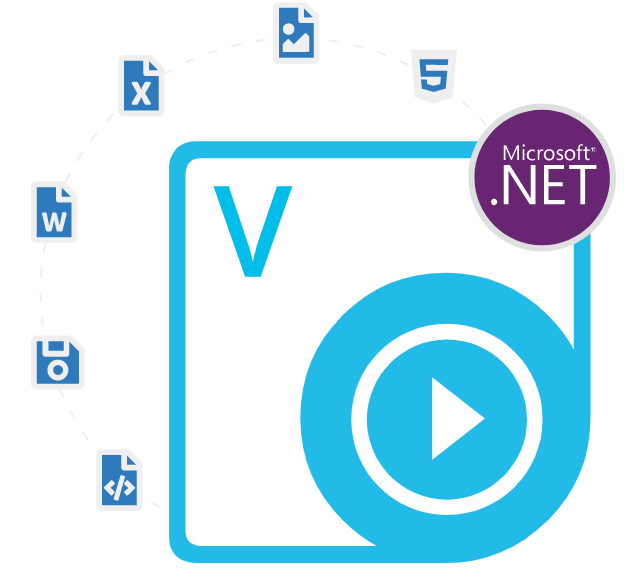
Aspose.Video Cloud SDK for .NET
C# .NET API to Read, Combine & Convert Videos
Advanced Videos Processing C# .NET Library allows Software Developers to Load, Read, Merge, Re-encode & Convert Videos in the Cloud.
Aspose.Video Cloud SDK for .NET is a powerful cloud-based API that enables developers to perform a wide range of video-related operations without the need for complex programming. The library allows software developers to work with remote video files and can easily upload, edit, download, view, and delete video files from cloud storage services such as Amazon S3, Google Cloud Storage, Dropbox, and many others. It is a fully-managed service that doesn’t require any hardware or infrastructure management.
Aspose.Video Cloud SDK for .NET is designed to be straightforward to use, with well-documented resources, samples, and code snippets. This API provides a variety of features for working with videos, including video conversion, resizing, cropping, and trimming, extracting audio from videos, generating video thumbnails, applying watermarks to videos, and many more. The cloud-based API is built on top of Aspose.Video for .NET, a robust library for working with video files in .NET.
Aspose.Video Cloud SDK for .NET provides a wide range of video formats, including WMV, AVI, FLV, MKV, MK3D, MKA, MKS, WEBM, MP4, MXF, MOV, QT, and more. The library also included support for some video and audio codecs such as Apple ProRes, DNxHD, H.264, H.265, Motion JPEG, VP6, P8, VP9, XviD, Windows Media Video v9, AAC, AC-3, MP3, Opus and many more. This makes Aspose.Video is an ideal solution for a wide range of applications, from video editing and media management to video streaming and sharing.
Getting Started with Aspose.Video Cloud SDK for .NET
The recommend way to install Aspose.Video Cloud SDK for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Video Cloud SDK for .NET via NuGet
Install-Package Aspose.Video-Cloud
You can also download it directly from Aspose product release page.Convert Video to Other Formats via .NET API
Aspose.Video Cloud SDK for .NET has included complete support for converting video files to other supported file formats inside .NET applications. The library has included various important features related video loading and conversion, such as adjusting video properties (FPS, resolution, volume level), converting video from AVI to mp4 format, adding text watermark into the video, adding image watermark into the video, changing video resolution, encoding the video with different codec, increase or decrease video playback speed, adjust video aspect ratio, making sound of the video quieter half of the original, adjusting video bitrate and many more.
Convert AVI Videos intoMP4 File Format
// For complete examples and data files, please go to https://github.com/aspose-video-cloud/aspose-video-cloud-dotnet/
using Aspose.Video.Cloud.Sdk.Api;
using Com.Aspose.Storage.Api;
using Aspose.Video.Cloud.Sdk.Model;
using Aspose.Video.Cloud.Sdk.Model.Requests;
private const string BaseProductUri = @"https://api.aspose.cloud/v1.1";
// Get App Key and App SID from https://dashboard.aspose.cloud/
private const string AppSID = "";
private const string AppKey = "";
this.VideoApi = new VideoApi(AppKey, AppSID, BaseProductUri);
this.StorageApi = new StorageApi(AppKey, AppSID, BaseProductUri);
var localName = "sample.avi";
var remoteName = "toconvert.avi";
var fullName = Path.Combine(this.dataFolder, remoteName);
var resultPath = Path.Combine(this.dataFolder, "converted.mp4");
ConvertOptions options = new ConvertOptions();
this.StorageApi.PutCreate(fullName, null, null, File.ReadAllBytes(BaseTestContext.GetDataDir() + localName));
var request = new PostConvertVideoRequest(remoteName, "mp4", resultPath, options, this.dataFolder);
var actual = this.VideoApi.PostConvertVideo(request);
Aspose.Video Cloud SDK for .NET
Extract Part of a Video via .NET API
Aspose.Video Cloud SDK for .NET enables software developers to load their videos in different file formats and extract part of their videos without any external dependencies. The library allows extracting part of a video using Aspose.Video by specifying the start and end time of the desired segment using the ExtractVideoApi class. The API allows developers to set additional options for the segment extraction, such as the output format and the quality of the extracted video. The response object returned contains the extracted video data, which you can save to a file using the Save method.
Extract Part of a Video via .NET API
// First, you need to initialize the VideoApi instance
var videoApi = new VideoApi(apiKey, appSid, basePath);
// Specify the name of the source video file and the start and end times of the desired segment in seconds
var name = "sample.mp4";
var startTime = 30; // start time in seconds
var endTime = 60; // end time in seconds
// Set the options for the segment extraction
var options = new ExtractOptions
{
StartTime = startTime,
EndTime = endTime
};
// Extract the desired segment from the video and save it to a new file
var response = videoApi.ExtractVideo(name, options);
response.Save("extracted.mp4");
How to Get Video Properties via .NET API
Aspose.Video Cloud SDK for .NET has included complete support for handling video properties inside their own C# .NET applications. The API returns an object that contains information about the video such as codec, resolution, path, duration etc. Audio properties are also included. The following code example demonstrates how to retrieve the video properties and use it according to their needs.
How to Get Video Properties via .NET API?
using Aspose.Video.Cloud.Sdk.Api;
using Com.Aspose.Storage.Api;
using Aspose.Video.Cloud.Sdk.Model;
using Aspose.Video.Cloud.Sdk.Model.Requests;
private const string BaseProductUri = @"https://api.aspose.cloud/v1.1";
// Get App Key and App SID from https://dashboard.aspose.cloud/
private const string AppSID = "";
private const string AppKey = "";
var localName = "sample.avi";
var remoteName = "TestGetVideo.avi";
var fullName = Path.Combine(this.dataFolder, remoteName);
this.StorageApi.PutCreate(fullName, null, null, File.ReadAllBytes(BaseTestContext.GetDataDir() + localName));
var request = new GetVideoPropertiesRequest(remoteName, this.dataFolder);
var actual = this.VideoApi.GetVideoProperties(request);