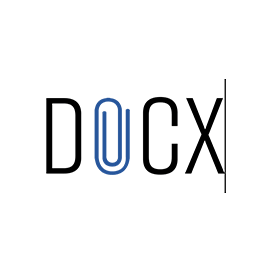
Docx
Generate & Manage Word DOCX via JavaScript Library
Open Source JavaScript Library to create, modify & convert Microsoft Word DOCX Documents. Add paragraph, headers & footers, Tables, bullet and numbers inside Word files.
What is Docx?
Docx is an open source JavaScript API that provides the capability to generate and manage Word DOCX files inside their own JavaScript application with ease. The library can smoothly work for Node as well as on the Browser. The library is very stable and easy to use. With just a couple of lines of code, developers can create and manipulate word documents without any external dependencies.
The Docx API has included support for several important features related to working with Word documents such as creating Word documents, modifying DOCX files, adding a paragraph to a word file, adding and managing headers & footers, inserting and editing tables, bullet and numbering support, Table of content creation, set document margins, set page size, text alignment, Manage fonts and font sizes, document sections creation and many more.
Getting Started with Docx
The most recent release of DOCX can be installed directly from the online DOCX repository by applying the following command.
Install DOCX via npm
npm install --save docx
Word Docx File Creation via JavaScript API
The open source DOCX API enables software developers to generate new Word documents in DOCX file formats with just a couple of lines of JavaScript code. Once the document is created you can easily modify it and add all your content such as Paragraphs, images, tables, etc. to it. You can also access and add word document properties, such as creator, description, title, subject, keywords, lastModifiedBy, and revision.
Install DOCX via npm
//create a new document
const doc = new docx.Document();
// add properties to document
const doc = new docx.Document({
creator: "Dolan Miu",
description: "My extremely interesting document",
title: "My Document",
});
Manage Section of Word Docx File
Sections are subdivisions of a word document that contains its own page formatting. A section can be a collection of paragraphs that have a specific set of properties such as page size, page numbers, page orientation, headers, borders, and margins. These properties are used to describe the pages on which the text will appear. The DOCX library completely supports section creation and all the relevant properties related to a section.
Creates Simple Section in Word Document via .NET
const doc = new Document({
sections: [{
children: [
new Paragraph({
children: [new TextRun("Hello World")],
}),
],
}];
});
Manage Tables inside a Word File
Tables are one of the most common formatting elements that we are using in Microsoft Word. Tables help users to organize their content in different ways. The open source DOCX library gives software developers the capability to use tables inside their own JavaScript applications. The library supports adding a row to tables, repeating table rows, adding cells to the table, setting the width of a cell, adding text to a cell, adding the nested table, merging cells, adding columns, adding a border to a table, merge columns, and many more.
Add Table inside Word Documents via C#
const docx = require('docx@6.0.1');
const express = require("@runkit/runkit/express-endpoint/1.0.0");
const app = express(exports);
const { Document, Packer, Paragraph, Table, TableCell, TableRow } = docx;
app.get("/", async (req, res) => {
const table = new Table({
rows: [
new TableRow({
children: [
new TableCell({
children: [new Paragraph("Hello")],
}),
new TableCell({
children: [],
}),
],
}),
new TableRow({
children: [
new TableCell({
children: [],
}),
new TableCell({
children: [new Paragraph("World")],
}),
],
}),
],
});
const doc = new Document({
sections: [{
children: [table],
}],
});
const b64string = await Packer.toBase64String(doc);
res.setHeader('Content-Disposition', 'attachment; filename=My Document.docx');
res.send(Buffer.from(b64string, 'base64'));
});
Manage Headers/Footers in Word DOCX
The open source DOCX library has included complete support for adding and managing custom headers and footers inside Word DOCX files. The library allows developers to insert tables, pictures, text, and charts into the headers and footers section with ease. The library also allows us to add multiple headers and footers by creating more sections. You easily set new headers and footers as per Section.
Add Header/Footer in Word DOCX via NET API
const docx = require('docx@6.0.1');
const express = require("@runkit/runkit/express-endpoint/1.0.0");
const app = express(exports);
const { Document, Footer, Header, Packer, Paragraph } = docx;
app.get("/", async (req, res) => {
const doc = new Document({
sections: [{
headers: {
default: new Header({
children: [new Paragraph("Header text")],
}),
},
footers: {
default: new Footer({
children: [new Paragraph("Footer text")],
}),
},
children: [new Paragraph("Hello World")],
}],
});
const b64string = await Packer.toBase64String(doc);
res.setHeader('Content-Disposition', 'attachment; filename=My Document.docx');
res.send(Buffer.from(b64string, 'base64'));
});