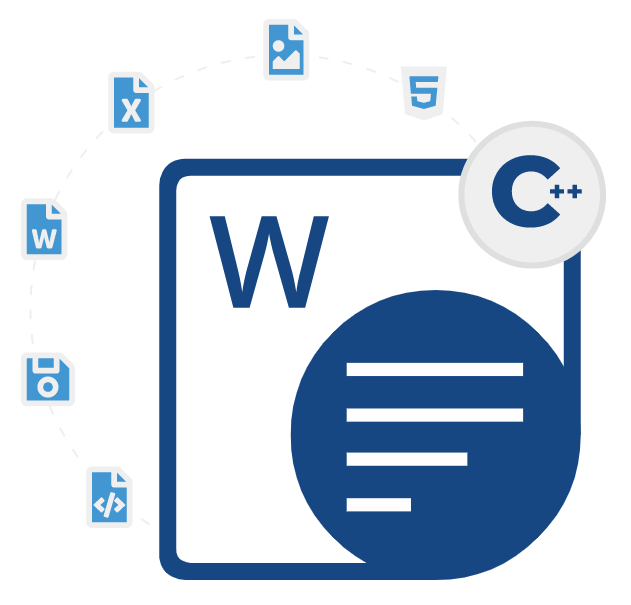
Aspose.Words for C++
C++ API to Create & Convert MS Word Documents
A Powerful C++ Word document processing Library to Generate, Edit, Encrypt, Convert, & Manipulate Microsoft Word Files without using Microsoft Word.
What is Aspose.Words for C++?
Aspose.Words for C++ is a high-performance word processing API tailored for C++ developers building desktop, web, or cloud-based applications. Without relying on Microsoft Word, it allows seamless document creation, editing, rendering, and format conversion across platforms like Windows and Linux. Whether you're working with C++ DOCX or DOC files, this versatile library supports a broad range of formats including RTF, ODT, HTML, and PDF. Developers can programmatically handle content, apply formatting, and execute advanced tasks like mail merge, document comparison, and structured reporting—all through clean and efficient C++ code.
Ideal for modern word processing app development via C++, this robust API also enables conversion of Word documents to PDF or image formats like PNG, JPEG, and TIFF. Developers can embed HTML, insert online videos, generate DML charts, and even read/write VBA scripts within documents. Its rich functionality supports scalable document automation workflows, making it a powerful alternative to open source word processing C++ libraries. Whether you’re building large-scale document generation tools or integrating PDF conversion in C++ word processing apps, Aspose.Words for C++ delivers speed, reliability, and extensive file support.
Getting Started with Aspose.Words for C++
The recommend way to install Aspose.Words for C++ is via NuGet. Please use the following command for a smooth installation.
Install Aspose.Words for C++ via NuGet
NuGet\Install-Package Aspose.Words.Cpp -Version 22.12.0
You can also download it directly from Aspose product page.Convert Word Document via C++ API
Aspose.Words for C++ makes it is easy for software developers to load and convert various types of documents inside their own C++ applications. The library can convert a document from any supported load format into any supported save format. The library supports Word Doc and DOCX conversion to PDF, DOCX to JPEG and PNG, Convert a Document to Markdown, convert Word to HTML and Web formats to PDF. Apart from the it can also convert DOCX to DOC, HTML to Word, RTF to PDF, ODT to PDF, TXT to PDF, Convert MHT (MHTML) to PDF and so on.
Convert Word DOC to PDF via C++ API
// Load the document from disk.
System::SharedPtr doc = System::MakeObject(inputDataDir + u"Rendering.doc");
System::String outputPath = outputDataDir + u"Doc2Pdf.SaveDoc2Pdf.pdf";
// Save the document in PDF format.
doc->Save(outputPath);
Apply Mail Merge in C++ Apps
Aspose.Words for C++ has included complete support for creating various types of documents such as such as letters, labels, and envelopes using Mail Merge features. The library allows documents creation from templates using mail merge fields. Using standard mail merge fields you can design reports in Microsoft Word, insert images, define regions in the document that are growing, documents filling with data from any type of data source and so on. After executing the mail merge, save the resulting document by calling the Save method on the Document object and passing in the file path where you want to save the resulting document.
Create Documents using Mail Merge via C++ API
using namespace Aspose::Words;
void MailMerge()
{
// ExStart:MailMerge
// Create a new document.
System::SharedPtr doc = System::MakeObject();
doc->get_MailMerge()->set_CleanupOptions(MailMergeCleanupOptions::RemoveUnusedFields);
// Execute mail merge.
doc->get_MailMerge()->Execute(
{ u"FullName", u"Company", u"Address", u"Address2", u"City" },
{ { u"James Bond", u"MI6", u"Milbank", u"", u"London" },
{ u"Ethan Hunt", u"IMF", u"Curzon Street", u"", u"London" } });
// Save the document to disk.
doc->Save(u"MailMerge.docx");
// ExEnd:MailMerge
}
Add/Manage paragraph to Word Documents via C++
Aspose.Words for C++ has included complete support for working with paragraph inside word documents. The library allows inserting a new paragraph as well as managing existing one with ease. There library has provided various features for working with paragraph such as apply formatting to a paragraph, auto adjust space between Asian and Latin text, numbers, set line break options, apply styles to paragraph, insert a style separator to put different paragraph styles, identifying style separator paragraph, add borders and shading to a paragraph and so on.
Apply Borders and Shading to a Paragraph via C++ API
System::SharedPtr doc = System::MakeObject();
System::SharedPtr builder = System::MakeObject(doc);
// Set paragraph borders
System::SharedPtr borders = builder->get_ParagraphFormat()->get_Borders();
borders->set_DistanceFromText(20);
borders->idx_get(BorderType::Left)->set_LineStyle(LineStyle::Double);
borders->idx_get(BorderType::Right)->set_LineStyle(LineStyle::Double);
borders->idx_get(BorderType::Top)->set_LineStyle(LineStyle::Double);
borders->idx_get(BorderType::Bottom)->set_LineStyle(LineStyle::Double);
// Set paragraph shading
System::SharedPtr shading = builder->get_ParagraphFormat()->get_Shading();
shading->set_Texture(TextureIndex::TextureDiagonalCross);
shading->set_BackgroundPatternColor(System::Drawing::Color::get_LightCoral());
shading->set_ForegroundPatternColor(System::Drawing::Color::get_LightSalmon());
builder->Write(u"I'm a formatted paragraph with double border and nice shading.");
System::String outputPath = outputDataDir + u"DocumentBuilderSetFormatting.ApplyBordersAndShadingToParagraph.doc";
doc->Save(outputPath);
Protect or Encrypt a Document via C++ API
Aspose.Words for C++ enables software developers to protect various types of documents inside their own C++ applications. By protecting or encrypting a document means to apply more control over who can access, copy or modify documents without permission. The library has provided various useful features for protecting your documents, such as Open a Document Read-Only, Encrypt a Document, Restrict Document Editing, Add a Digital Signature and so on.
How to Encrypt a Document with Password via C++ API
// Create a document.
auto doc = System::MakeObject();
auto builder = System::MakeObject(doc);
builder->Write(u"Hello world!");
// DocSaveOptions only applies to Doc and Dot save formats.
auto options = System::MakeObject(SaveFormat::Doc);
// Set a password with which the document will be encrypted, and which will be required to open it.
options->set_Password(u"MyPassword");
doc->Save(u"DocSaveOptions.SaveAsDoc.doc", options);