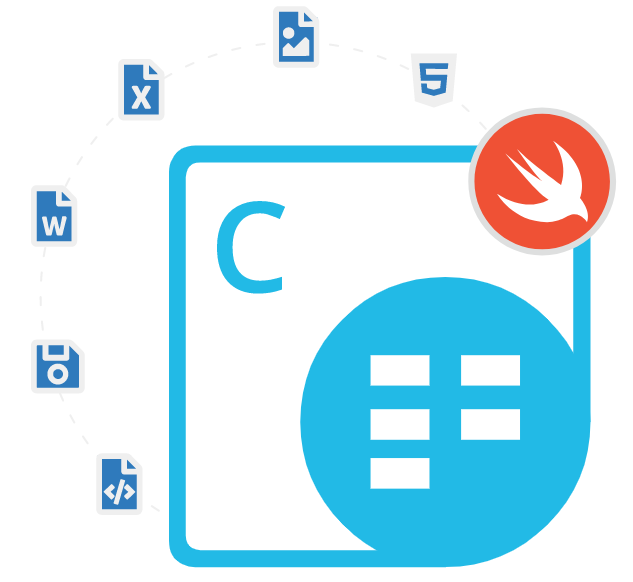
Aspose.Cells Cloud SDK for Swift
Swift API for Spreadsheet Management & Conversion
Cloud-based Swift API that provides a comprehensive set of features to create, edit, manage and manipulate spreadsheets in Swift applications.
What is Aspose.Cells Cloud SDK for Swift ?
Aspose.Cells Cloud SDK for Swift is a dominant cloud-based SDK that provides a comprehensive set of features enabling software developers to create, edit, convert, manage, and manipulate Microsoft Excel as well as OpenOffice spreadsheets inside their Swift applications. The API makes it easy for software programmers to work with popular spreadsheet file formats such as XLS, XLSX, XLSB, XLSM, XLT, XLTX, XLTM, XML, CSV, TSV, TXT, HTML, MHTML, ODS, and many more without the need for any additional software or tools. It also included support for applying formatting and styling to spreadsheets, including font styles, background colors, borders, and more.
With Aspose.Cells Cloud SDK for Swift, software developers can create spreadsheets from scratch on the fly using a wide range of templates, add data to existing spreadsheets, merge or split Excel workbooks, search text from Excel files, copy rows on an Excel worksheet, convert chart to image, add or delete a pivot table on an Excel worksheet, add a shape on an Excel worksheet, calculate all formulas on an Excel workbook, group/Ungroup rows on an Excel Worksheet, add a filter for a filter column on an Excel worksheet, import data into Excel files and many more. The SDK supports a range of advanced operations that are commonly required in spreadsheet management, such as the ability to protect and unprotect worksheets, add and remove comments, and perform conditional formatting based on predefined rules.
Aspose.Cells Cloud SDK for Swift has included the ability to import or export Excel spreadsheets to various popular file formats such as XLS, XLSX, XLSB, CSV, TSV, XLSM, ODS, TXT, PDF, OTS, XPS, DIF, PNG, JPEG, BMP, SVG, TIFF, EMF, NUMBERS, FODS, and many more. If you're a developer who works with spreadsheet data on a regular basis, Aspose.Cells Cloud SDK for Swift is an invaluable tool that can help you streamline your workflow and save time and effort. With its comprehensive set of features and easy-to-use API, it's the perfect solution for managing your spreadsheet data in the cloud.
Getting Started with Aspose.Cells Cloud SDK for Swift
The recommend way to install Aspose.Cells Cloud SDK for Swift is using CocoaPods. Please use the following command for a smooth installation.
Install Aspose.Cells Cloud SDK for Swift via CocoaPods
'AsposeCellsCloud', '~> 21.7'
pod install
You can also download it directly from GitHub.
Create Excel Spreadsheet in Different Ways via Swift API
Aspose.Cells Cloud SDK for Swift has included complete support for generating and managing Excel spreadsheet documents in various file formats inside Swift applications. The library enables software developers to create and manage workbooks in different ways such as creating an empty workbook from the scratch, creating an Excel workbook with a smart marker template or creating an Excel workbook with a template file, getting pages count on an Excel workbook, set and clear password for an Excel workbook, auto-fit columns on an Excel workbook and many more.
Excel Spreadsheet Conversion via C#.NET API
Aspose.Cells Cloud SDK for Swift gives software developers the power to convert their Excel worksheets to various other supported file formats using Swift commands. The SDK allows Excel Spreadsheet conversion to PDF, HTML, PowerPoint, XPS, HTML, MHTML, JSON, Plain Text, and popular image formats including TIFF, JPG, PNG, BMP, and SVG. To convert a file to a desired file format, first, you need to upload the file to Aspose Cloud Storage and then with just a couple of lines of code, you can convert it to the supported file formats.
How to Convert Excel File to PDF via Swift API?
//upload the Excel file to Aspose Cloud Storage
let localFilePath = "path/to/your/local/file.xlsx"
let remoteFolderPath = "your/remote/folder"
let remoteFileName = "file.xlsx"
let uploadRequest = UploadFileRequest(
fileContent: localFilePath.data(using: .utf8)!,
path: "\(remoteFolderPath)/\(remoteFileName)"
)
cellsApi.uploadFile(request: uploadRequest) { response, error in
if let error = error {
print("Error uploading file: \(error)")
} else {
print("File uploaded successfully")
}
}
// convert it to your desired forma
let format = "pdf" // or any other supported format
let convertRequest = PostDocumentSaveAsRequest(
name: remoteFileName,
saveOptions: SaveOptions(
saveFormat: format,
defaultFont: "Arial"
),
folder: remoteFolderPath,
storage: nil
)
cellsApi.postDocumentSaveAs(request: convertRequest) { response, error in
if let error = error {
print("Error converting file: \(error)")
} else {
print("File converted successfully")
// download the converted file using the response's outputFilePath property
}
}
Excel Formulas Calculations Support via Swift
Aspose.Cells Cloud SDK for Swift has provided full support for various Excel formulas and calculations inside Swift applications. You can use the SDK to perform calculations on your Excel files programmatically. The library supports calculates the sum of a range of cells, calculates the average of a range of cells, counts the number of cells that contain numbers in a range of cells, finds the maximum value in a range of cells, finds the minimum value in a range of cells, conditional formulas support, array formulas, financial formulas support and many more. The following example shows how to calculate the sum of a range of cells of a worksheet using Swift commands.
How to Calculate the Sum of Range of Cells via Swift API?
let cellsApi = CellsAPI(appKey: "your_app_key", appSid: "your_app_sid")
let filename = "sample.xlsx"
let worksheet = "Sheet1"
let range = "A1:A10"
cellsApi.cellsGetWorksheetCellRangeValue(name: filename, sheetName: worksheet, range: range, storage: nil, folder: nil) { (response, error) in
if error == nil {
let cellValues = response?.value
let sum = cellValues?.reduce(0, { x, y in x + (y as? Double ?? 0)})
print("Sum of the range \(range) in \(worksheet) is \(sum ?? 0)")
} else {
print("Error while calculating sum: \(error?.localizedDescription ?? "")")
}
}
Add Charts & Images in Excel Files via Swift
Aspose.Cells Cloud SDK for Swift has provided some useful features for handling images as well as charts inside Excel worksheets using Swift code. The API supports adding a chart to a worksheet, getting a chart from the worksheet, deleting an unwanted chart from a worksheet, exporting a chart to an image, getting chart legends from a worksheet, hiding chart legends, adding or updating chart title, get chart value, update chart category, update chart values, update chart second category axis and so on. Same as charts software developers can also add, delete, update, and convert images inside a worksheet. The following example shows how software developers can add a chart to Excel File using Swift code.
How to Add Charts to Excel File via Swift API?
let name = "Workbook1.xlsx"
let sheetName = "Sheet1"
let chartType = "Bar"
let upperLeftRow = 5
let upperLeftColumn = 5
let lowerRightRow = 20
let lowerRightColumn = 20
let chartTitle = AsposeCellsCloud.DTO.ChartTitle()
chartTitle.text = "Sales Data"
let chartArea = AsposeCellsCloud.DTO.ChartArea()
let series = AsposeCellsCloud.DTO.Series()
let seriesData = AsposeCellsCloud.DTO.SeriesData()
let chart = AsposeCellsCloud.DTO.Chart()
chart.chartTitle = chartTitle
chart.chartArea = chartArea
chart.series = [series]
chart.seriesData = [seriesData]
self.cellsAPI.putWorksheetAddChart(name: name, sheetName: sheetName, chartType: chartType, upperLeftRow: upperLeftRow, upperLeftColumn: upperLeftColumn, lowerRightRow: lowerRightRow, lowerRightColumn: lowerRightColumn, chart: chart, storage: nil, folder: nil) { response, error in
if let error = error {
print("Error while adding chart: \(error)")
} else {
print("Chart added successfully.")
}
}