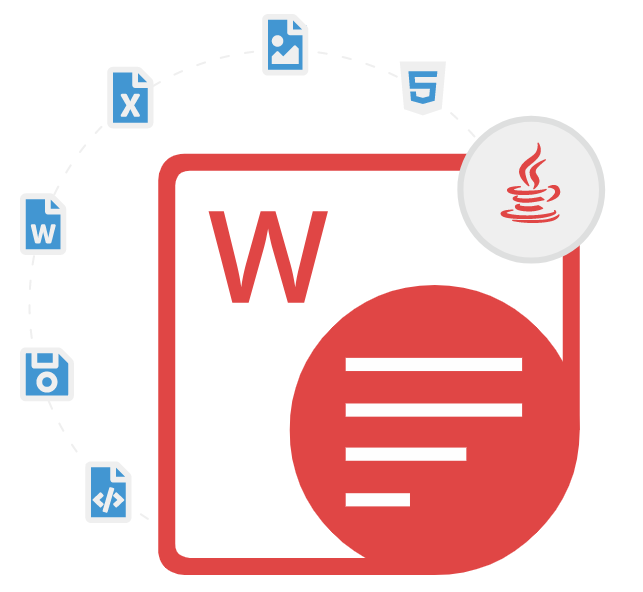
Aspose.Words for Java
Java API to Process Microsoft Word Documents
Cross-platform Java library to create, modify, convert, render, and print Word processing documents without Microsoft Word, or Office Automation
What is Aspose.Words for Java?
Aspose.Words for Java is a powerful and feature-rich Java Word processing API that enables software developers and programmers to generate Word documents with ease, edit, convert, render, merge/split, protect and print files—without needing Microsoft Word or Office Automation. Fully compatible with server and client-side applications, it integrates smoothly across major Java environments and platforms.
This leading Word processing API supports 35+ file formats, including DOC, DOCX, RTF, PDF, XPS, ODT, HTML, and popular image types like PNG, JPEG, SVG, and TIFF. It allows developers to easily convert Word documents in Java, such as convert DOCX to PDF, with high accuracy. The API also offers advanced features like mail merge, document rendering, report creation, font and image management, paragraph editing, and 3D effects. Whether you need to create DOCX files, manipulate pages, or build cross-platform applications for Windows, Linux, macOS, or Android, Aspose.Words for Java provides everything to streamline Word document creation and conversion.
Getting Started with Aspose.Words for Java
The recommend way to install Aspose.Words for Java is via Maven repository. You can easily use Aspose.Words for Java API directly in your Maven Projects with simple configurations:.
Aspose.Words for Java Maven Dependency
//Define the Aspose.Words for Java API dependency in your pom.xml as follows
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-words</artifactId>
<version>22.11</version>
</dependency>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-words</artifactId>
<version>22.11</version>
<classifier>javadoc</classifier>
</dependency>
</dependencies>
You can download the directly from Aspose.Words Release pageDocument Creation & Loading via Java API
Aspose.Words for Java allows software developers to programmatically create a new blank document or add document contents inside their own Java applications. To create a blank word document you just need to call the Document constructor without a parameter. It is very easy to load an existing document, just need to pass the document name or the stream into one of the Document constructors. The library recognizes the format of the loaded file by its extension. Once the document is created you can easily add text, images, shapes, fonts, define styles and formatting, set page size, insert tables and charts, add headers/footers, and so on.
Create Word Document via Java API
// The path to the documents directory.
String dataDir = Utils.getDataDir(CreateDocument.class);
// Load the document.
Document doc = new Document();
DocumentBuilder builder = new DocumentBuilder(doc);
builder.write("hello world");
doc.save(dataDir + "output.docx");
Word Document Rendering via Java API
Aspose.Words for Java library gives software developers the power to render Word documents or part of the document inside their own Java applications. The library has included very powerful rendering features, such as rendering a document to fixed-layout formats, exporting document or selected pages into PDF, XPS, HTML, XAML, PostScript, and PCL formats, rendering a document into a multi-page TIFF document, converting any page into a raster image ( BMP, PNG, JPEG), document page conversion into SVG image, and many more.
How to Save a Document to JPEG Format via Java API?
Document doc = new Document(dataDir + "Rendering.doc");
// Save as a JPEG image file with default options
doc.save(dataDir + "Rendering.JpegDefaultOptions.jpg");
// Save document to stream as a JPEG with default options
OutputStream docStream = new FileOutputStream(dataDir + "Rendering.JpegOutStream.jpg");
doc.save(docStream, SaveFormat.JPEG);
// Save document to a JPEG image with specified options.
// Render the third page only and set the JPEG quality to 80%
// In this case we need to pass the desired SaveFormat to the ImageSaveOptions
// constructor
// to signal what type of image to save as.
ImageSaveOptions imageOptions = new ImageSaveOptions(SaveFormat.JPEG);
imageOptions.setPageSet(new PageSet(2, 1));
imageOptions.setJpegQuality(80);
doc.save(dataDir + "Rendering.JpegCustomOptions.jpg", imageOptions);
Join & Split Word Documents via Java Library
CIt is open needed to join various documents into a single document or split a large file into smaller ones. Aspose.Words for Java library has provided various useful features for combining and splitting documents using Java library. It allows developers to insert the content of another document to a newly created document or append a document only at the end of another document. The library has provided various ways for inserting a document to other files such as inserting a file during mail merge operation, inserting a document at a bookmark, adding a document to the end of another one, importing and inserting nodes manually, and so on. Same like joining the library also included several functions for splitting documents such as splitting a document by headings, splitting a document by sections, splitting a document page by page, splitting a multi-page document page by page, and so on.
How to Split a Document Page by Page via Java API?
// For complete examples and data files, please go to https://github.com/aspose-words/Aspose.Words-for-Java
Document doc = new Document(dataDir + "TestFile (Split).docx");
int pageCount = doc.getPageCount();
// Save each page as a separate document.
for (int page = 0; page <= pageCount; page++)
{
Document extractedPage = doc.extractPages(page, 1);
extractedPage.save(dataDir + "SplitDocumentPageByPageOut_" + (page + 1) + ".docx");
}
Print Word Documents inside Java Apps
Aspose.Words for Java enables software developers to print various types of documents inside their own Java applications. The library has provided support for print preview dialog to visually examine how the document will appear and select a needed print option. Using the MultipagePrintDocument class programmers can print multiple pages of a document on a single sheet of paper.
How to Print Multiple Pages on One Sheet via Java API?
Document doc = new Document(dataDir + "TestFile.doc");
// Create a print job to print our document with.
PrinterJob pj = PrinterJob.getPrinterJob();
// Initialize an attribute set with the number of pages in the document.
PrintRequestAttributeSet attributes = new HashPrintRequestAttributeSet();
attributes.add(new PageRanges(1, doc.getPageCount()));
// Pass the printer settings along with the other parameters to the print document.
MultipagePrintDocument awPrintDoc = new MultipagePrintDocument(doc, 4, true, attributes);
// Pass the document to be printed using the print job.
pj.setPrintable(awPrintDoc);
pj.print();