart_type, { :upper_left_row=>upper_left_row, :upper_left_column=>upper_left_column, :lower_right_row=>lower_right_row, :lower_right_column=>lower_right_column, :area=>area, :is_vertical=>is_vertical, :folder=>folder})
expect(result.code).to eql(200)
end
end
chart = Chart.new()
puts chart.add_new_chart_to_worksheet
Manage Shapes in Excel Spreadsheet via Ruby
Aspose.Cells Cloud SDK for Ruby has provided complete support for handling shapes inside Microsoft Excel Worksheets using Ruby REST API. There are several important features part of the library that makes the developer's job easy while working with Excel shapes, such as adding new shapes to Excel Worksheet, modifying properties of an existing shape, getting all shapes from a worksheet, getting a shape by Index inside on an Excel worksheet, delete all shapes on an Excel worksheet, convert a shape to image on an Excel worksheet and many more.
Convert shape to image via Ruby API
require 'aspose_cells_cloud'
class Worksheet
include AsposeCellsCloud
def initialize
#Get client_id and client_secret from https://cloud.aspose.com
@instance = AsposeCellsCloud::CellsApi.new($client_id,$client_secret,$api_version,$baseurl)
end
# Convert autoshape to Different File Formats.
def convert_autoshape_to_different_file_formats
name = $MYDOC
sheet_name = $SHEET2
autoshape_number = 4
format = 'PNG'
folder = $TEMPFOLDER
result = @instance.upload_file( folder+"/"+name, ::File.open(File.expand_path("data/"+name),"r") {|io| io.read(io.size) })
expect(result.uploaded.size).to be > 0
result = @instance.cells_autoshapes_get_worksheet_autoshape(name, sheet_name, autoshape_number, { :format=>format,:folder=>folder})
end
end
worksheet = Worksheet.new()
puts worksheet.convert_autoshape_to_different_file_formats
Excel Worksheet Handling via Ruby API
Aspose.Cells Cloud SDK for Ruby makes it easy for software developers to work with Excel worksheets inside their own Ruby cloud applications. There are several important features part of the library for handling worksheets, such as adding new Excel worksheets, getting worksheets in different file formats, adding worksheet backgrounds, sorting Excel worksheet data, hiding/unhiding Excel worksheets, sorting worksheet data, moving Excel worksheets, modify worksheet properties, freeze worksheet panes, autofit an Excel workbook, copy Excel worksheet, and many more. The following example shows how to copy contents and formats from another worksheet in the cloud inside Ruby applications.
Copy Excel Worksheet Formats via Ruby API
class Worksheet
include AsposeCellsCloud
def initialize
#Get client_id and client_secret from https://cloud.aspose.com
@instance = AsposeCellsCloud::CellsApi.new($client_id,$client_secret,$api_version,$baseurl)
end
# Copy worksheet
def post_copy_worksheet
name = 'NewCopy.xlsx'
sheet_name = $SHEET5
source_sheet = $SHEET6
options = AsposeCellsCloud::CopyOptions.new({:ColumnCharacterWidth=>true})
source_workbook = $BOOK1
source_folder = $TEMPFOLDER
folder = $TEMPFOLDER
result = @instance.upload_file( folder+"/"+name, ::File.open(File.expand_path("data/"+name),"r") {|io| io.read(io.size) })
expect(result.uploaded.size).to be > 0
result = @instance.upload_file( folder+"/"+source_workbook, ::File.open(File.expand_path("data/"+source_workbook),"r") {|io| io.read(io.size) })
expect(result.uploaded.size).to be > 0
result = @instance.cells_worksheets_post_copy_worksheet(name, sheet_name, source_sheet,{ :options=>options, :source_workbook=>source_workbook, :source_folder=>source_folder,:folder=>folder})
expect(result.code).to eql(200)
end
end
worksheet = Worksheet.new()
puts worksheet.post_copy_worksheet
Excel Worksheet Conversion via Ruby Excel API
Aspose.Cells Cloud SDK for Ruby has included complete support for Excel Worksheet Conversion to numerous popular file formats inside their own Ruby applications in the cloud. The library allows developers to import or export some popular Excel file formats, such as XLS, XLSX, XLSB, CSV, TSV, XLSM, ODS, TXT, and many more. It is also possible to convert from Excel file formats to some other popular formats, such as PDF, OTS, XPS, DIF, PNG, JPEG, BMP, SVG, TIFF, EMF, NUMBERS, FODS, and many more. The following example demonstrates how to convert Excel spreadsheets to other supported file formats inside Ruby applications.
Convert Excel File and Save Result to Storage via Ruby API
require 'aspose_cells_cloud'
class Workbook
include AsposeCellsCloud
def initialize
# Get client_id and client_secret from https://cloud.aspose.com
@instance = AsposeCellsCloud::CellsApi.new($client_id,$client_secret,$api_version,$baseurl)
end
# Convert document and save result to storage.
def post_document_save_as
name = $BOOK1
save_options = nil
newfilename = 'newbook.xlsx'
is_auto_fit_rows = true
is_auto_fit_columns = true
folder = $TEMPFOLDER
result = @instance.upload_file( folder+"/"+name, ::File.open(File.expand_path("data/"+name),"r") {|io| io.read(io.size) })
expect(result.uploaded.size).to be > 0
result = @instance.cells_save_as_post_document_save_as(name, { :save_options=>save_options, :newfilename=>(folder+"/"+newfilename), :is_auto_fit_rows=>is_auto_fit_rows, :is_auto_fit_columns=>is_auto_fit_columns, :folder=>folder})
expect(result.code).to eql(200)
end
end
workbook = Workbook.new()
puts workbook.post_document_save_as