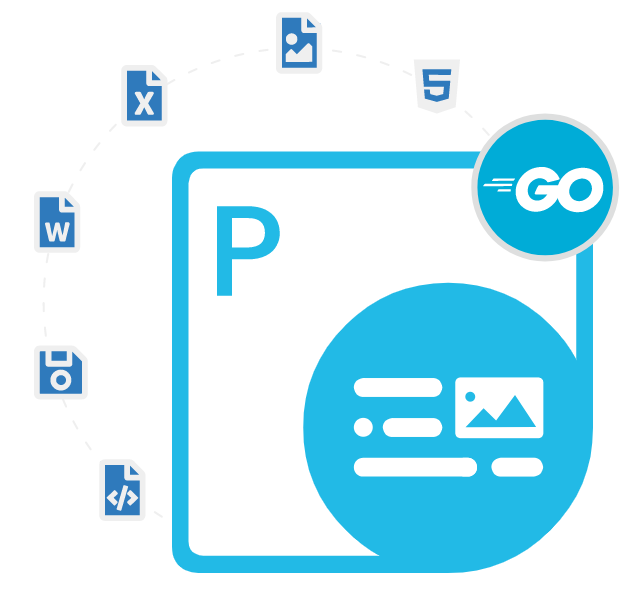
Aspose.PDF Cloud Go SDK
GO Cloud SDK for PDF Generation & Conversion
A Leading REST API to Generate, Modify, Manipulate and Export PDF files to HTML, PCL, XPS, TIFF, JPEG, SVG & more without using Adobe Acrobat.
Aspose.PDF Cloud is a powerful REST API that enables software developers to create, edit, annotate, convert, and manipulate PDF files in the cloud. The Aspose.PDF Cloud Go SDK has provided a very simple and efficient way to allow Go developers to use the Aspose.PDF Cloud API to handle PDF documents inside their applications. The Go SDK makes it easy for software developers to convert PDF files to a wide range of file formats, including XLS, XLSX, PPTX, DOC, DOCX, MobiXML, JPEG, EMF, PNG, BMP, GIF, TIFF, Text, and more. The library also provides support for converting non-PDF files to PDF format inside Go applications.
Aspose.PDF Cloud Go SDK is highly flexible and has included some useful functions that allow users to perform various operations on PDF files, such as creating new PDF documents, converting PDF files to different formats, adding watermarks & annotations to PDFs, adding or removing pages, updating text or images, setting security permissions, adding comments, and many more. Software developers can use the SDK to work with PDF forms, including creating, filling, and submitting forms. Developers can also perform PDF optimization operations, such as reducing PDF file size, removing unused resources, and optimizing images.
Aspose.PDF Cloud Go SDK enables programmers to extract various elements of PDF documents with ease, such as extracting PDF document annotations and images including GIF, JPEG, PNG, and TIFF format, and save it to a place of your choice. Another great feature supported by the SDK is adding OCR layers to PDF files and creating searchable documents from scanned PDF Files. With its wide range of functions for creating, editing, and manipulating PDF files, the SDK can help you build sophisticated PDF-based applications in the cloud.
Getting Started with Aspose.PDF Cloud Go SDK
The recommend way to install Aspose.PDF Cloud Go SDK is using GitHub. Please use the following command for a smooth installation.
Install Aspose.PDF Cloud Go SDK via GitHub
go get -u github.com/aspose-pdf-cloud/aspose-pdf-cloud-go/v23
You can download the library directly from Aspose.PDF product page
Create PDF Documents from Other Formats via Go
Aspose.PDF Cloud Go SDK provides complete support for PDF document creation and management inside Cloud-based applications. The library has included numerous features for PDF generation from other file formats, such as PDF creation from images, HTML, JPEG, PNG, TIFF, XML and many more using Go REST APIs. The following example demonstrates how to create empty PDF documents using Go commands.
Create an Empty PDF File via Go REST API
// For complete examples and data files, please go to https://github.com/aspose-pdf/Aspose.Pdf-for-Cloud
fileName := "input.pdf"
// init words cloud api
config := asposepdfcloud.NewConfiguration(AppSid, AppKey, BaseURL)
client := asposepdfcloud.NewAPIClient(config)
options := map[string]interface{}{
"storage": "First Storage",
}
//Create empty document
documentResponse, response, _ := client.PdfApi.PutCreateDocument(fileName, options)
fmt.Println(response.Status)
fmt.Println(documentResponse.Document.Pages.List[0].Id)
Convert PDF Document to Other Formats via Go
Converting PDF documents to other file format is a common task that many developers need to perform. Aspose.PDF Cloud Go SDK has facilitate software developers by providing complete functionality for loading and converting PDF documents to numerous other supported file formats. The SDK supports conversion to EPUB, HTML, MHT, PCL, DOC, PDFA, PPTX, SVG, TIFF, XLS, XLSX, XML, XPS, PS PCL, BMP, EMF, GIF, JPEG, PNG, TIFF, and many more. Software developers can also upload the resulting file to a storage and use it according to their own needs. The following example shows how to convert PDF to Doc file format inside Go applications.
Convert PDF to DOC File via Go REST API
import (
"github.com/aspose-pdf-cloud/aspose-pdf-cloud-go/pdf_api"
)
pdfApi, err := pdf_api.NewPdfApi("Client ID", "Client Secret", "API Version")
convertToFormatRequest := pdf_api.ConvertToFormatRequest{Name: "input.pdf", Format: "doc"}
response, err := pdfApi.ConvertToFormat(ctx, convertToFormatRequest)
It
// Save the output DOC file:
outputFile, err := os.Create("output.doc")
defer outputFile.Close()
io.Copy(outputFile, response)
Insert & Manage Image in PDF via Go API
Aspose.PDF Cloud Go SDK has provided some useful features for handling various types of images inside PDF documents using Go REST API. The SDK has included support for popular image file formats like BMP, GIF, JPEG, PNG, TIFF, and more. There are several important features part of the library for working with images in PDF files such as inserting new images into PDF, reading image information, replacing existing images in a PDF, extracting a particular image from a PDF, exporting document images to an other format, and many more. The following example shows how software developers can replace an existing image inside PDF documents using Go commands.
How to Replace Images in PDF Document via Go API?
// For complete examples and data files, please go to https://github.com/aspose-pdf/Aspose.Pdf-for-Cloud
fileName := "4pages.pdf"
filePath := "data/4pages.pdf"
imageFile := "butterfly.jpg"
imageFilePath := "data/butterfly.jpg"
imageID := "GE5TKOBSGI2DWMJQHAWDOMRMGEYDSLBXGM"
// init words cloud api
config := asposepdfcloud.NewConfiguration(AppSid, AppKey, BaseURL)
client := asposepdfcloud.NewAPIClient(config)
// Upload document
file, _ := os.Open(filePath)
_, _, err1 := client.PdfApi.UploadFile(fileName, file, nil)
if err1 != nil {
fmt.Println(err1)
}
// Upload image
imgFile, _ := os.Open(imageFilePath)
_, _, err2 := client.PdfApi.UploadFile(imageFile, imgFile, nil)
if err2 != nil {
fmt.Println(err2)
}
options := map[string]interface{}{
"storage": "First Storage",
"imageFilePath": imageFile,
"image": imgFile,
}
//Replace image
imageResponse, response, _ := client.PdfApi.PutReplaceImage(fileName, imageID, options)
fmt.Println(response.Status)
fmt.Println(imageResponse.Image.Id)
Manage Pages inside PDF via Go REST API
Aspose.PDF Cloud Go SDK makes it easy for computer programmers to work with PDF pages inside their own Go cloud applications. The SDK has included several important features for handling pages, such as adding new pages to PDF files, retrieving PDF page information, moving pages to a new location in PDF files, deleting unwanted PDF pages, PDF file page to image format conversion, getting page count of a PDF, signing PDF page, getting PDF page count and many more. The following example shows how to add a new page to a PDF document inside Go applications.
Insert New Page to PDF Document via Go REST API
// For complete examples and data files, please go to https://github.com/aspose-pdf/Aspose.Pdf-for-Cloud
fileName := "4pages.pdf"
filePath := "data/4pages.pdf"
// init words cloud api
config := asposepdfcloud.NewConfiguration(AppSid, AppKey, BaseURL)
client := asposepdfcloud.NewAPIClient(config)
// Upload 1st document
file, _ := os.Open(filePath)
_, _, err1 := client.PdfApi.UploadFile(fileName, file, nil)
if err1 != nil {
fmt.Println(err1)
}
options := map[string]interface{}{
"storage": "First Storage",
}
//Add new page to PDF doc
documentPageResponse, response, _ := client.PdfApi.PutAddNewPage(fileName, options)
fmt.Println(response.Status)
fmt.Println(documentPageResponse.Pages.List[0].Id)